Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial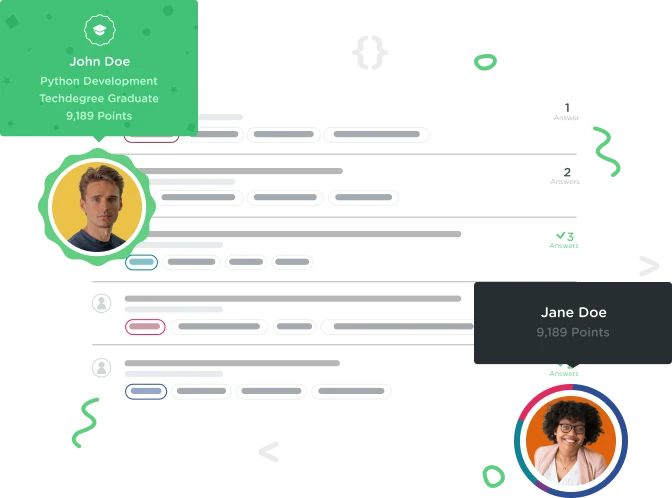

Shandralynn Mayberry
948 Pointsmadlibs print statement
In your solve code, you use 3 separate print statements. This is mine: print("I enjoy practice! I find it helps me to ", verb1, "better. Without practice, my ", noun1, "would probably not even work. My code is getting more ", adj1, "every single day!") Is this okay? Or does the statement need to be broken up into 3 separate statements? I understand why it would be broken up (possibly easier to debug?). I think I just got comma happy because my train of thought was "and this, then this, then this."
1 Answer
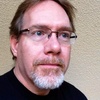
Chris Freeman
Treehouse Moderator 68,423 PointsYou style works as long as it's readable. Here are some alternatives along with wrapping long lines:
# Your style works as long as it's readable. Here are some alternatives along
# with wrapping long lines:
verb1 = "think"
noun1 = "brain"
adj1 = "precise"
# original code
print("I enjoy practice! I find it helps me to ", verb1, "better. Without practice, my ", noun1, "would probably not even work. My code is getting more ", adj1, "every single day!")
# I enjoy practice! I find it helps me to think better. Without practice, my brain would probably not even work. My code is getting more precise every single day!
# same, but wrapped
print("I enjoy practice! I find it helps me to ", verb1, "better. Without "
"practice, my ", noun1, "would probably not even work. My code is "
"getting more ", adj1, "every single day!")
# I enjoy practice! I find it helps me to think better. Without practice, my brain would probably not even work. My code is getting more precise every single day!
# older format style
print("I enjoy practice! I find it helps me to %s better. Without" % verb1,
"practice, my %s would probably not even work. My code is" % noun1,
"getting more %s every single day!" % adj1)
# I enjoy practice! I find it helps me to think better. Without practice, my brain would probably not even work. My code is getting more precise every single day!
# new format style
print("I enjoy practice! I find it helps me to {} better.".format(verb1),
"Without practice, my {} would probably not even work.".format(noun1),
"My code is getting more {} every single day!".format(adj1))
# I enjoy practice! I find it helps me to think better. Without practice, my brain would probably not even work. My code is getting more precise every single day!
# using newest f strings
print(f"I enjoy practice! I find it helps me to {verb1} better. Without "
f"practice, my {noun1} would probably not even work. My code is getting "
f"more {adj1} every single day!")
# I enjoy practice! I find it helps me to think better. Without practice, my {noun1} would probably not even work. My code is getting more {adj1} every single day!
Notice:
- Bare strings can be listed without commas, but that also means that no additional space will be added between them.
- using a
.format()
method or the%s
formating requires commas between the arguments. This adds a space between arguments - f strings can be concatenated without commas
Shandralynn Mayberry
948 PointsShandralynn Mayberry
948 PointsAlso, I used numerical tags at the end in case it eventually ended up being a longer form (verb1, noun1, adj1 could then be expanded upon with verb2, noun2, adj2, etc).