Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial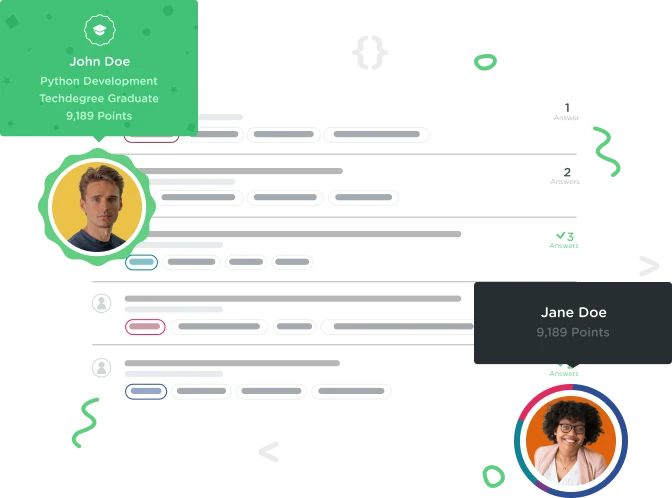

Felipe Lo Valvo
156 Pointsmain camera doesn't has rigid body
when i try to test my game unity says that my camera doesn't has "rigid body" and when i put it my camera does weird things. what should i do? heres the code: using System.Collections; using System.Collections.Generic; using UnityEngine;
public class PlayerMovement : MonoBehaviour { private Animator playerAnimator; private float moveHorizontal; private float moveVertical; private Vector3 movement; private float turningSpeed = 20f; private Rigidbody playerRigidbody;
// gather components to the player gameobject
void Start () {
playerAnimator = GetComponent<Animator> ();
playerRigidbody = GetComponent<Rigidbody> ();
}
// Update is called once per frame
void Update () {
// gather imput from the keyboard
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpdate () {
// if the players movement vector is not equal to zero...
if (movement != Vector3.zero) {
// ...then create a target rotation based on the movement vector...
Quaternion targetRotation = Quaternion.LookRotation(movement, Vector3.up);
//... and create another rotation that moves from the current rotation to the target rotation...
Quaternion newRotation = Quaternion.Lerp (playerRigidbody.rotation, targetRotation, turningSpeed * Time.deltaTime );
//... and change the players rotation to the new incremental rotation...
playerRigidbody.MoveRotation(newRotation);
//... then play the jump animation.
playerAnimator.SetFloat ("Speed", 3f);
} else {
// otherwise, dont play the jump animation
playerAnimator.SetFloat ("Speed", 0f);
}
}
}
1 Answer

Michael Zdarsky
4,533 PointsIt sounds like your camera has conflicting information. I would try to comment out the sections where you are assigning rotations and lerping functions and reset or set the cameras position and rotation to a constant in the code and see if you are still having problems. Then I would I would use that information to determine if the problem is in the code or the inspector. If the problem is in the code,I would make subtle changes and test it, until you figure out which section is conflicting.
Felipe Lo Valvo
156 PointsFelipe Lo Valvo
156 Pointshow do i reset or set the cameras position and rotation to a constant in the code?