Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial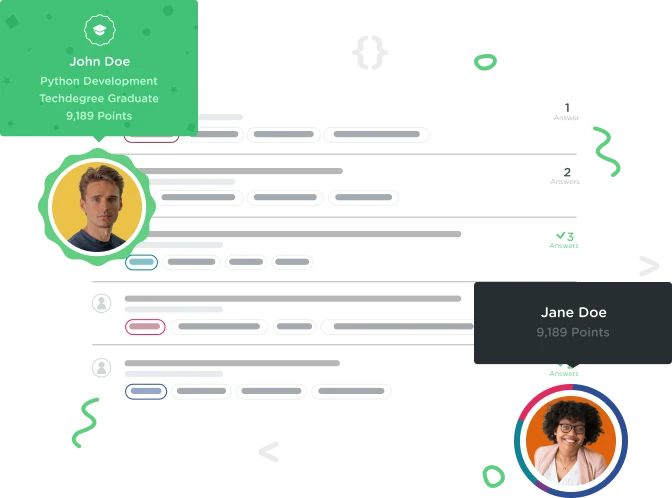
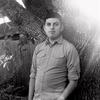
Oziel Perez
61,321 PointsMAJOR UPDATE: Must read if you are having problems with webpack
If people are still having problems with web pack and, additionally, with babel, be sure that your package.json file looks like this:
{
"name": "webpack-treehouse-example",
"version": "0.0.1",
"scripts": {
"build": "webpack --optimize-minimize",
"start": "webpack-dev-server"
},
"devDependencies": {
"babel-core": "^6.26.0",
"babel-loader": "^6.4.1",
"babel-preset-es2015": "^6.24.1",
"babel-preset-stage-2": "^6.24.1",
"css-loader": "^0.23.1",
"html-webpack-plugin": "^2.30.1",
"node-sass": "^3.8.0",
"sass-loader": "^4.0.0",
"style-loader": "^0.13.1",
"webpack": "^3.10.0",
"webpack-cli": "^2.0.13",
"webpack-dev-server": "3.0.0"
},
"dependencies": {
"jquery": "^3.1.0"
}
}
The first thing I want to point out is webpack itself. You must upgrade your version of webpack to version 3.10.0 (at the time of this comment). The reason for this is because if you upgrade to the latest version (4.x.x), the entire build will break. Html-webpack-plugin is not supported for the latest version at this time, so to prevent the "compilation.mainTemplate.applyPluginsWaterfall is not a function" error, you have to use version 3.10.0 of webpack. In this json file, this is the latest html-webpack-plugin version at this time. See this github issue thread for more insight: https://github.com/jantimon/html-webpack-plugin/issues/860
Next, install webpack-dev-server version 3.0.0 (yes this version). The latest version, 3.1.x, gives error that it cannot read property 'compile' of undefined. According to this github thread (https://github.com/webpack/webpack-dev-server/issues/1334), version 3.X.X is meant to run only with webpack version 4.X.X, so it is recommended to downgrade to dev server version 2.X.X but it seems version 3.0.0 works ok. Thread carefully.
Next, make sure that you install babel-core. Otherwise, you will get a dependency error. Apparently it was not included in this branch.
I took the liberty of upgrading babel-loader and the presets as well to the latest current versions (at the time of this comment).
Next, you have to install webpack-cli. Apparently, this is a separate package needed to run webpack in the command line. You will get error if it's not there.
Lastly, configure your webpack.config.js file like so:
const path = require('path');
var HtmlWebpackPlugin = require("html-webpack-plugin");
var webpackConfig = {
entry: "./src/index.js",
output: {
path: path.resolve(__dirname, "build"),
filename: "bundle.js"
},
module: {
rules: [
{
loader: "babel-loader",
test: /\.js$/,
exclude: path.resolve(__dirname, "node_modules")
}
]
},
plugins: [
new HtmlWebpackPlugin({
template: "src/index.ejs"
})
]
};
module.exports = webpackConfig;
1 Answer
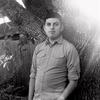
Oziel Perez
61,321 PointsVang Nguyen, that's strange behavior. I do see the directory under node modules listing webpack, and if your npm run build script is working then webpack is installed, it's just for some reason npm won't list it.
I mention to explicitly install babel-core just in case you cloned the repo from teamtreehouse. As far as I could tell, babel-core was not in that original package.json file and was throwing errors because it was not there, so as long as you npm install it or you write it in your package.json and install from there, you should be fine.
As for you build error, it appears that you may be missing a component of some sort. Have you checked that the js file for loadDropDown wasn't accidentally deleted?
Vang Nguyen
429 PointsVang Nguyen
429 PointsI updated my package.json. Deleted node_modules and package-loc.json and ran 'npm install' again. All packages seem to be installed except when I run npm list webpack it gives:
webpack-treehouse-example@0.0.1 /home/vang/code/treehouse/webpack-workshop └── (empty)
npm list babel-core gives:
webpack-treehouse-example@0.0.1 /home/vang/code/treehouse/webpack-workshop └─┬ babel-core@6.26.3 └─┬ babel-register@6.26.0
babel-core is in the package.json, why do you have say 'make sure that babel-core is installed.' Isn't it installed just part of npm install?
I'm on ubuntu. Why isn't webpack being listed as installed?
npm run build errors out:
Hash: 6477fffc92b6523ae42d Version: webpack 3.12.0 Time: 595ms Asset Size Chunks Chunk Names bundle.js 709 bytes 0 [emitted] main index.html 2.04 kB [emitted]
[0] ./src/index.js 284 bytes {0} [built]
ERROR in ./src/index.js Module not found: Error: Can't resolve './loadDropDown' in '/home/vang/code/treehouse/webpack-workshop/src' @ ./src/index.js 3:20-45 Child html-webpack-plugin for "index.html": 1 asset [0] ./node_modules/html-webpack-plugin/lib/loader.js!./src/index.ejs 2.4 kB {0} [built] [2] (webpack)/buildin/global.js 509 bytes {0} [built] [3] (webpack)/buildin/home/vang/.npm/_logs/2018-05-16T19_56_44_092Z-debug.log/module.js 517 bytes {0} [built] + 1 hidden module npm ERR! code ELIFECYCLE npm ERR! errno 2 npm ERR! webpack-treehouse-example@0.0.1 build:
webpack --optimize-minimize
npm ERR! Exit status 2 npm ERR! npm ERR! Failed at the webpack-treehouse-example@0.0.1 build script. npm ERR! This is probably not a problem with npm. There is likely additional logging output above.npm ERR! A complete log of this run can be found in: npm ERR! /home/vang/.npm/_logs/2018-05-16T19_56_44_092Z-debug.log