Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial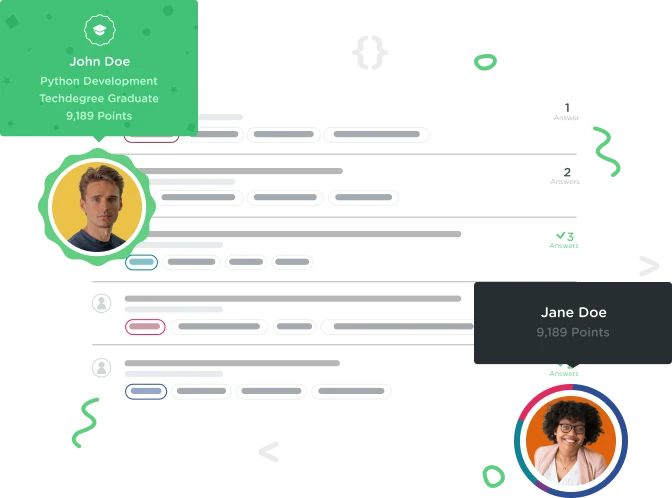
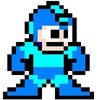
Robert Richey
Courses Plus Student 16,352 PointsMake a Constructor Challenge - My Dice Simulator
I accepted Andrew's challenge. Here is my Dice Simulator.
/**
* By default, new objects created by the Dice constructor will
* be 6-sided or the value of sides, if defined.
*
* It's assumed sides will be a number - i.e. I'm too lazy to validate the input
*/
function Dice(sides) {
this.sides = sides || 6;
this.roll = function () {
return Math.floor(Math.random() * this.sides) + 1;
};
}
var d6 = new Dice();
var d20 = new Dice(20);
4 Answers

Matt F.
9,518 PointsVery cool. Just tried it out and works great.
Right now I am learning about the prototype chain from materials produced by Kyle Simpson and one suggestion may be to add the role method to the .prototype of the Dice function. This way, if you for some reason had 10,000 dice, it would not require a separate role method in each object.
Again great work.
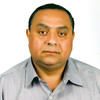
Mohsen Qaddoura
22,237 Points// can't add much to the beautiful answer preceding this one
function Dice(sides) {
this.sides = sides || 6;
this.roll = function() {
return Math.floor(Math.random() * this.sides) + 1;
};
}
dice6 = new Dice();
dice10 = new Dice(10);
console.log(dice10.roll()); /* Example for logging out
the return value of the roll method to the console. */
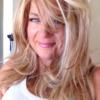
erika harvey
Courses Plus Student 14,540 Pointsi believe this is right ''' function Dice(sides) { this.sides = sides; this.roll = function{ Math.floor(Math.random() * this.sides) + 1;
}; } '''
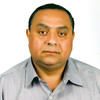
Mohsen Qaddoura
22,237 Points/* You missed: 1- "()" after "function". 2- and the "return" keyword in:
this.roll = function{ Math.floor(Math.random() * this.sides) + 1;
};
so it finally should look like this to run without errors and get some output of your code:
this.roll = function() {
return Math.floor(Math.random() * this.sides) + 1;
};
also modify, at the very beginning of your code, the " ``` " to " ```JavaScript " for your code to be shown formatted as code here on the forum. Best of luck you are doing well and keep at it :)
*/
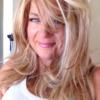
erika harvey
Courses Plus Student 14,540 Pointstyvm :)
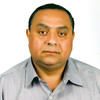
Mohsen Qaddoura
22,237 PointsYou are welcome.
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHi Matt,
Thanks for the feedback! You're right on about prototyping a roll method, which is useful for inheritance. At this point in the course, Andrew hasn't talked about prototyping yet, so I wanted to keep it as close to the covered material as possible.
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsIn the spirit of prototyping, here is an updated version. I'm so glad you mentioned this, because I had nearly forgotten that major benefit of prototyping - code isn't copied for each new object, they instead refer to the one copy from their parent.
Andrew Chalkley
Treehouse Guest TeacherAndrew Chalkley
Treehouse Guest TeacherI do talk about this shortly after :)