Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial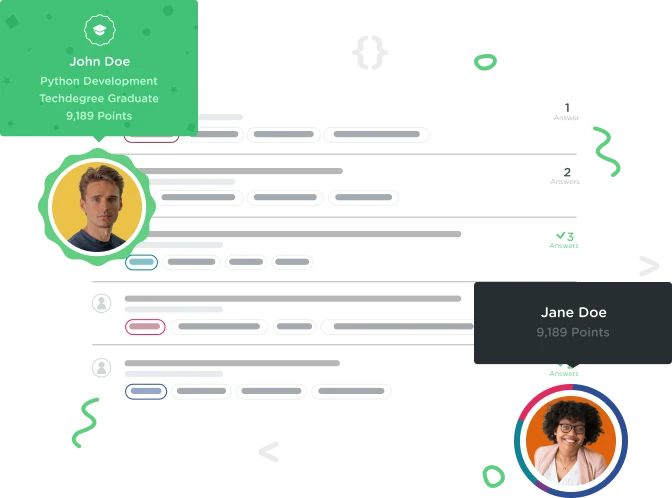
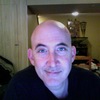
Charles Harpke
33,986 PointsMake a function name first_and_last_4 that accepts an iterable and returns the first 4 and last 4 items in the iterable.
Is there a way to contain the first and last 4 values after the utterable?
here is my code:
def first_4(iterable):
return iterable[0:4]
first_4([66, 333, 222, 1, 1234])
def first_4(iterable):
return iterable[0:4]
first_4([66, 333, 222, 1, 1234])
def odds(iterable):
return iterable[1::2]
def first_and_last(iterable):
return(iterable)[0:4]
15 Answers
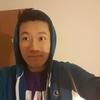
Anthony Liu
11,374 PointsI am not completely sure if the way I did it is the most efficient. In the "first_and_last" function
1.) I created a list for the first 4
2.) a list for the last 4.
3.)Combined the 2 lists
4.) returned the new list
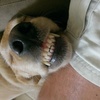
Herb Bresnan
10,658 Pointsdef first_4_last_4(item):
return item[:4] + item[-4:len(item):1]
item[:4] gives the first four numbers by index [0, 1, 2, 3]
concatenated with the
START is -4: STOP is len(item):STEP is 1
So start at the -4 index position, stop at the end of the list, and step by 1 until you reach the end of the list.

Anthony Attard
43,915 PointsCould be simplified to:
def first_4_last_4(item):
return item[:4] + item[-4::1]

Martin Krkoska
10,514 Pointsdef first_and_last_4(iterable):
return iterable[:4] + iterable[-4:]
this is working too and you dont need len(item) or step
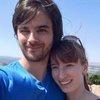
Matt Milburn
20,786 PointsHey guys, just wanted to offer up the correct answer here. You're welcome!
def first_and_last_4(iterable):
return iterable[:4] + iterable[-4:]
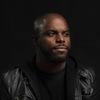
Anthony Jackson
19,505 PointsIf you want to do it in one line you can try
return first_4(iterable) + iterable[len(iterable)-4:]
this will reuse the code you already create in the first_4() function and combine it with your code for getting the last four
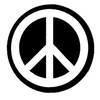
john larson
16,594 PointsHey Anthony, I was doing some review and I caught your answer here. I wouldn't have thought to do that. It's a nice little mind expander for me. I get so focused and boxed in sometimes I lose sight of the possibilities. Thanks
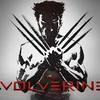
Wolverine .py
2,513 Pointsi know its been 4 months since you last post the question but i just got past through now. i hope it will help others

wafic fahme
3,309 Pointsod = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14]
return od[0:4] + od[-4:len(od)]

Kishore Kumar
5,451 Pointsdef first_and_last_4(arg2): list = arg2[:4] + arg2[len(arg2)-4:len(arg2)] return list

Jarrod Gillis
16,760 Pointsdef first_and_last_4(timberwolves): return timberwolves[:4] + timberwolves[-4:]

Akshay Kumar
1,098 Pointsdef first_and_last_4(iterable ): ite1 =iterable[0:4] ite2 = iterable[-4:] result = [] result.extend(ite1) result.extend(ite2) return result

patrick georges
1,827 Pointsdef first_4(something): return something[0:4]
def first_and_last_4(something): return something[:4] + something[-4:]
def odds(something): return something[1::2]
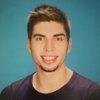
Murat Can Berber
3,238 Pointsdef first_and_last_4(text):
first = ''.join(text[:4])
second = ''.join(text[-4:])
return '{}{}'.format(first,second)
this should work also but it doesnt. Can anyone tell me why?
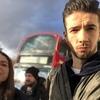
Jan Durcak
12,662 Pointsthis one ? def first_and_last_4(a): c= a[0:4] d = a[-1:-5:-1] a = c+d return a
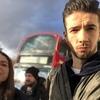
Jan Durcak
12,662 Points'''' def first_4_last_4(a): c= a[0:4] d = a[-4:] a = c+d return a ''''

victor chingtham
1,548 PointsThis is the correct one, already completed the first_4 in the task one
def first_4(iterable): return iterable[0:4]
def first_and_last_4(iterable): return first_4(iterable) + iterable[-4:]

Sean M
7,344 Pointshere's what I did:
#practice
messy_list = [1,2,3,4,5,6,7,8]
def first_4(a_list):
return a_list[:4]
def last_4(a_list):
return a_list[-4:]
def first_and_last_4(a_list):
return first_4(a_list) + last_4(a_list)
print(first_and_last_4(messy_list))
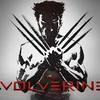
Wolverine .py
2,513 Pointsreturn iterable[0:4]+ iterable[45:50]
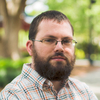
Kenneth Love
Treehouse Guest TeacherThis is not the right way to do it. This won't work if you don't know the length of the iterable. You need to come up with a solution that'll work on ANY iterable.
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherThat's pretty much the only way to do it. You can avoid creating some variables, though, by just combining the slices directly.