Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial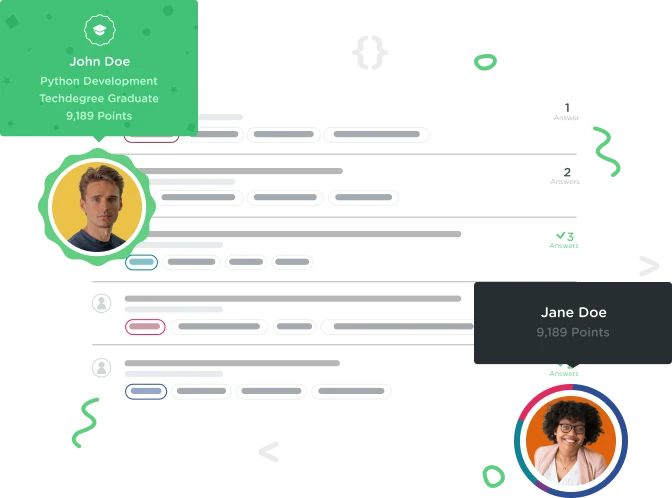

Kitty Wan
Courses Plus Student 4,048 PointsMake a function name first_and_last_4 that accepts an iterable and returns the first 4 and last 4 items in the iterable.
What's wrong with my code?
def first_4(x): return x[0:4]
def odds(x): return x[1::2]
def last_4(x): return x[-4:]
def first_and_last_4(x): return first_4.extend(last_4)
1 Answer
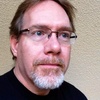
Chris Freeman
Treehouse Moderator 68,423 PointsThree errors with the function first_and_last_4()
.
- The functions
first_4()
andlast_4()
need to be passed arguments: -
extend()
only works on lists and not on strings, so if a string iterable were passed tofirst_and _last_4()
it would fail with anAttributeError: str has no attribute extend
- If passed an actually list,
extend()
operates on a list "in place", that is the output offirst_4
, which would be a list, is assigned to a temporary unnamed variable. Then extend alters this variable in place, but does not return a value. There is no value to be returned.
Ignoring the string input issue (not sure if you are asking about that aspect), see updated code below:
def first_4(x):
return x[0:4]
def odds(x):
return x[1::2]
def last_4(x):
return x[-4:]
def first_and_last_4(x):
result = first_4(x)
result.extend(last_4(x))
return result
or alternatively, just add the resultant parts:
def first_and_last_4(x):
return first_4(x) + last_4(x)
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsThis would be calling a method on a function/object, not a string:
Also, Kitty Wan, I think your
odds
function isn't returning the correct result, since lists in Python begin with a zero index.