Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial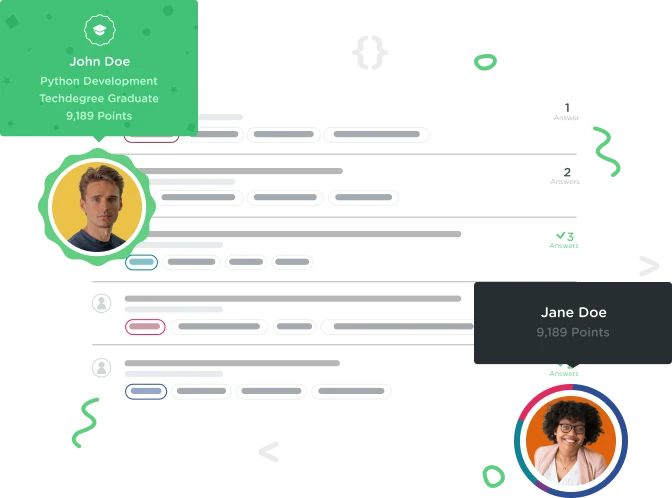

Rakshith Nayak
1,893 PointsMake a function name first_and_last_4 that accepts an iterable and returns the first 4 and last 4 items in the iterable.
Why does the code pass even though the word 'water' doesn't have enough characters in them. Water has only 5 characters in them. But here they want us to get the first 4 and the last 4 characters and water doesn't have that many characters but the code still passes. Any help would be much appreciated. thank you.
def first_4(formula):
return formula[:4]
def odds(springfeilds):
return springfeilds[1::2]
def first_and_last_4(water):
return water[:4] + water[-4:]
3 Answers

andren
28,558 Points"water" is not the word being sliced, you defined water as a parameter for the function (by placing it in the parenthesis), a parameter is essentially just a variable that gets set to a value when your function is called.
Take this code for example:
abc = "Hello World!"
print(abc[:-10])
Looking at that code is it surprising that you can use slice the abc variable with -10? No it probably isn't because it's obvious that it's just a variable, and the slicing is actually done on the string it contains which is longer than 10 characters.
The exact same is true of water, the only difference is that you can't see it being assigned a value because you are not the one calling your function, if you were you would also be specifying an argument to the function which would be the word that would be assigned to the water variable.
This is also true for your other functions, and all functions in fact. Parameters are simply variables, their entire purpose is to be assigned a value when the function is called, it's part of how you can send data to a function which is part of what makes them so dynamic and useful.
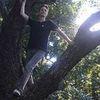
gyorgyandorka
13,811 PointsRegarding your second question: a word with three or less letters won't cause a problem, because, in Python, if the slice is longer than the actual list, then it will simply return the list - no "index out of bounds" error will be thrown. It's just the way that slicing was implemented in the language. If the slice is out of range in one or both directions, then the intersection will be returned; following this logic, if the slice is completely out of bounds (like list[5:7]
if len(list) < 5
), then it returns an empty list:
my_list = [1, 2, 3, 4]
my_slice1 = my_list[:5] # my_slice == [1, 2, 3, 4]
my_slice2 = my_list[2:7] # my_slice2 == [3, 4]
my_slice3 = my_list[5:7] # my_slice3 == []
Similarly, list.insert(<an index out of range>, <item>)
will do the same as list.append(<item>)
. (Again, no "out of bounds" error.) If the index is negative (and of course, >= len(list)
), then it will insert the item at the 0th index.
Other languages work differently, e.g. Ruby fills the places with nil
-s, Java throws an exception, etc.
http://stackoverflow.com/questions/25840177/list-insert-at-index-that-is-well-out-of-range-behaves-like-append

Rakshith Nayak
1,893 Pointsthank you for your response. It makes more sense now.
Rakshith Nayak
1,893 PointsRakshith Nayak
1,893 PointsOH i think I get it. In this case, it takes the first four items which is 'wate' and the last four items which is 'ater'. Am I right? But what if the word only has three characters in it. For example 'rum'. How would it take the first four items and the last four? thank you