Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial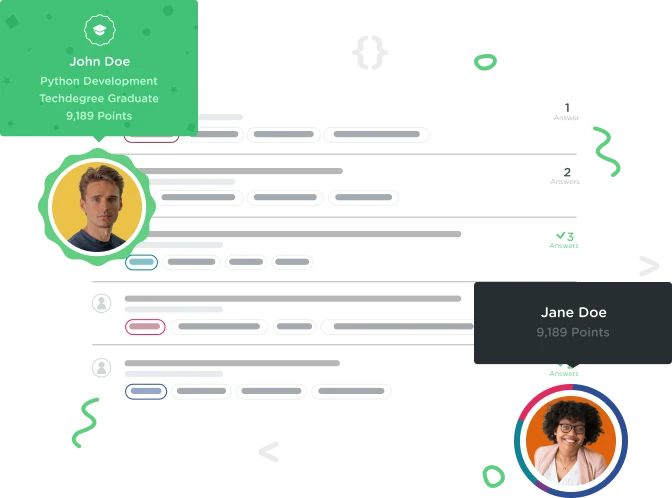

Joe Simonsen
2,684 PointsMake a function named add_list that adds all of the items in a passed-in list together and returns the total.
Completely stuck on this one. Any starting points would be nice.
3 Answers

Stone Preston
42,016 Pointsyou need to create a variable to store the total sum of the list and set it to 0 first. then loop over each item in the list parameter and add that item to the sum variable, then return the sum
remember that you can use a for in loop like so:
for something in some_list:
where something is the name you want to give to the item in the list you are looping over. it can be any valid variable name. after the word in you type the name of the list you want to iterate over (in this case the list we want to iterate over is some_list). then in the loop body you can reference the item in the list you are iterating over as whatever you decided to call it
so creating the variable to store the sum, looping over each item in the list and adding that item to the sum, and then returning the sum should look something like this:
def add_list(some_list):
sum = 0
for number in some_list:
sum = sum + number
return sum

Milos Rujevic
Full Stack JavaScript Techdegree Student 39,661 PointsI did it this way
def add_list(fun, *num): return sum(fun)
I didn't test this....
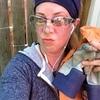
Ann Cascarano
17,191 PointsYou'll need to iterate over each number in the list and add it to the next. To do this, you could use a function - passing the list as the parameter. Then you could use a variable and give it a value of '0' so that you can add to it each number of the list. Once the iteration is complete, your variable will be the sum of the numbers in the list. A for loop would be a good fit for this.
Also - you might want to make use of the sum function - such as: x = [2, 3, 5, 567] total = sum(x)
Joshua Bivens
8,586 PointsJoshua Bivens
8,586 PointsWow. I don't think you could have figured that out from the video set.
Logan Wu
10,792 PointsLogan Wu
10,792 Pointsagree. it feels like your solution is not derived from the video, but yourself.