Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial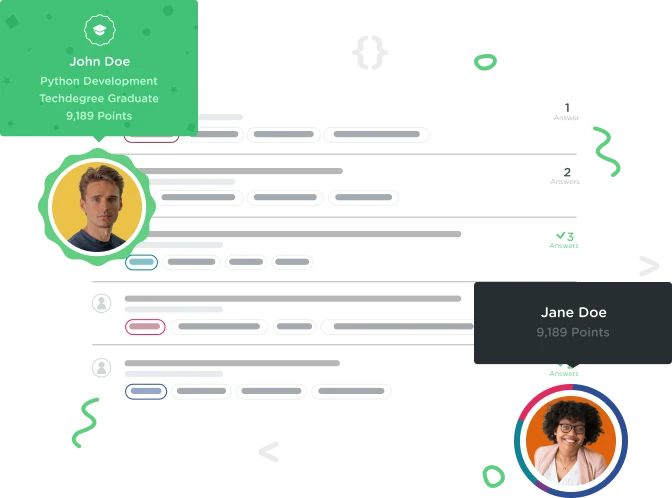
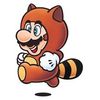
Corey Chattin
777 PointsMake a function named add_list that takes a list. I am stuck help!
Make a function named add_list that takes a list. The function should then add all of the items in the list together and return the total. Assume the list contains only numbers. You'll probably want to use a for loop. You will not need to use input().
I can't seem to get past this, what am I doing wrong? I'd love to continue the content but I am trapped!
9 Answers
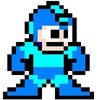
Robert Richey
Courses Plus Student 16,352 PointsHi Corey,
Let's break this down together and tackle each component separately.
- Make a function named add_list that takes a list
- The function should then add all of the items in the list together
- Return the total
def add_list(list_of_numbers):
# create a variable to holder the sum of values from the list - possibly call it counter
# loop over each number in list_of_numbers
# add current number in list to counter
# return counter
Let me know if this helps or not.
Cheers
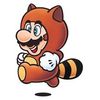
Corey Chattin
777 PointsThis started me thinking: I am so furstrated and feel like a complete idiot. I have this...
list_of_numbers = [1, 2, 3]
def add_list(list_of_numbers):
counter = []
for item in list_of_numbers:
counter.append(item)
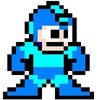
Robert Richey
Courses Plus Student 16,352 PointsEdited your post to display python with proper markdown. Your markdown was really close, just had an extra p in python (Ppython) and there needed to be a blank line above the markdown. Give me a few minutes and I'll take a closer look at your code.
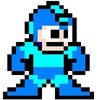
Robert Richey
Courses Plus Student 16,352 PointsThere is no need to define a list of numbers - when the challenge is run, it will supply it's own list to your function.
Your code is pretty close to being good.
- The counter variable will just be adding numbers together during each loop iteration, so this variable can be a number that starts at 0.
- The for loop declaration looks good.
- Inside the loop, for each item, add item to counter.
- Finally, at the end of the function, don't forget to return counter - which holds the sum of values from the number list.
def add_list(list_of_numbers):
counter = 0
for item in list_of_numbers:
# add to counter the value of item
return counter
So, just one line of code left to figure out. I'm confident you can do this.
Kind Regards
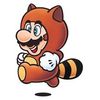
Corey Chattin
777 Pointsyeah I got it!
def add_list(list_of_numbers):
counter = 0
for item in list_of_numbers:
counter = counter + item
return counter
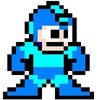
Robert Richey
Courses Plus Student 16,352 PointsAwesome! It's a great feeling to work through and solve a problem.
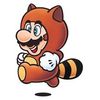
Corey Chattin
777 PointsSo I have this:
def add_list(list_of_numbers):
counter = 0
for item in list_of_numbers:
counter = item + counter
return counter
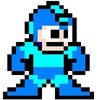
Robert Richey
Courses Plus Student 16,352 PointsYour code looks great! Only one small issue - the return statement is indented too far. The program thinks it's the last line of execution within your for loop - so the loop iterates once and then returns counter. Dedent return and you should pass the challenge (the level of indentation is a big deal in python).
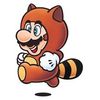
Corey Chattin
777 PointsTried this and every indent inbetween:
def add_list(list_of_numbers):
counter = 0
for item in list_of_numbers:
counter + item
return counter
The version of the code I posted above was returning an error of "Bummer! add_list didn't return the right answer. Got 0 when adding [1, 2, 3], expected 6."
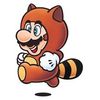
Corey Chattin
777 PointsThank you very much for your help today Robert! :)
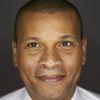
Neil Gordon
8,823 Pointsthis is what worked for me
list_of_numbers = [1,2,3]
def add_list(list_of_numbers):
for item in list_of_numbers:
counter = 3
counter = counter + item
return counter

Thomas Ton
9,836 PointsCheck this out
def add_list(number_list):
return sum(number_list)