Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial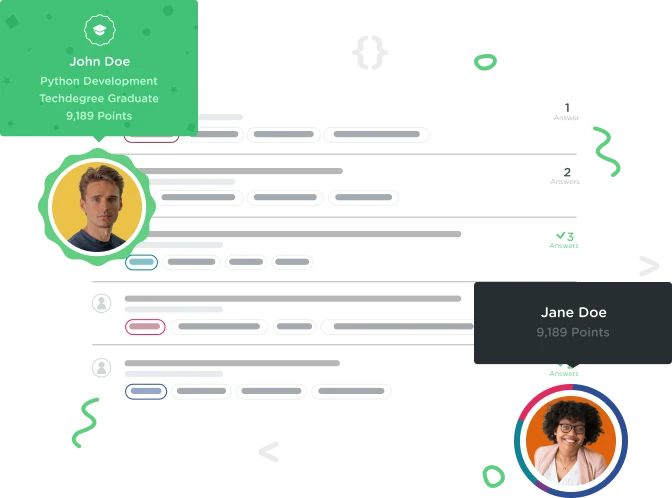

alec burnett
2,501 PointsMake a function named add_list that takes a list. The function should then add all of the items in the list together and
what did i do wrong
def add_list(lst) :
result = 0
for item in lst:
result += item
return result
8 Answers
Jack Middlebrook
19,746 PointsIt looks like for part 1 you need to change the line "return result" to be indented less. What you have currently returns the result after adding just the first item. With the changed indentation of the return statement your code should look like this and correctly return the result:
def add_list(lst) :
result = 0
for item in lst:
result += item
return result
For the second part you need to write a function that will use add_list from above. The way that I did it was using add_list() and just concatenating strings like below:
def summarize(lst):
return "The sum of " + str(lst) + " is " + str(add_list(lst)) + "."
The str() function will return a string representation of the object that you pass it. In the example above, str(lst) will return the list as a string and str(add_list(lst)) will return the sum total of the list as a string.
Hope that helps.

Orestis Pouliasis
5,561 Pointsdef add_list(items_list):
total = 0
for item in items_list:
total = total + item
return total
Try this and let me know if it worked for you. I will be here to answer any question.

alec burnett
2,501 Pointsit worked can you help- me with part 2 please

Orestis Pouliasis
5,561 PointsPlease post as a comment the question.

alec burnett
2,501 PointsNow, make a function named summarize that also takes a list. It should return the string "The sum of X is Y.", replacing "X" with the string version of the list and "Y" with the sum total of the list.

Orestis Pouliasis
5,561 Pointsdef add_list(items_list):
total = 0
for item in items_list:
total = total + item
return total
def summarize(items_list):
list = str(items_list)
total = 0
for item in items_list:
total = total + item
return "The sum of {} is {}.".format(list,total)
Let me know if it worked or if you have any questions.

alec burnett
2,501 Pointsthat full bottom part???????????????

alec burnett
2,501 Pointsare you mad bruh

alec burnett
2,501 Pointsits ok if your mad im only 15

Orestis Pouliasis
5,561 PointsWhat do you mean? Do you want something to be explained further?

alec burnett
2,501 Pointsit didn't work it says its wrong

Orestis Pouliasis
5,561 PointsTry deleting those:
add_list([1, 2, 3]) should return 6 summarize([1, 2, 3]) should return "The sum of [1, 2, 3] is 6." Note: both functions will only take one argument each.
It may solve your problem