Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial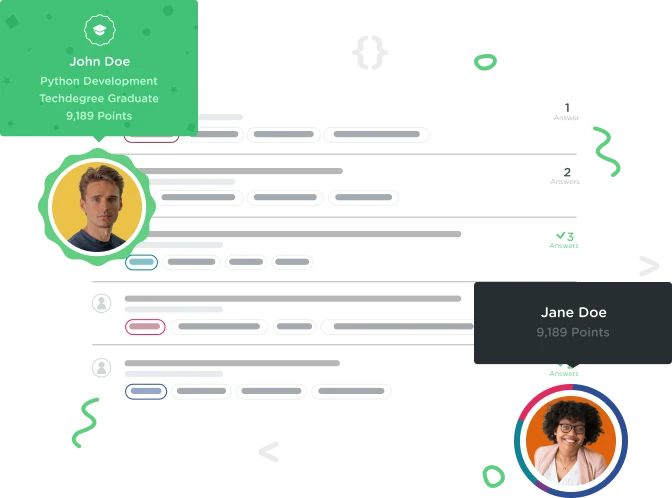

Frank Sherlotti
Courses Plus Student 1,952 PointsMake a function named first_4 that accepts an iterable as an argument and returns the first 4 items in the iterable.
I don't understand what I did wrong. I've done this in workspaces and it returns the first 4 items.
first_4 = [1, 2, 3, 4, 5, 6, 7, 8, 9]
first_4[0:4]
4 Answers

Dan Johnson
40,532 PointsYou need to define a function that will take any iterable and return the first four elements of whatever was passed in:
def first_4(iterable):
# Return the first four elements of iterable
You won't need to define a global list, one will be passed in automatically.

MUZ141110 Bryan Bera
3,943 Pointsdef first_4(iterable): return list(iterable)[0:4]

Frank Sherlotti
Courses Plus Student 1,952 PointsDan Johnson i need your help again. Or anyone else that can help with this would be great. Make a function name first_and_last_4 that accepts an iterable and returns the first 4 and last 4 items in the iterable.
def first_4(num):
return num[0:4]
def odds(oklahoma):
return oklahoma[1::2]
def first_and_last_4(numbers):
return numbers[0:4]
I understand that the numbers[0:4] will pull the first 4 numbers that i want but I don't understand how to pull the last 4 numbers. Now I'm sure I can use -1 to pull the last number and -2 to pull to pull the second to last number and so on. But I don't understand where to put it in the function.

Dan Johnson
40,532 PointsAn easier way to think about how to get the last four numbers is to think about what negative index you should start from, i.e. how to get the fourth element starting from the end.
As for how to structure the function, we can define two variables local to it:
def first_and_last_4(numbers):
# left and right are local to the function
left = numbers[0:4]
right = # Try your solution for the last 4 elements
# Adding two lists will create a merged list.
return left + right
You can do this on one line, but if you were curious about how the lifetime of variables works in a function, when first_and_last_4 is called left and right will be assigned the list slices defined above, and once the function returns they will no longer exist. This will be repeated each time the function is called.

Frank Sherlotti
Courses Plus Student 1,952 PointsOk i got it! But you mentioned that I could do this all on one line. I'm curious to how that works because it would seem more efficient than writing all of that out. I really do appreciate you going through this much detail with me it means a lot.

Dan Johnson
40,532 PointsIt's no problem, it's what we're here for.
For reducing the code, since left and right were just placeholders for the slices with no further modifications needed, you can just append them right from the results of the slices instead:
return numbers[:4] + numbers[-4:]

Frank Sherlotti
Courses Plus Student 1,952 PointsAlright thanks! I do have one last question though if you don't mind answering it for me. I have an extra credit thing that I've been working on and the section asks: Change the formatting of the list display. I don't quite understand what the question is asking me to do, if you could give me a brief explanation of what it means so I can try and work it out that'd be great.

Dan Johnson
40,532 PointsSounds like it just wants you to mess with the options of format to make a nicer display. Here's a list of some examples from the official docs.
This will let you mess with fill, text alignment, etc.

Shelley Brown
7,613 PointsThis will work also. I had a difficult time with this challenge.
def first_4(number): return list (number[0:4])
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsStill don't understand this and it's starting to get demoralizing.
Dan Johnson
40,532 PointsDan Johnson
40,532 PointsWhen you want to return a value from a function, you use the
return
keyword.This will exit the function, sending back the result of
iterable[0:4]
.Note that you don't have to use the name iterable, you can rename it to whatever you want.
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsThe help is greatly appreciated! I'm really bad at reading the question and i'll try and work on this more.
Michel van Essen
8,077 PointsMichel van Essen
8,077 Pointsreturn iterable can be written even shorter by typing return iterable[:4]