Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial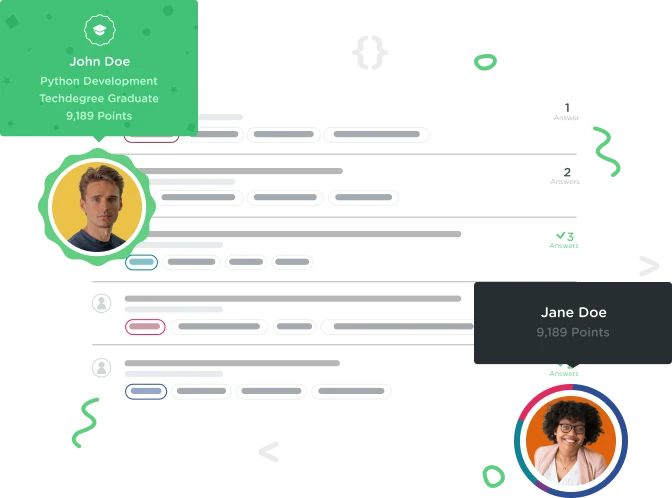

Pete P
7,613 PointsMake my 'correct' code better. Is it possible to view instructor's solution?
I'm successfully completing the challenge tasks. However, I'm sure that there sometimes may exist a more efficient, pythonic or just plain better way of doing it. Is it possible to view the instructors answers to the challenges?
As an example, here is my 'Teacher Stats' code. I have a few ideas on how I could shorten the code but I'm failing with implementation...
Thanks for the help!
# The dictionary will be something like:
# {'Jason Seifer': ['Ruby Foundations', 'Ruby on Rails Forms', 'Technology Foundations'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Often, it's a good idea to hold onto a max_count variable.
# Update it when you find a teacher with more classes than
# the current count. Better hold onto the teacher name somewhere
# too!
#
# Your code goes below here.
def most_classes(my_dict):
max_count = 0
teacher = ''
for key in my_dict:
if len(my_dict[key]) > max_count:
max_count = len(my_dict[key])
teacher = key
return teacher
def num_teachers(my_dict):
return len(my_dict)
def stats(my_dict):
my_list = []
for key in my_dict:
temp_list = [key, len(my_dict[key])]
my_list.append(temp_list)
return my_list
1 Answer
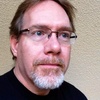
Chris Freeman
Treehouse Moderator 68,423 PointsYour stats
function is good! The only improvement I see would be to take advantage of the dict.items()
method.
By default, when iterating over a dictionary the assumed method is dict.keys()
, there is also dict.values()
(just the values instead of the keys), and dict.items()
which is both the key and the value as a tuple. By using .items()
you can save having to look up the teacher's courses again.
temp_list
isn't strictly needed, so it can be folded into the append
statement.
def stats(my_dict):
my_list = []
for key, value in my_dict.items():
my_list.append([key, len(value)])
return my_list
If you curious about other questions and solutions on this challenge, you can follow the Teacher Stats link at the top of the page.