Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial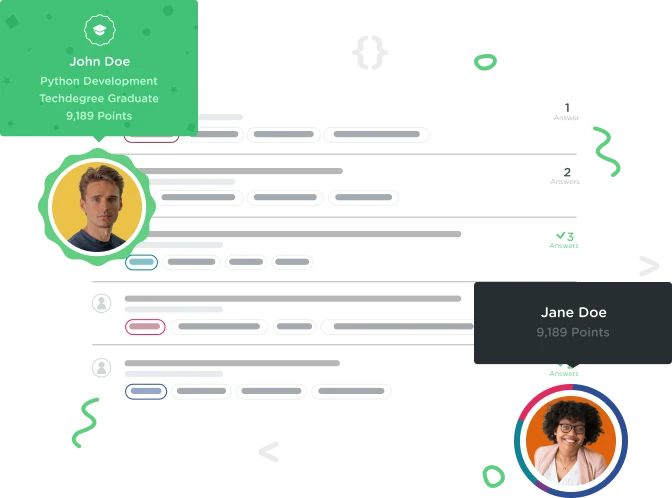
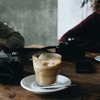
Mitchell Warmerdam
4,225 PointsMake sure the results array contains the first 10 multiples of 6
I'm stuck at the point where I'm asked to create append the results of a value by creating a loop. The array has to contain the first 10 multiples of 6. This is my query and I don't understand why it's not working. Your help would be much appreciated ;)
Thanks,
var results: [Int] = [1,2,3,4,5,6,7,8,9,10]
for multiplier in 1...10 { results.append(multiplier * 6) }
5 Answers

Michael Reining
10,101 PointsHi Mitchell,
I would break it down.
Step 1: Create a loop that iterates 10 times and name the constant multiplier
for multiplier in 1...10 {
}
Step 2: Multiply the value by 6 and add it to the results array.
var results: [Int] = []
for multiplier in 1...10 {
let result = multiplier * 6
results.append(result)
}
The initial array should definitely be left empty. You specify how often to run a loop by using 1...X where X is the number of times you want to run the loop.
I hope that helps,
Mike
PS: Thanks to the awesome resources on Team Treehouse, I just launched my first app. :-)
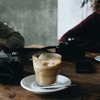
Mitchell Warmerdam
4,225 PointsHi Michael,
Thanks for helping out. I understand what you mean and I tried your solution but it still tells me "Your array needs to contain the first 10 multiples. Make sure your range goes from 1 to 10". Stefan, your explanation also makes sense now, I see what I am doing wrong but I'm still not able to find the solution. Hope you guys can still help me out here.
Thanks! Mitchell
var results: [Int] = [1,2,3,4,5,6,7,8,9,10]
for multiplier in 1...10 {
let result = multiplier * 6
results.append(result)
}
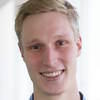
Stefan Cimander
37,115 PointsThe only problem in your code is, that still your results array does contain too many values at the end. You don't need to write
var results: [Int] = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Instead, you should start with an empty array, that is only filled within the for-loop.
var results: [Int] = []
The rest of your code works perfectly fine.
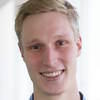
Stefan Cimander
37,115 PointsImplementing your approach, the final results array looks like this after executing the loop: results = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 6, 12, 18, 24, 30, 36, 42, 48, 54, 60]
Instead, make sure to start with an empty results array.
I'm not sure, why you are instantiating the results array with the values from 1 to 10. I guess you kind of think that you need it for the loop condition, but therefore, the range operator 1...10 is used. So, there is really no need to start with a non-empty results array.
Hope this helps.
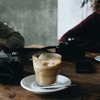
Mitchell Warmerdam
4,225 PointsYou are right! I finally completed the challenge with your help. Thanks a lot, I appreciate it
Cheers, Mitch
Michael Reining
10,101 PointsMichael Reining
10,101 PointsHave you tried to complete the challenge by leaving the initial array empty? That should definitely do the trick.