Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial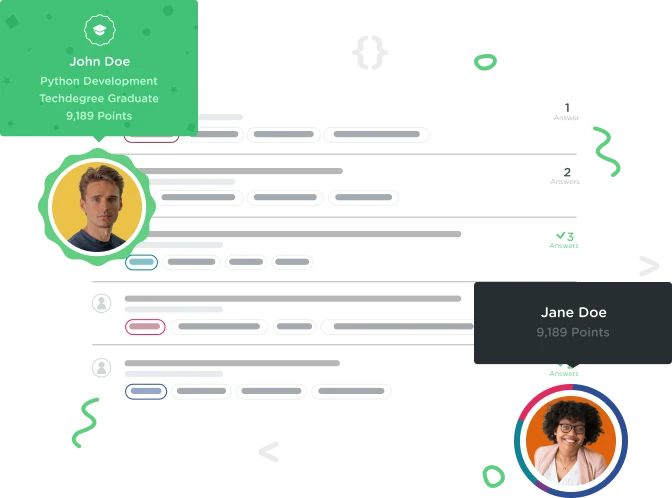
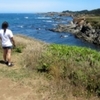
Nancy Melucci
Courses Plus Student 35,157 PointsMaking a function to return an object from an ArrayList
Hello,
The task is to: "Person getPerson(); - Returns the next Person in the collection. Each time this function is called it will return the next Person in the list or null if the AddressBook is empty. Once you get to the end of the list you should start from element 0 and return the first person."
I have created a working class that makes person objects. I have tried to make a constructor that accepts an arraylist of the Person objects. I had a lot of trouble with the static/non static error and having solved that, I can't get the getPerson(); function return anything but null.
I am including my code. I hope someone can pont me in the right direction. I hope I am being clear...
import java.util.Scanner;
import java.util.ArrayList;
import java.util.Arrays;
//program class
public class Person {
private String firstName;
private String lastName;
private String address;
static ArrayList <Person> list;
//
//default
public Person()
{
}
public Person(ArrayList<Person> list) {
// TODO Auto-generated constructor stub
new ArrayList<Person>();
}
//sets to string
public Person(String firstName, String LastName)
{
this.firstName = " ";
this.lastName = " " ;
}
//official constructor
public Person(String firstName, String lastName, String address) {
// System.out.println("Constructing a Person");
this.firstName = firstName;
this.lastName = lastName;
this.address = address;
}
//setters and getters
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public void setAddress(String address) {
this.address = address;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
public String getAddress() {
return address;
}
//to String
@Override
public String toString() {
return "Person is named " + this.firstName + " " + this.lastName + " and works at " + this.address;
}
public static Person getPerson() {
list = new ArrayList<Person>();
int i = 0;
if (list.size() > 0) {
Person person = list.get(i);
i++;
return person;
} else {
return null;
}
}
//main
public static void main(String[] args) {
String firstName = "";
String lastName = "";
String address = "";
//declare and fill array
Person person[] = new Person[3];
for (int i = 0; i < 3; i++) {
person[i] = new Person(firstName, lastName, address); // convert each reference variable into object
}
//function calls
getPeople(person);
printPeople(person);
//declare and fill arraylist
ArrayList<Person> people = new ArrayList<Person>(Arrays.asList(person));
//System.out.println("Print Arraylist using for loop");
for(int i=0; i < people.size(); i++){
System.out.println( people.get(i) );
}
System.out.println(getPerson());
}//end main
//utility functions
public static Person[] getPeople(Person person1[]) {
Scanner input = new Scanner(System.in);
for (int i = 0; i < 3; i++) {
System.out.println("Enter the first name: ");
String firstName = input.nextLine();
person1[i].setFirstName(firstName);
System.out.println("Enter the last name: ");
String lastName = input.nextLine();
person1[i].setLastName(lastName);
System.out.println("Enter the address: ");
String address = input.nextLine();
person1[i].setAddress(address);
}
input.close();
return person1;
}//end getPeople
public static void printPeople(Person person[]) {
int arrayLength = person.length;
for (int i = 0; i < arrayLength; i++) {
System.out.println(person[i].toString());
}
}//end printPeople
}//end program
3 Answers

Katsiaryna Krauchanka
Full Stack JavaScript Techdegree Graduate 22,154 PointsIt looks like you mixed up java data structures: Array and ArrayList.
In the class declaration you say "static ArrayList <Person> list" but your function "public static Person[] getPeople(Person person1[])" is dealing with the array. Remember that Arrays in Java have fixed length and you cannot append new values to them.
ArrayList of people (objects of Person class) as well as all methods which deal with it must be static, because they don't belong to a particular person (compare with attributes firstName or lastName) and must exist independently of any instance of a Person object.
You can create an ArrayList of people following this approach:
public static ArrayList <Person> createPeople() {
ArrayList <Person> people = new ArrayList<>();
Scanner input = new Scanner(System.in);
for (int i = 0; i < 3; i++) {
System.out.println("Enter the first name: ");
String firstName = input.nextLine();
System.out.println("Enter the last name: ");
String lastName = input.nextLine();
System.out.println("Enter the address: ");
String address = input.nextLine();
people.add(new Person(firstName, lastName,address));
}
input.close();
return people;
}
Also, I'd not initialize a list of people in the constructor, I'd do that in a setter method. However, there might be even better way of doing that.
public static void setPeople(){
list = createPeople();
}
After that, creating getPerson() function is quite easy. The only trick is that you need a global variable 'counter' which will keep track of where you stopped last time
private static int counter = 0;
public static Person getPerson1(){
if (list.size()==0){
return null;
}
if (list.size() ==counter){
counter = 0;
}
counter ++;
return list.get(counter -1);
}
To start the program, call in the main method:
Person.setPeople();
Person.getPerson1();
You can call getPerson1() several times to make sure that it returns the next person every time
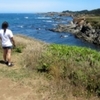
Nancy Melucci
Courses Plus Student 35,157 PointsPS. List is the AddressBook. When I figure this out, I'll refactor.