Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial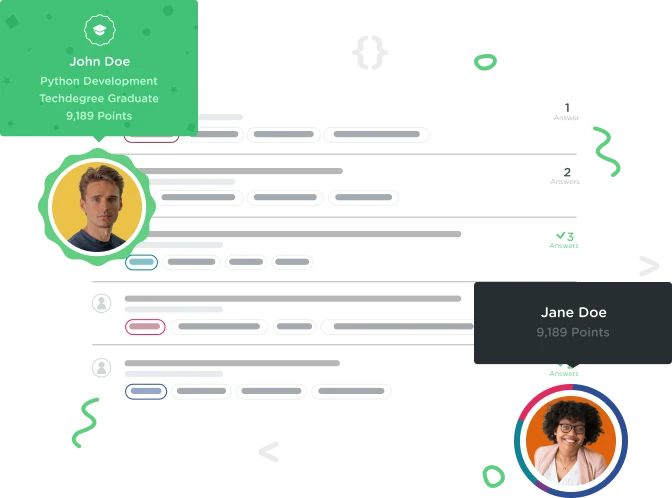

Terence Evans
1,870 PointsMaking a Toast
In the first Challenge I entered this:
Toast allDoneToast; Toast.makeText(this, "All Done!", Toast.LENGTH_LONG).show();
The first line was provided.
When I compiled it I got:
JavaTester.java:76: error: variable allDoneToast might not have been initialized else if (allDoneToast.mConstructorCalled) { ^ 1 error
What am I missing?
6 Answers
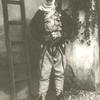
amathiaitishar
13,229 PointsHi Terence,
You do not need to initialize with a [new] keyword, but you type equal sign [=] and the rest of the code.
the exact code that worked for me and should work for you as well is as follows:
[Toast allDoneToast = Toast.makeText(this, "All Done!", Toast.LENGTH_LONG);]
Best, Amathia

Terence Evans
1,870 PointsThanks for the help Prateek. I tried both and a combination of them (1st line from #2 added to solution #1) and got the message: "Make sure to use the 'this' keyword as the first parameter of the 'makeText()' method."
This doesn't seem to make sense as I have used 'this' right after the " ( " . Any other ideas?

Terence Evans
1,870 PointsThanks Amathia.
My compiler error is gone, but when I hit the "Check Work" button in the right side, I'm getting a message that says "Bummer! Make sure to use the 'this' keyword as the first parameter of the 'makeText()' method. How is the word "this" NOT the first parameter?
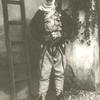
amathiaitishar
13,229 PointsHi Terence,
If would be good if you could post your code and we can see where is the issue, cuz it is hard to guess only with textual information.
The first challenge tasks, requires from your to use Toast class (.) makeText(this, "All Done!", Toast.LENGTH_LONG)... the exact code would be: Toast allDoneToast = Toast.makeText(this, "All Done!", Toast.LENGTH_LONG);
Hope that helped :)

Terence Evans
1,870 PointsSorry for being a bother. Maybe I should take up knitting or something.
What is in the ToastTest.java box is: 1 Toast allDoneToast = new Toast(); 2 Toast.makeText(this, "All Done", Toast.LENGTH_LONG).show();
and the message I get back when I submit it is:
Bummer! Make sure to use the 'this' keyword as the first parameter of the 'makeText()' method.
By the way, this is Challenge Task 1 of 3
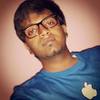
Prateek Prasad
7,292 PointsOk so you've gone wrong here's how it's done...
There are 2 ways of Implementing a Toast.
1) Via method chaining-
Toast.makeText(this,"All Done !",Toast.LENGTH_SHORT).show();
2) Creating an object of the Toast class -
Toast allDoneToast = new Toast();
allDoneToast.makeText(this,"All Done !",Toast.LENGTH_SHORT);
allDoneToast.show();

Terence Evans
1,870 PointsThank you, thank you, thank you. You know, pounding your head against the wall feels better when you stop.
amathiaitishar
13,229 Pointsamathiaitishar
13,229 PointsIt says that your variable allDoneToast was not initialized, so in your case you can do like this:
//--> a string variable to hold your message "All Done":
[String allDoneToast = "All Done";]
//--> a Toast variable, data type Toast --> initializing this variable using a static method of the toast class.
[Toast welcomeToast = Toast.makeText(this, allDoneToast, Toast.LENGTH_LONG);]
//--> method to show this in our app : [welcomeToast.show();]
This was a more structured way of coding (better to understand), whereas you can write this code in one single line:
[Toast.makeText(this, "All Done", Toast.LENGTH_LONG).show();]
Hope that helps :) Amathia
p.s. within [] is the code you can use, the rest is just an explanation. In the code challenge you do not need the show method()!