Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial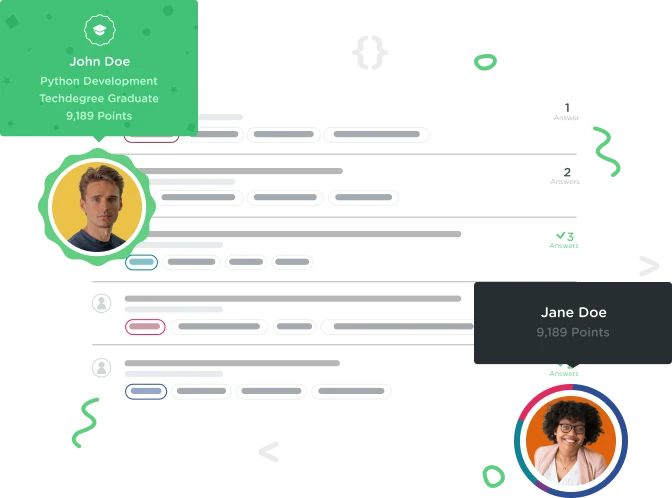

Tim rkan
36 Pointsmaking healer system
how can i add a healer system so you can choose to doge or heal your self?
8 Answers
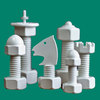
Steven Parker
231,572 PointsThe Rest option allows you to heal.
Assuming you are using (and have not modified) the code from the final video, then if it's your turn, your choices are "[A]ttack, [R]est, [Q]uit". If you choose "R" (rest), and the current hit points are less than the base points, your character will heal by one point.
If a monster is attacking, you should have the option "Dodge? Y/N". If you choose "Y" your character will attempt to dodge. A random value will be chosen by the program to determine if you are successful, with a probability of 1 out of 3.

Tim rkan
36 Pointsokej, I want Rest inside a class named healer.py how can i change that?
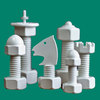
Steven Parker
231,572 PointsDid you add import healer
to game.py?

Tim rkan
36 Pointsgame.py : AttributeError: 'Character' object has no attribute 'rest'
need to change to game.py understand its inside heal.py

Tim rkan
36 Pointsgame.py
import sys
from character import Character
from heal import Heal
from monster import Dragon
from monster import Goblin
from monster import Troll
class Game:
def setup(self):
self.player = Character()
self.monsters = [
Goblin(),
Troll(),
Dragon()
]
self.monster = self.get_next_monster()
def get_next_monster(self):
try:
return self.monsters.pop(0)
except IndexError:
return None
def monster_turn(self):
if self.monster.attack():
print("{} is attacking!".format(self.monster))
if input("Dodge? Y/N ").lower() == 'y':
if self.player.dodge():
print("You dodged the attack!")
else:
print("You got hit anyway!")
self.player.hit_points -= 1
else:
print("{} hit you for 1 point!".format(self.monster))
self.player.hit_points -= 1
else:
print("{} isn't attacking this turn.".format(self.monster))
def player_turn(self):
player_choice = input("[A]ttack, [R]est, [Q]uit? ").lower()
if player_choice == 'a':
print("You're attacking {}!".format(self.monster))
if self.player.attack():
if self.monster.dodge():
print("{} dodged your attack!".format(self.monster))
else:
if self.player.leveled_up():
self.monster.hit_points -= 2
else:
self.monster.hit_points -= 1
print("You hit {} with your {}!".format(
self.monster, self.player.weapon))
else:
print("You missed!")
elif player_choice == 'r':
self.players.rest()
elif player_choice == 'q':
sys.exit()
else:
self.player_turn()
def cleanup(self):
if self.monster.hit_points <= 0:
self.player.experience += self.monster.experience
print("You killed {}!".format(self.monster))
self.monster = self.get_next_monster()
def __init__(self):
self.setup()
while self.player.hit_points and (self.monster or self.monsters):
print('\n'+'='*20)
print(self.player)
self.monster_turn()
print('-'*20)
self.player_turn()
self.cleanup()
print('\n'+'='*20)
if self.player.hit_points:
print("You win!")
elif self.monsters or self.monster:
print("You lose!")
sys.exit()
Game()
heal.py
import os
class Heal:
def rest(self):
if self.hit_points < self.base_hit_points:
self.hit_points += 1
character.py
import random
from combat import Combat
from heal import Heal
class Character(Combat):
attack_limit = 10
experience = 0
base_hit_points = 10
def attack(self):
roll = random.randint(1, self.attack_limit)
if self.weapon == 'sword':
roll += 1
elif self.weapon == 'axe':
roll += 2
return roll > 4
def get_weapon(self):
weapon_choice = input("Weapon ([S]word, [A]xe, [B]ow): ").lower()
if weapon_choice in 'sab':
if weapon_choice == 's':
return 'sword'
elif weapon_choice == 'a':
return 'axe'
else:
return 'bow'
else:
return self.get_weapon()
def __init__(self, **kwargs):
self.name = input("Name: ")
self.weapon = self.get_weapon()
self.hit_points = self.base_hit_points
for key, value in kwargs.items():
setattr(self, key, value)
def __str__(self):
return '{}, HP: {}, XP: {}'.format(self.name, self.hit_points, self.experience)
def leveled_up(self):
return self.experience >= 5
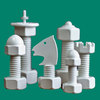
Steven Parker
231,572 PointsBe sure to format your code using the instructions in the Markdown Cheatsheet below the "Add an Answer".

Tim rkan
36 Pointsupdated Steven. still have problem in file game.py: AttributeError: 'Character' object has no attribute 'rest' need to make game.py understand the object is inside heal.py
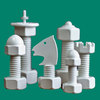
Steven Parker
231,572 PointsYou may need much more extensive changes to make this work.
I didn't see any problem recognizing the new heal.py file. But there are several other more serious issues.
For one thing, in game.py, when the player choses the "R
" option, there is this code:
self.players.rest()
But the Game object, which "self" refers to here, has no "players" property. Now it does have a "player" property (no "s"), but the player contains a Character object, and that object no longer has a "rest" method (It did in the original code).
Even if you were to invoke Heal.rest(), you would still have other issues. The code in that method refers to two properties, "hit_points" and "base_hit_points", but neither of those properties exist in the Heal class. And if you added them to the class, the method will still not improve the health of the Character object. If you still want to improve the player health, it's not clear to me why removing the method from the Character object would be desirable, but it will certainly require a lot more code.
This might be a good time to re-think your objectives for making the changes. Perhaps what you want to accomplish can be done in a simpler way.

Tim rkan
36 Pointscan you tell me how i can make it easier?
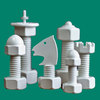
Steven Parker
231,572 PointsI still have no clue what you are trying to accomplish.

Tim rkan
36 Pointsi want rest (R) to be a own class. rest will be inside heal.py insted of character.
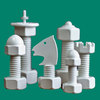
Steven Parker
231,572 PointsYou misunderstood me .. I meant what did you want to make different about the way the game behaves?
Making changes that do not affect program behavior is called refactoring, and has no value unless it makes the code smaller, faster, or more maintainable. What you are suggesting would do the opposite.

Tim rkan
36 PointsI only want to put rest inside a own class. its about learning :)
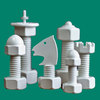
Steven Parker
231,572 PointsYou'll get much better value from your learning if you concentrate on "best practices". What you are talking about doing would actually violate many best practices. It's like a student of auto mechanics asking another student to "help me replace the gas tank with a tin washtub mounted on the roof."
I might help you with some custom projects if I thought they would be a positive reinforcement to your course learning, but I'm going to let you continue this particular task on your own.
But good job on learning how to format your code with Markdown. And when lots of code is involved, an even better way to share it is to make a snapshot of your workspace and post the link to it here.
Best of luck, and happy coding.
Chris Howell
Python Web Development Techdegree Graduate 49,702 PointsChris Howell
Python Web Development Techdegree Graduate 49,702 PointsCould you show us the code you have thus far? Or Share your Workspace if you wrote your code in it?
Its hard to say how to approach something, the less I know what code you have in-place the more assumptions I have to make. :)