Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial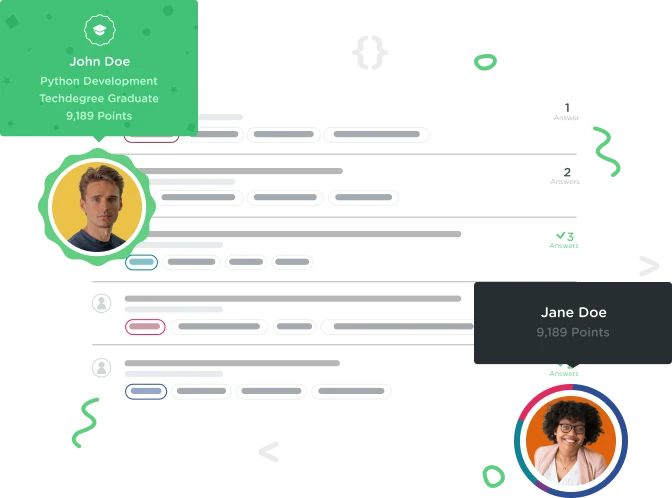
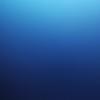
Michael Meadows
2,402 PointsMaking sure I am accepting a single parameter in my function with correct argument labels.
For some reason the compiler keeps saying that I have incorrect argument labels, but I cannot seem to figure out what the problem is. I'm using the local label in the function. Any idea on what the problem seems to be? Maybe it has to do with tuple instead. Thanks!
// Enter your code below
func coordinates (For location: string) -> (double, double) {
switch location {
case "Eiffel Tower": var lat = 48.8582;
var lon = 2.2945;
case "Great Pyramid": var lat = 29.9792;
var lon = 31.1344;
case "Sydney Opera House": var lat = 33.8587;
var lon = 151.2140;
default: var lat = 0;
var lon = 0;
}
return (lat, lon)
}
1 Answer
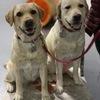
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Michael,
You have a few problems in your code:
First, your external argument name is 'For' but the task wanted 'for'.
Next, you have listed your types in all-lowercase, but Swift types have capitalised first letters.
Next, your variables are scoped to within the switch statement only. To make them available to your return statement you need to define them before the switch statement and then set their values within the switch statement, such as in the following example:
let (left, right): (Int, Int) // declare the names
switch input_value {
case "foo" : (left, right) = (1, 2); // set the value
case "bar": (left, right) = (3, 4);
default: (left, right) = (5, 6)
}
return (left, right) // return the value
Note that I've used constants instead of variables because the value will never change once it is set within the function. Using let instead of var when the value is not going to be mutated is preferred (for performance reasons) and Xcode will emit a warning when you've used var for code where the value is not mutated.
Next your tuples are formatted incorrectly, they should be enclosed in parens and comma separated, you've left off the parens and used semicolons as separators (the semicolons should only be used to separate the case statements)
You might find that building up your code in Xcode's Playground before copy-pasting it into the challenge workspace might help you as Xcode will point out a lot of syntax errors as you are writing them.
Hope this helps,
Alex
Michael Meadows
2,402 PointsMichael Meadows
2,402 PointsThank you so much, this helps a lot!