Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial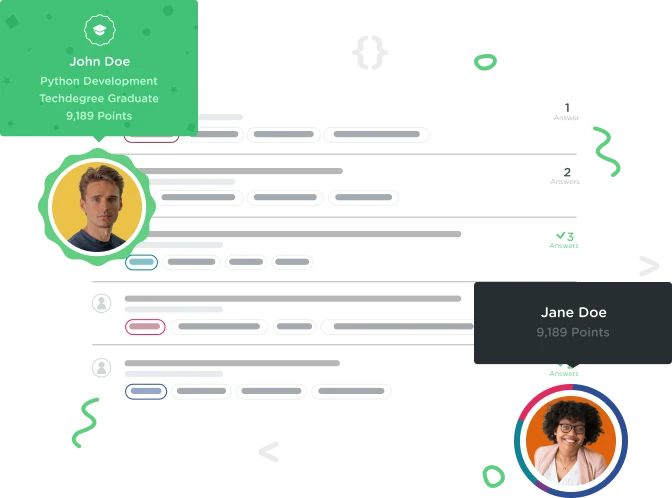

Sam Davidoff
5,295 PointsMaking the artists' names show up correctly
I've been following the IOS videos to the T but have had difficulty in making my artists name link with the library file.
Here is my detail view
import UIKit
class PlaylistDetailViewController: UIViewController {
var playlist: Playlist?
@IBOutlet weak var playlistCoverImage: UIImageView!
@IBOutlet weak var playlistTitle: UILabel!
@IBOutlet weak var playlistDescription: UILabel!
@IBOutlet weak var playlistArtist0: UILabel!
@IBOutlet weak var playlistArtist1: UILabel!
@IBOutlet weak var playlistArtist2: UILabel!
override func viewDidLoad() {
super.viewDidLoad()
if playlist != nil {
playlistCoverImage.image = playlist!.largeIcon
playlistCoverImage.backgroundColor = playlist!.backgroundColor
playlistTitle.text = playlist!.title
playlistDescription.text = playlist!.description
playlistArtist0.text = playlist!.artists[0]
playlistArtist1.text = playlist!.artists[1]
playlistArtist2.text = playlist!.artists[2]
}
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
here is my master view
//
// ViewController.swift
// Algorhythm
//
// Created by Sam Davidoff on 1/26/15.
// Copyright (c) 2015 Wavey Media Group. All rights reserved.
//
import UIKit
class PlaylistMasterViewController: UIViewController {
@IBOutlet weak var playlistImageView0: UIImageView!
override func viewDidLoad() {
super.viewDidLoad()
let playlist = Playlist(index: 0)
playlistImageView0.image = playlist.icon
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "showPlaylistDetail" {
let playlistDetailController = segue.destinationViewController as PlaylistDetailViewController
playlistDetailController.playlist = Playlist(index: 0)
}
}
@IBAction func showPlaylistDetail(sender: AnyObject) {
performSegueWithIdentifier("showPlaylistDetailSegue", sender: sender)
}
}
and here is the music library, appreciate the help
import Foundation
struct MusicLibrary {
let library = [
[
"title": "Rise and Shine",
"description": "Get your morning going by singing along to these classic tracks as you hit the shower bright and early!",
"icon": "coffee.pdf",
"largeIcon": "coffee_large.pdf",
"backgroundColor": ["red": 255, "green": 204, "blue": 51, "alpha": 1.0],
"artists": ["American Authors", "Vacationer", "Matt and Kim", "MGMT", "Echosmith", "Tokyo Police Club", "La Femme"]
],
[
"title": "Runner's Rampage",
"description": "Hit the track hard and get in beast mode with everything from dance tracks to classic hip hop. All the right fuel to motivate you to push your limits.",
"icon": "running.pdf",
"largeIcon": "running_large.pdf",
"backgroundColor": ["red": 255, "green": 102, "blue": 51, "alpha": 1.0],
"artists": ["Avicii", "Calvin Harris", "Pitbull", "Iggy Azalea", "Rita Ora", "Drake", "Lil Wayne"]
],
[
"title": "Joy Ride",
"description": "Let this eclectic playlist take you wherever your heart desires. Cruise along in style to these energetic beats.",
"icon": "helmet.pdf",
"largeIcon": "helmet_large.pdf",
"backgroundColor": ["red": 153, "green": 102, "blue": 204, "alpha": 1.0],
"artists": ["Afrojack", "Kid Cudi", "Daft Punk", "Icona Pop", "Gesaffelstien", "Roksnoix", "deadmau5"]
],
[
"title": "In The Zone",
"description": "Keep calm and focus. Shut out the noise around you and grind away with some mind sharpening instrumental tunes.",
"icon": "laptop.pdf",
"largeIcon": "laptop_large.pdf",
"backgroundColor": ["red": 51, "green": 153, "blue": 204, "alpha": 1.0],
"artists": ["Dr. Dre", "Snoop Dogg", "Com Truise", "D12", "Flying Lotus", "Kanye West", "Rundfunk"]
],
[
"title": "Button Masher",
"description": "Turn up the speakers and get out of my way! The ultimate gaming playlist to get you hyped up and ready for the crazy fun that’s about to happen.",
"icon": "joystick.pdf",
"largeIcon": "joystick_large.pdf",
"backgroundColor": ["red": 51, "green": 204, "blue": 102, "alpha": 1.0],
"artists": ["Skrillex", "The Game", "2 Chainz", "Jay Z", "T.I.", "Rihanna", "Eminem"]
],
[
"title": "Futbal Remix",
"description": "There’s nothing like the world’s game. Kick around the field with this eclectic playlist from around the world. Futbal for life.",
"icon": "ball.pdf",
"largeIcon": "ball_large.pdf",
"backgroundColor": ["red": 255, "green": 102, "blue": 153, "alpha": 1.0],
"artists": ["Shakira", "Santana", "Wyclef Jean", "Aloe Blacc", "Pitbull", "Enrique Iglesias", "Ricky Martin"]
]
]
}
1 Answer
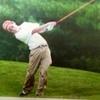
kjvswift93
13,515 PointsHere is your problem- You referenced the segue identifier in two different ways. They must be identical.
override func prepareForSegue(segue: UIStoryboardSegue, sender: AnyObject?) {
if segue.identifier == "showPlaylistDetail" {
let playlistDetailController = segue.destinationViewController as PlaylistDetailViewController
playlistDetailController.playlist = Playlist(index: 0)
}
}
@IBAction func showPlaylistDetail(sender: AnyObject) {
performSegueWithIdentifier("showPlaylistDetailSegue", sender: sender)
}
the if statement uses "showPlaylistDetail" as the identifier, and the performSegueWithIdentifier method passes "showPlaylistDetailSegue" as the identifer.