Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial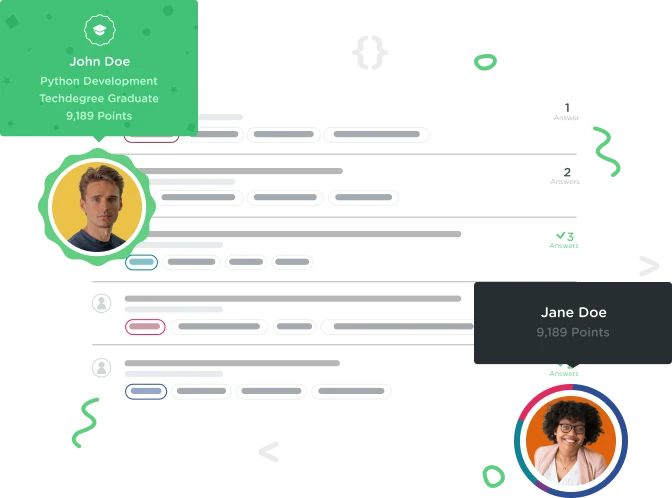
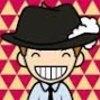
Aaron Selonke
10,323 PointsMaking this same request in Vanilla JavaScript (instead of using jQuery)
HI there, I'm following along with Dave using Ajax to connect to the Flickr API. No problems getting desired results with the $.getJSON and $.ajax methods.
I want to see how to make this request using normal JavaScript, I think I am just missing the syntax of referensing the data that is sent with the GET request. for this API it is an Object.
$(document).ready(function(){
var url = "https://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?";
function pictures(data)
{
console.log(data);
}
$('button').click(function(){
$('button').removeClass('selected');
$(this).addClass('selected');
var animal = $(this).text();
var sendData = { tags:animal, format:"json" };
$.ajax(url,{
type:"GET",
dataType:"json",
data:sendData,
success: pictures
});
basically if translating this Ajax request from jQuery to pure JavaScript where do I reference the variable above called 'sendData'?
Here is what I have:
var xhr = new XMLHttpRequest();
xhr.onreadystatechange = function(){
if(xhr.readyState === 4 && xhr.status === 200)
{
var returnObject = JSON.parse(xhr.responseText);
console.log(returnObject);
}
}
xhr.open('GET', url);
xhr.send();
3 Answers
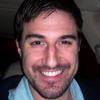
Spencer Snyder
4,030 PointsAaron,
I believe that in this instance jQuery recognizes the CORS stop and then creates a new <script> tag in your header, it then reads the data into this script tag and then into your own javascript. I believe this is related to how jsonp works. I am not entirely sure about this statement, and please correct me if I am wrong(anyone who reads this). But jQuery ajax method is just much more advanced than your xhr. However, trust that your code is good, and that the flickr server is rejecting you , not because of your code, but because you are requesting from an ourside domain.
You may want to get an API key and figure out what to do with it so that flickr authorizes your requests.
Sorry, I wasn't more helpful!
cheers, Spencer
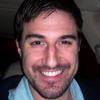
Spencer Snyder
4,030 PointsFrom reading the jQuery ajax documentation (http://api.jquery.com/jquery.ajax/) you can see that the data setting turns your sendData object into a query string that looks like "tags=animal&format=json" and then appends that onto the end of your url string.
The easiest solution in my opinion here is to set your url variable to have those params added to the end of it OR create another string variable like
var sendData="tags=animal&format=json" ;
url+=sendData;
xhr.open('GET', url); ....
Let me know if that works for you or if you need further clarification!
Spencer
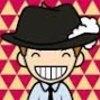
Aaron Selonke
10,323 PointsNo, but thanks for the pointer
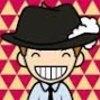
Aaron Selonke
10,323 PointsThanks for the pointer but it didn't work. I got the No-Access-Control-Allow Origin.
XMLHttpRequest cannot load https://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=?tags=Cat&format=json. No 'Access-Control-Allow-Origin' header is present on the requested resource. Origin 'http://port-80-j8ya3yz877.treehouse-app.com' is therefore not allowed access.
When using the jQuery $.Ajax method, the GET request looks like this: Request
URL:https://api.flickr.com/services/feeds/photos_public.gne?jsoncallback=jQuery311007204148311048675_1479747828202&tags=Cat&format=json&_=1479747828203
Request Method:GET
Aaron Selonke
10,323 PointsAaron Selonke
10,323 PointsBasically I'm going to stick with the jQuery.Ajax() method from here on out.