Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial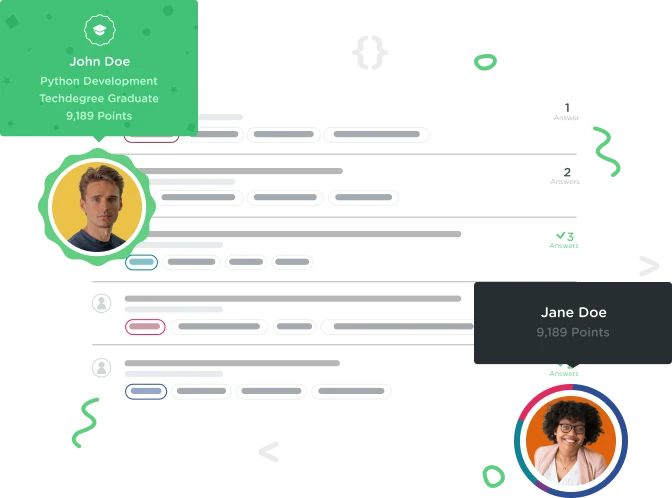

Chris Feltus
3,970 PointsManipulating the DOM using .querySelector Question part II
I am trying to understand how to use .querySelector
better, and made some simple HTML to target with it in javascript. The two variables I defined below are both returning `null
. Would appreciate any pointers, thanks
<!DOCTYPE html>
<html>
<head>
<title>I hate querySelector</title>
</head>
<body>
<p>Misty is the leader of the water gym</p>
<p>She uses a Staru pokemon</p>
<p class='lead-sentance'>Her pokemon are weak to electricity</p>
<p id='demo'>This text will be replaced with a queryselector</p>
<div>
<p>Misty wears overalls</p>
</div>
<a href='http://ign.com' target='ign.com' class='fancy'>visit IGN.com</a>
<script type="text/javascript" src="snakes.js"></script>
<h3>My Favorite Pokemon</h3>
<ul id="incomplete-tasks">
<li><input type="checkbox"><label>Ghastly</label><input type="text"><button class="edit">Edit</button><button class="delete">Delete</button></li>
</ul>
</body>
</html>
var label = document.querySelector('[label="ghastly"]');
console.log(label);
//returns null
var listItem = document.getElementById('incomplete-tasks');
var editInput = listItem.querySelector('input[type=text]');
console.log(editInput)
//throws error 'uncaught TypeError: cannot read property querySelector of null'
1 Answer

Lorenzo Minto
13,898 PointsOn the first query selector the syntax is wrong: square brackets are used to target attributes and 'label' is not an attribute but actually an element itself. Plus in javascript there's not a way to target elements based on their inner HTML so you should probably give a title or a name to the label so you can then reference to it, if you want to go a step further there's a possible way to target elements based on their inner HTML in jQuery, a very powerful javascript library. On the second query selector you forgot the quotes around "text". Try this:
var editInput = listItem.querySelector('input[type="text"]');