Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial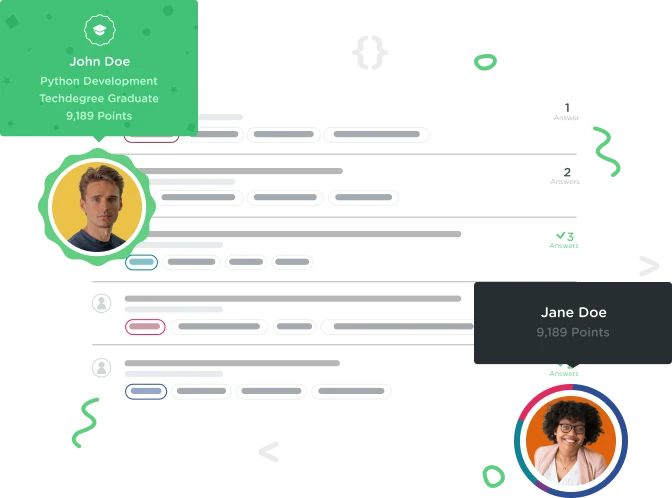

Demetris Christofi
1,819 PointsMap interface does not working properly
Hi,
I really cannot understand what is the error in my code below.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
import java.util.HashMap;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Map<String,Integer> getCategoryCounts()
{
Map<String,Integer> categoryCounts = new HashMap<String,Integer>();
for (BlogPost blogPost : mPosts) {
for(String category : blogPost.getCategory()){
Integer count = categoryCounts.get(category);
if (count==null){
count = 0;
}
count++;
categoryCounts.put(category,count);
}
}
return categoryCounts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
}

Demetris Christofi
1,819 PointsHi Mark,
Here is the error received:
error: for-each not applicable to expression type for(String category : blogPost.getCategory()){ ^ required: array or java.lang.Iterable
Both classes are from the JCF objective exercise.
2 Answers

Seth Kroger
56,413 PointsgetCategory() returns a single String, not an array or collection. This means that BlogPosts only have one category each and there is no need to iterate over an collection of categories. If you replace the 2nd for loop with String category = blogPost.getCategory()
it should work perfectly.

Demetris Christofi
1,819 PointsThank you Seth. I really didn't think about not iterating over a collection and just grab directly the category by calling the method.
It works perfectly. Cheers.

markmneimneh
14,132 PointsThe problem is here
for (BlogPost blogPost : mPosts) {
for(String category : blogPost.getCategory()){
Integer count = categoryCounts.get(category);
if (count==null){
count = 0;
}
count++;
categoryCounts.put(category,count);
}
}
Note this: for(String category : blogPost.getCategory()){
blogPost.getCategory() is a String
But is should be a collection
Please see the method signature for getCategory ... it returns a String
public String getCategory() { return mCategory; }
mCategory is a String
you may want to rethink your logic
Thanks
markmneimneh
14,132 Pointsmarkmneimneh
14,132 PointsHello
what is the error that you are seeing? also, include you Main class as the error could be originating from there.
Thanks