Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial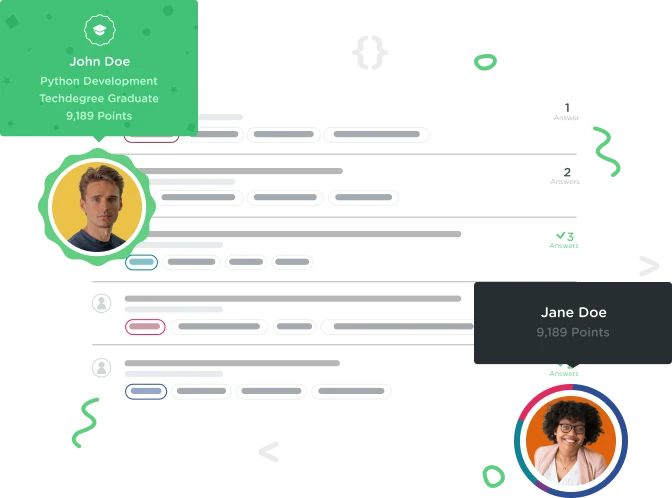
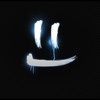
Grigorij Schleifer
10,365 PointsMap problem ...
I cant solve the last "Using the Map to store the Contact method" challenge
Can someone help me ?
My code is:
package com.example.model;
import java.util.Map;
import java.util.Set;
import java.util.TreeSet;
import java.util.HashMap;
public class Contact {
private String mFirstName;
private String mLastName;
private Map<String, String> mContactMethods;
private Set<String> contactMethodSet;
public Contact(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
/* This stores contact methods by name
* eg: "phone" => "(555) 555-1234"
*/
mContactMethods = new HashMap<String, String>();
contactMethodSet = new TreeSet<String>();
}
public void addContactMethod(String method, String value) {
// TODO: Add to the contact method map
mContactMethods.put(method, value);
}
/**
* Returns the available contact methods. eg: phone, pager,
* @return The name of the contact methods that are available
*/
public Set<String> getAvailableContactMethods() {
// FIXME: This should return the current contact method names.
Set<String> contactMethodSet = mContactMethods.keySet();
return contactMethodSet;
}
/**
* Returns the value for the contact method if it exists,
*
* @param methodName The name of the contact method to look up.
* @return The name of the contact methods that are available
*/
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
mContactMethods.get(methodName);
return methodName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
Thank you so much ....
2 Answers
Christopher Augg
21,223 PointsHello Grigorij,
You are very close. Just remember that when we call methods that returns a value, we need to assign it to a variable unless we call the method where it can be used.
public String getContactInfo(String methodName) {
// Do I need to assign this to a String variable when I call the get method?
mContactMethods.get(methodName);
// Of course, you could also just call the method as part of the return
// the methodName String is not what you want. The value that is returned from calling get is.
return methodName;
}
I hope these hints help, but let me know if not and I will elaborate more.
Regards,
Chris
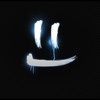
Grigorij Schleifer
10,365 PointsIt doesnΒ΄t work :(
I get the error:
Called getContactInfo with "pager" and expected "(222)222 222 22" but instead got "pager"
:( :( :(
Christopher Augg
21,223 PointsHeya Grigorij,
Sorry to hear that. Let's get it fixed. I used the code that you provided and pasted it into the challenge on step 3. You had the following:
public String getContactInfo(String methodName) {
// FIXME: return the value for the passed in *methodName*
mContactMethods.get(methodName);
return methodName;
}
I then followed the hints I provided like this:
public String getContactInfo(String methodName) {
// You could also assign to a String first and then return the String
// but this works fine
return mContactMethods.get(methodName);
}
Did you do this? Did you change any other code? Please let me know if this helps or not. And if not, please post your entire code again.
Regards,
Chris