Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial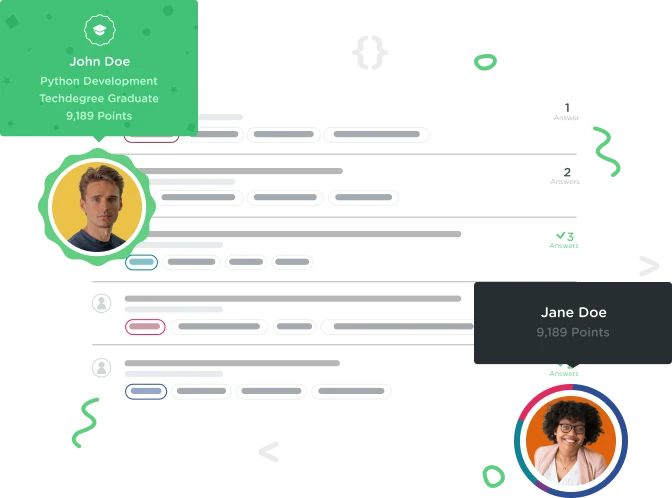

Emily Strobl
5,429 Pointsmaps challenge
Create a function named area() that takes a single argument which will be a two-member tuple. area() should return the result of multiplying the first item in the tuple by the second item in the tuple.
i dont understand the task at all
dimensions = [
(5, 5),
(10, 10),
(2.2, 2.3),
(100, 100),
(8, 70),
]
2 Answers
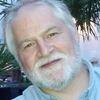
Jeff Muday
Treehouse Moderator 28,720 PointsA tuple is a data type that is similar to a list that contains multiple data items. A tuple differs from a list because it is immutable, that is, once defined it cannot change or be added to.
What the challenge is asking-- create a function "area()" that multiplies the first two items in a tuple and returns the value.
Here is an example that was done with an interactive Python REPL-- The ">>>" indicates where I typed something in to the interactive shell.
Python 3.7.4 (tags/v3.7.4:e09359112e, Jul 8 2019, 20:34:20) [MSC v.1916 64 bit (AMD64)] on win32
Type "help", "copyright", "credits" or "license()" for more information.
>>> # let's name a tuple 'y' and put in three city names
>>> y = ("Chicago", "Denver", "Portland")
>>> # let's access the first or [0] item.
>>> y[0]
'Chicago'
>>> y[1]
'Denver'
>>> # show that we can assign a new variable 'cities' to the value of 'y'
>>> cities = y
>>> cities[0]
'Chicago'
>>> cities[1]
'Denver'
>>> cities[2]
'Portland'
>>> # show that if we try to access an item that is not in the list, we get an error
>>> cities[3]
Traceback (most recent call last):
File "<pyshell#7>", line 1, in <module>
cities[3]
IndexError: tuple index out of range
>>> # suppose we have a simple dimension tuple variable named "x"
>>> # note, a tuple is typically defined using parenthesis.
>>> x = (5,5)
>>> # we can easily create a function that can multiply the first and second items of the dimension
>>> def area(dim):
return dim[0] * dim[1]
>>> # now, let's calculate an "area" of the dim variable
>>> area(x)
25
>>>
I hope this helps your understanding! Good luck with your Python journey!

Zimri Leijen
11,835 Pointsa tuple in python is a collection of data, for example:
("apple", "banana", "cherry")
is a tuple with 3 items.
You are asked to make a function that takes a tuple (with two values as input) and will give the result of multiplying the two values in that tuple as a result.
That function should be named area()
Emily Strobl
5,429 PointsEmily Strobl
5,429 Pointsthis helped a lot thank you
Ryan McGuire
3,758 PointsRyan McGuire
3,758 PointsSuch a great explanation!