Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial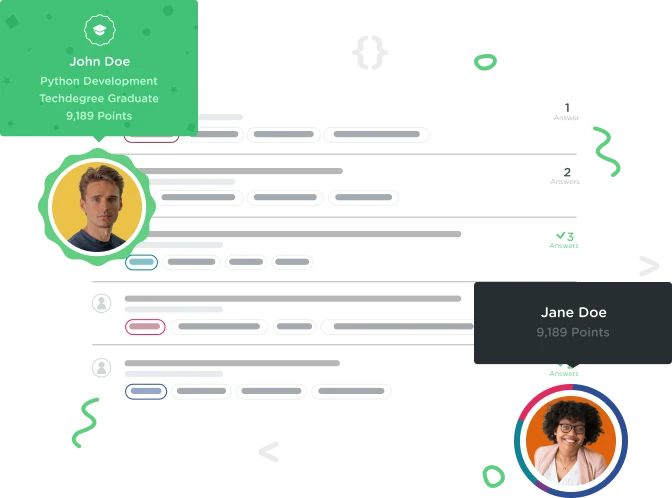

Paul Joiner
1,682 Points(Maps video lesson) Example.java won't compile even though I've followed the video step by step. What's going on?
import java.util.Arrays;
import java.util.ArrayList;
import java.util.Date;
import java.util.Set;
import java.util.HashMap;
import java.util.HashSet;
import java.util.TreeSet;
import java.util.List;
import java.util.Map;
import com.teamtreehouse.Treet;
import com.teamtreehouse.Treets;
public class Example {
public static void main(String[] args) {
Treet[] treets = Treets.load();
System.out.printf("There are %d treets. %n",
treets.length);
Set<String> allHashTags = new TreeSet<String>();
Set<String> allMentions = new TreeSet<String>();
for (Treet treet : treets) {
allHashTags.addAll(treet.getHashTags());
allMentions.addAll(treet.getMentions());
}
System.out.printf("Hash tags: %s %n", allHashTags);
System.out.printf("Mentions: %s %n", allMentions);
Map<String, Integer> hashTagCounts = new HashMap<String, Integer>();
for (Treet treet : treets) {
for (String hashTag : treet.getHashTags()) {
Integer count = hashTagCounts.get(hashTag);
if (count == null){
count = 0;
}
count++;
hashTagCounts.put(hashTag, count);
}
}
System.out.printf("Hash tag counts: %s %n", hashTagCounts);
Map<String, List<Treet>> treetsByAuthor = new HashMap<String, List<Treet>>();
for (Treet treet : treets) {
List<Treets> authoredTreets = treetsByAuthor.get(treet.getAuthor());
if (authoredTreets == null) {
authoredTreets = new ArrayList<Treet>();
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
}
authoredTreets.add(treet);
}
System.out.printf("Treets by author: %s %n", treetsByAuthor);
System.out.printf("Treets by nickrp: %s %n", treetsByAuthor.get("nickrp"));
}
}
2 Answers

Seth Kroger
56,413 Points Map<String, List<Treet>> treetsByAuthor = new HashMap<String, List<Treet>>();
for (Treet treet : treets) {
List<Treets> authoredTreets = treetsByAuthor.get(treet.getAuthor());
//-----------^^^ This should be List<Treet> like the rest.
if (authoredTreets == null) {
authoredTreets = new ArrayList<Treet>();
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
}
authoredTreets.add(treet);
}

Paul Joiner
1,682 PointsThat worked. Thank you!!

Paul Joiner
1,682 PointsExample.java:46: error: incompatible types: List<Treets> cannot be converted to List<Treet>
treetsByAuthor.put(treet.getAuthor(), authoredTreets);
^
Example.java:48: error: no suitable method found for add(Treet)
authoredTreets.add(treet);
^
method Collection.add(Treets) is not applicable
(argument mismatch; Treet cannot be converted to Treets)
method List.add(Treets) is not applicable
(argument mismatch; Treet cannot be converted to Treets)
I get the same "incompatible types" error for java lines 43 and 45 but can't copy and paste for some reason
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsAnd what is the error message the compiler gives you?