Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial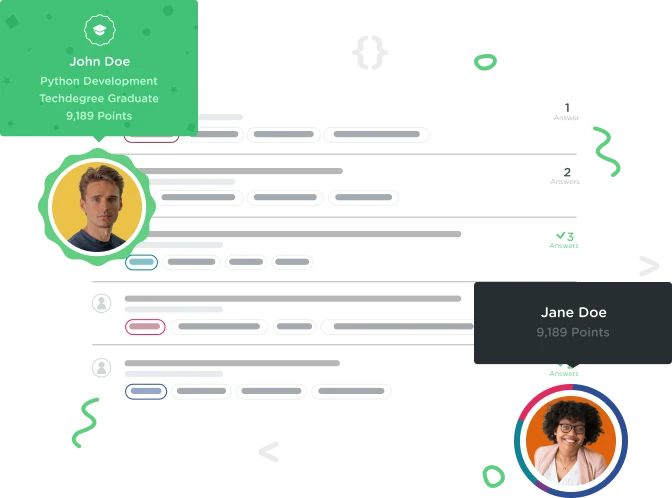
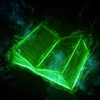
Zain Gill
5,253 PointsMarking items as complete works but not the other way around
i have no idea whats wrong console gives no errors been trying to work it out for 2 hours but couldn't find anything so i came here
// Problem: User interaction doesn't provide desired results.
// Solution: Add interactivity so the user can manage daily tasks.
var taskInput = document.getElementById("new-task"); //new-task
var addButton = document.getElementsByTagName("button")[0]; //first button
var incompleteTasksHolder = document.getElementById("incomplete-tasks"); //incomplete-tasks
var completedTasksHolder = document.getElementById("completed-tasks"); //completed-tasks
//New Task List Item
var createNewTaskElement = function(taskString) {
// Create list item
var listItem = document.createElement("li");
// input (checkbox)
var checkBox = document.createElement("input"); // checkbox
// label
var label = document.createElement("label");
// input (text)
var editInput = document.createElement("input");
// button.edit
var editButton = document.createElement("button");
// button.delete
var deleteButton = document.createElement("button");
// Each elements needs modifing
// Each element needs appending
listItem.appendChild(checkBox);
listItem.appendChild(label);
listItem.appendChild(editInput);
listItem.appendChild(editButton);
listItem.appendChild(deleteButton);
return listItem;
}
// Add a new task
var addTask = function() {
console.log("add task...");
// Create a new list item with the text from #new-task:
var listItem = createNewTaskElement("Some new task");
// Append list item to incompleteTaskHolder
incompleteTasksHolder.appendChild(listItem)
bindTaskEvents(listItem, taskCompleted);
}
// Edit an existing task
var editTask = function() {
console.log("edit task...");
// When the edit Button is pressed
// if the class of the parent is .editMode
// Switch from .editMode
// label text vecome the input's value
// else
// Switch to .editMode
// input value becomes the label's text
// Toggle .editMode on the parent
}
// Delete an existing task
var deleteTask = function() {
console.log("delete task...");
// When the Delete button is pressed
// Remove the parent list item from the ul
}
// Mark a task as complete
var taskCompleted = function() {
console.log("task complete...");
// Append the task list item to the #completed-tasks
var listItem = this.parentNode;
completedTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, incompleteTask);
}
// Mark a task as incomplete
var incompleteTask = function() {
console.log("task incomplete...");
// Append the task list item to the #incompleted-tasks
var listItem = this.parentNode;
incompleteTasksHolder.appendChild(listItem);
bindTaskEvents(listItem, taskCompleted);
}
var bindTaskEvents = function(taskListItem, checkBoxEventHandler) {
console.log("bind list item events")
// select taskListItem's children
var checkBox = taskListItem.querySelector("input[type=checkbox]");
var editButton = taskListItem.querySelector("button.edit");
var deleteButton = taskListItem.querySelector("button.delete");
// bind editTask to edit button
editButton.onclick = editTask;
// bind deleteTask to delete button
deleteButton.onclick = deleteTask;
// bind taskCompleted to checkbox
checkBox.onchange = taskCompleted;
}
// Set the click handler to the addTask function
addButton.onclick = addTask;
// Cycle over incompleteTaskHolder ul list items
for(var i = 0; i < incompleteTasksHolder.children.length; i++) {
// bind events to list item's children (taskCompleted)
bindTaskEvents(incompleteTasksHolder.children[i], taskCompleted);
}
// Cycle over completedTaskHolder ul list items
for(var i = 0; i < completedTasksHolder.children.length; i++) {
// bind events to list item's children (taskIncompleted)
bindTaskEvents(completedTasksHolder.children[i], incompleteTask);
}
1 Answer
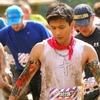
egaratkaoroprat
16,630 PointsHi Zain
I haven't taken this course for a while now... but I think you missed the task binding for the check box. Probably you should try to change line 102 from this
checkBox.onchange = taskCompleted;
to this
checkBox.onchange = checkBoxEventHandler;
The reason for why you use checkBoxEventHandler (comes as a parameter), is that depending if this box is marked as completed it should be bound to the function incompleteTask and the other way around (this will be done by the loop below - from line 109 onwards)
I hope this helps.
Egarat