Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial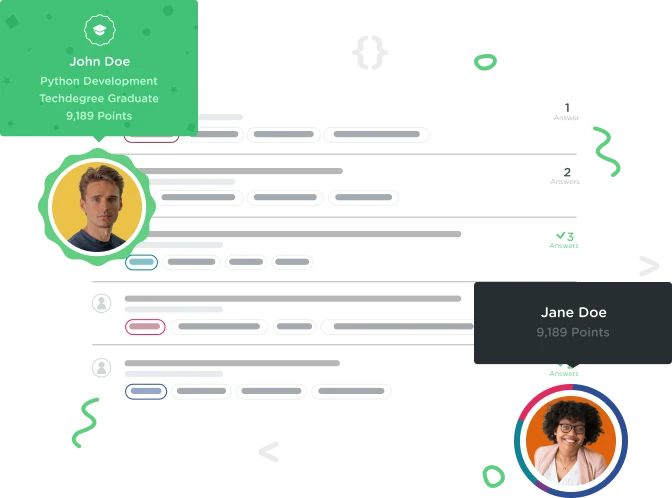
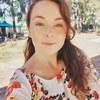
Tanisha Wakefield
9,383 Pointsmasterticket.py ValueError as err
After adding the 'as err' to fix the user issue of inputting too many tickets, when I tested the issue for the blue input again, it comes up with "invalid literal for int() with base 10: 'blue'" instead of the original message.
5 Answers

Josh Stephens
13,529 PointsIf I am reading that error output correctly it appears that you are trying to use a string literal somewhere in which your program is expecting an int. However I concur with Josh Keenan that posting your code might be really useful to help with the debugging of it.

Gia Hoang Nguyen
4,137 Pointsi ran into the same problem and still dont know how to fix it

Josh Keenan
20,315 PointsCan you post the code?

Aimee Malzac
1,727 PointsI have the same problen and I don't know how to fix it
ticket_price = 10 ticket_remaining = 100
while ticket_remaining >= 1: print("There are {} tickets remaining.".format(ticket_remaining)) name = input("What is your name? ") number_tickets = input ("How many tickets do you want, {}? ".format(name)) try: number_tickets = int(number_tickets) if number_tickets > ticket_remaining: raise ValueError ("There is only {} tickets remaining.".format(ticket_remaining)) except ValueError as err: print("Aconteceu um erro. {}. Por favor, tente novamente!".format(err)) else: total_price = (number_tickets) * (ticket_price) print("The total price will be $",total_price,".") ask = input ("Do you wnant to proceed? Y/N ") if ask.lower() == "y": print ("SOLD!") ticket_remaining -= int(number_tickets) else: print("No problem! Thanks {}.".format(name)) print("there is no more tickets available")

Josh Keenan
20,315 PointsCan you format your code, you can put the ` 3 times at the start and end of your code, and after the 3 backticks at the top of your code put python, without a space, check the markdown cheatsheet if this makes no sense
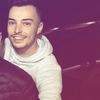
Ash Skelton
3,745 PointsI'll post the code on behalf of the others as I'm also experiencing this problem. Following the challenge exactly as Craig has, this (invalid literal for int() with base 10: 'blue') error only began to happen after using the raise ValueError within the try.
So before this, if you entered a non numerical answer of how many tickets you wanted (i.e. Blue), it would print the "we ran into an issue" error and loop back. Now after adding the ValueError for requesting tickets above the number remaining, it breaks the flow of presenting the correct error message.
I'm pretty certain it's because it's now trying to print the error with {} variable formatted to {err}. However, that {err} variable hasn't been set because it did not pass through the if num_tickets > tickets remaining
statement as it was a non numerical value that was entered (blue).
The order I believe it's now going in:
- It sets num_tickets to blue (based on user input)
- Goes through the try statement and attempts to convert Blue to an int which is not possible.
- Skips the if statement because a ValueError has been raised and tries to print the ValueError defined as {err}.
- Can't print the {err} part of the message because it did not go through the if statement which sets the ValueError message hence why it's still printing the manually written text within the print() statement.
I am pretty new to Python but familiar with reading other languages, so although I can see what's happening, I have no idea how to fix it or do it in the correct order.
TICKET_PRICE = 10
tickets_remaining = 100
while tickets_remaining >= 1:
print("There are {} tickets remaining".format(tickets_remaining))
name = input("What is your name? ")
# Expect a ValueError to happen and handle it appropriately... remember to test it out!
num_tickets = input("How many tickets would you like, {}? ".format(name))
try:
num_tickets = int(num_tickets)
# Raise a ValueError if the request is for more tickets than are available.
if num_tickets > tickets_remaining:
raise ValueError ("There are only {} tickets remaining".format(tickets_remaining))
except ValueError as err:
#include the error text in the output.
print("Oh no, we ran into an issue. {}. Please try again!".format(err))
else:
price = num_tickets * TICKET_PRICE
print("The price is: £{}".format(price))
proceed_YN = input("Do you want to proceed? Y/N ")
if proceed_YN.lower() == "y":
# TODO: Gather credit card information and process it.
print("SOLD!")
tickets_remaining -= num_tickets
else:
print("Thank you anyways, {}".format(name))
print("Tickets are sold out!")

Josh Stephens
13,529 PointsAfter watching the video I think I understand the confusion. So the code is correct and something you would generally see. What it is doing in that video is capturing for two potential issues
The first issue that this code fixes If the user attempts to enter too many tickets you Raise a ValueError with the Message of "There are only <some_number> tickets remaining". So what this does is basically instantiate ValueError and gives it a message. Then because you caught ValueError below and bound err to the ValueError when you go to print out err you basically did the same as str(ValueError)
The second issue that this code fixes and what I think the source of the confusion is, you are basically entering in "blue" into the number tickets and then when you attempt to cast it to an int you get the Value error of invalid literal for int() with base 10: 'Blue' which is normal. So then that gets added into the Oh no, we ran into an issue. invalid literal for int() with base 10: 'Blue'. Please try again!.
I don't know if this helps but I am hoping a small demo might clear things up
import sys; print('Python %s on %s' % (sys.version, sys.platform))
sys.path.extend(['/home/jstephens/PycharmProjects/python_playland'])
PyDev console: starting.
Python 3.7.4 (default, Oct 29 2019, 09:18:11)
[GCC 9.2.1 20191008] on linux
>>> ValueError("Some Issue")
ValueError('Some Issue')
>>> try:
... raise ValueError("Some Issue")
... except ValueError as err:
... print(err)
...
Some Issue
>>> try:
... int('blue')
... except ValueError as err:
... print(err)
...
invalid literal for int() with base 10: 'blue'
>>> try:
... int('blue')
... except ValueError as err:
... # Catching ValueError but adding my own custom message
... print('Why did you enter blue')
... print(f'If I printed err it would have been: {err}')
...
Why did you enter blue
If I printed err it would have been: invalid literal for int() with base 10: 'blue'

Kathy Kinsella
1,306 Pointswhen I enter a number larger than 100, I am getting this error while trying to run the same code. invalid literal for int() with base 10:
Here is what my code looks like. why am I getting the above message?
SERVICE_CHARGE = 2 TICKET_PRICE = 10
tickets_remaining = 100
create the calculate price function. It takes number of tickets and returns
num_tickets * TICKET_PRICE
def calculate_price(number_of_tickets):
# create a new constant for the 2 dollar service charge
#Add the service charge to our result
return (number_of_tickets * TICKET_PRICE) + SERVICE_CHARGE
while tickets_remaining >= 1:
print("There are {} tickets remaining.".format(tickets_remaining))
name = input("What is your name?" )
num_tickets = input("How many tickets would you like, {}? ".format(name))
# expect a ValueError to happen and handle it appropriately...remember to test it out.
try:
num_tickets = int(num_tickets)
#Raise ValueError if the request is for more tickets than are available
if num_tickets > tickets_remaining:
raise ValueError("There are only {} tickets remaining".format(tickets_remaining))
except ValueError as err:
#Include the error text in the output
print("Oh no, we ran into an issue. {}. Please try again".format(err))
else:
amount_due = calculate_price(num_tickets)
print("The total due is ${}".format(amount_due))
should_proceed = input("Do you want to proceed Y/N ")
if should_proceed.lower()=="y":
#TODO: gather credit card information and process it.
print("SOLD!")
tickets_remaining -= num_tickets
else:
#Thank them by name
print("Thank you anyways, {}!".format(name))
print ("Sorry the tickets are all sold out!!:(")
Josh Keenan
20,315 PointsJosh Keenan
20,315 PointsCan you post your code?