Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial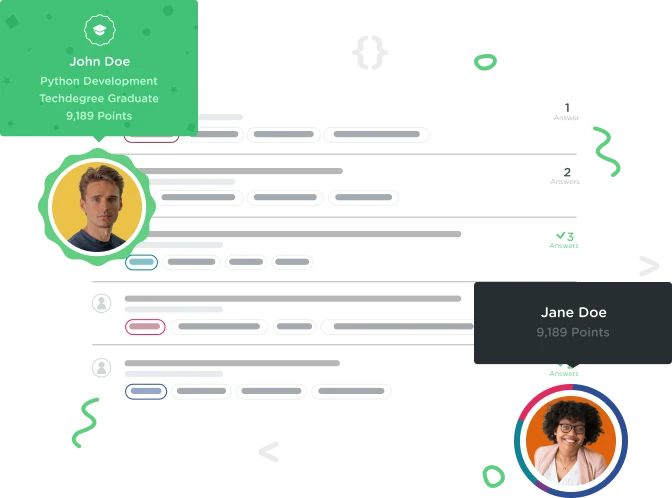
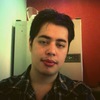
Jonathan Fernandez
8,325 PointsMatching up password Fields in Sign Up
I'm trying to get password fields to match up in the sign up of an app I'm creating. The code feels like it should make perfect sense, although every time I keep getting stuck in an "else if" statement that is meant to check the passwords fields and can't move on to creating the account.
Shown below is my code:
<p>
// SignUp.m
// ...
// User clicks Sign Up Button
- (IBAction)signup:(id)sender {
//Setting variables and triming characters.
NSString *password = [self.passwordField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
NSString *passwordCheck = [self.passwordCheckField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
NSString *email = [self.emailField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
NSString *screenName = [self.screenNameField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
NSLog(@"Password: %@, PasswordCheck: %@", password, passwordCheck);
//Situational Checking.
if ([password length] == 0 || [passwordCheck length] == 0 || [email length] == 0) {
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Oops!" message:@"Make sure you enter all required fields!" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alertView show];
} else if (password != passwordCheck) { //Can't get past this..
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Oops" message:@"Make sure that both passwords are the same!" delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alertView show];
} else {
// New User Created
PFUser *newUser = [PFUser user];
newUser.username = email;
newUser.email = email;
newUser.password = password;
newUser[@"screenName"] = screenName;
// Checking if there are any problems in backend..
[newUser signUpInBackgroundWithBlock:^(BOOL succeeded, NSError *error) {
if (error) {
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Sorry!" message:[error.userInfo objectForKey:@"error"] delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil];
[alertView show];
} else {
[self.navigationController popToRootViewControllerAnimated:YES];
}}];
}
}
//..
</p>
It's really frustrating because I have even set up an NSLog to log the variables. I have made a 2 test to see if they are different or if they are the same.
NSLog
Test 1 (Test with different string input): 2014-07-19 13:52:42.642 App[12541:60b] Password: Test, PasswordCheck: TestDifferent Result: UIAlertView for different Passwords shows up. (This is expected because "Test" != "TestDifferent")
Test 2 (Test with same string input): 2014-07-19 13:52:50.526 App[12541:60b] Password: Test, PasswordCheck: Test Result: UIAlertView for different Passwords shows up. (It shouldn't show up when both "Test" == "Test")
So if the NSLog in test 2 shows that they have the same value, why can I not get past the "else if" statement and go on the making the account in "else"?
3 Answers

muqbilhashi
1,498 Pointstry
else if (![passwordCheck isEqualToString:password]) {
UIAlertView *alertView = [[UIAlertView alloc] initWithTitle:@"Oops"
message:@"Make sure that both passwords are the same!"
delegate:nil
cancelButtonTitle:@"OK"
otherButtonTitles:nil];
[alertView show];
}

Muhammad Nadat
Full Stack JavaScript Techdegree Student 27,165 Pointsinstead of using the != operator, you can try to check if the passwords match. for example
if ([string1 isEqualToString: string2])
NSLog (@"Strings match");
else
NSLog (@"Strings do not match");
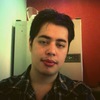
Jonathan Fernandez
8,325 PointsHey guys thank you so much for your feedback. Both answers you have provided work and I can now compare passwords in my app. I will go with Muqbil Hashi's code as it suits my coding style more. I usually like the last else statement to always be the part where things were successful.
Although it still bugs me as to why you can just throw 2 variable strings in if statements and compare them with != or == . So I did some googling and came across this link that helped explained as to why != for my passwords was working. I'll be posting it here in case anyone else comes across this thread and happens to have the same problem as me.