Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial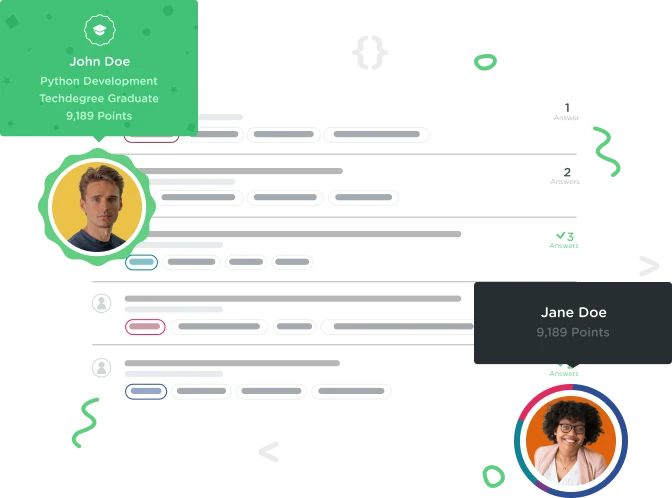

Patricio Vargas
4,665 Pointsmath formula
Suppose M=20 users are sharing B Mbps link. Also suppose each user requires b=0.2 Mbps when transmitting, but each user transmits only p=2% of the time. Suppose packet switching (i.e. statistical multiplexing) is used. Find the minimum value of B such that the probability of packet queuing is less than 0.5%. You may assume B is a multiple of b, i.e. if b is 0.2 Mbps, B would be 0.2 Mbps, 0.4 Mbps, 0.6 Mbps, etc. Also, you may assume 1 Mbps = 10^6 bps. this is the formula I need to code 1 - Σ^n ,x=0 (m!/x!*(m-x)!) p^x (1-p)^m-x
my code in JAVA, I'm not guetting the result I should, am I doing the math right?
import java.util.*;
public class queuing{
//get factorial
int m = 20;//20
double b = .2;//.2
double p = .02;//.2
double prob = .05;//.5
int notque = 0;
int not = 1-notque;
double B = 0;
public static int factorial(int n) {
if (n <= 1) {
return 1;
}
else {
return n * factorial(n-1);
}
}
public String math(){
while((1-notque) > prob){
B += .2;//.2
notque = 0;
double n = Math.round(B/b);
for(int i = 0; i<=n ;i++ ){
double mfact = factorial(m);//
double nfact = factorial(i);//
double msns = factorial(m-i);//
double nCr = (mfact)/(nfact*msns) ;//;nfact*factorial((int)msns)
double pow1 = Math.pow(p,i);
double inactive = m-i;
double pow2 = Math.pow((1-p),inactive);
notque += nCr * pow1 * pow2;
}
}
return "speed of " + B + " users " + m + " transmitting at " + b + " Mbps " + Math.round(p*100);
}
public static void main(String []args){
queuing x = new queuing();
System.out.println(x.math());
}
}
1 Answer

Seth Kroger
56,413 PointsFirst, you have notqueue as an integer but are comparing 1-notqueue to a value of 0.02. I think a double is more appropriate for this value.
Second, and this error is more subtle and harder to see, int isn't big enough to handle the results of the factorial method. The primitive number types like int, long, etc. all have a fixed number of digits. int is 32 bits (binary digits) long. In decimal terms it can hold a value between about +/- 2 billion (2 x 10^9). 20! by comparison is approximately 2.4 x10^18. Factorial is know to blowup very big, very fast and the results of trying to stuff too big a number into a int is undefined.
Details of the limits of each type: https://docs.oracle.com/javase/specs/jls/se8/html/jls-4.html#jls-4.2
There is a built-in class java.math.BigInteger for arbitrarily big integer numbers you could use intsead. You could also use double if you're not concerned about an "exact" answer. Floating point types have a wider range of values by using a fixed number of significant digits, 14 for a double IIRC, and an exponent. 14 digits of accuracy is good enough a lot of cases (but not all).