Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial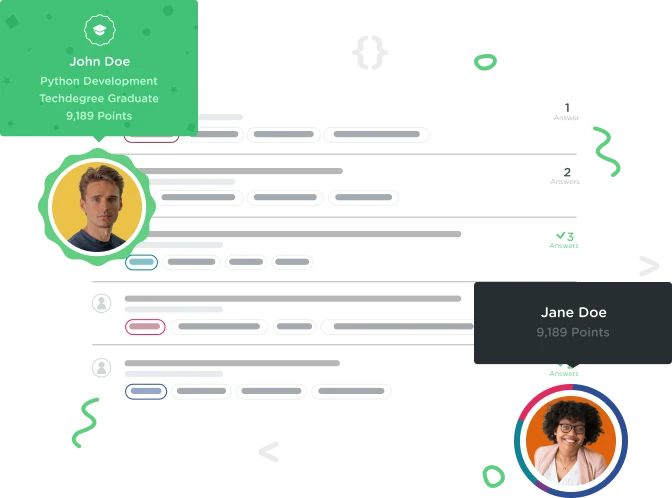

Brandyn lee murtagh
432 PointsMeet objects quiz - add a public constructor
Hi guys, back with another question. I am currently working through the quiz on adding a constructor. Below is my code.. can any one help? and also explain what is wrong with my code if possible.
Add a public constructor to the GoKart.java class that allows the parameter color to be passed in. In the constructor store the argument color in the private color field.
public class GoKart { private String mColor;
public GoKart(String Color) { mColor = Color; }
public String getColor() { return mColor; } }
Thanks
5 Answers
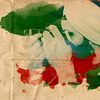
Gunjeet Hattar
14,483 PointsWell as for what I'm guessing you need to create a new object using the new keyword
So in your main method use
className any_name_for_object = new className();
the className() is the default constructor. But since you created one with a parameter it will become
className any_name_for_object = new className(String parameter);
This is just the skeleton, try replacing with the values and check if it works. If you get any errors let us know.
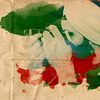
Gunjeet Hattar
14,483 PointsGo*Kart gokart = new Gok*art("red");
GoKart is spelt with a capital K on the left and with a small k on the right. :D ..
Be careful with typos in Java.

Brandyn lee murtagh
432 Pointsdone it man.. thanks!
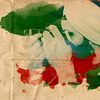
Gunjeet Hattar
14,483 PointsGreat!
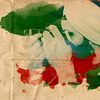
Gunjeet Hattar
14,483 PointsEverything seems fine.
Though I believe the question asks you to use color and not Color as a variable. I know it shouldn't make a difference but it does in these quizzes .
Also in the question its private private String mColor = "red";
Omitting that will also cause an error. Again these have nothing to do with actual programming outside these quizzes but to get through the quiz you will have to pay attention to the casing of variables
Hope this helps

Brandyn lee murtagh
432 PointsHi mate, thanks for the reply. Any chance you would be able to help in the second part? Please create a new GoKart object. As you know it takes a single parameter, color. ?

Brandyn lee murtagh
432 PointsAhh it still doesn't work.. my code is shown in the below: public class Example {
public static void main(String[] args) {
GoKart gokart = new Gokart("red");
System.out.println("We are going to create a GoKart");
}
}
Efe รzelรงi
3,776 PointsInstead of writing "red" between parentheses, you should define new string as a "red" then write the string name between parentheses without using "".
For example, private String mColor = "red"; GoKart gokart = new GoKart(mColor);

MUZ140566 Kundai Katambetambe@gmail.com
4,845 Pointspublic class Example {
public static void main(String[] args) { System.out.println("We are going to create a GoKart"); GoKart gokart = new GoKart("red"); } }
Brandyn lee murtagh
432 PointsBrandyn lee murtagh
432 PointsAhh okay, I done it like this - GoKart gokart = new Gokart(); . With that being said do I just have to add the string parameter in the () ?
Gunjeet Hattar
14,483 PointsGunjeet Hattar
14,483 PointsExactly this way is the first way of doing it. Like I mentioned in the comment
Since you created a constructor with a parameter it should read
GoKart gokart = new Gokart("Yellow");
I've put yellow but it can be any string value
Remember the format should match
public GoKart(String Color) -- pay attention to String color, this is what you pass in.
Giancarlo M.
1,502 PointsGiancarlo M.
1,502 PointsOMG, thank you so much! The skeleton really helped! I wish there more of them!