Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial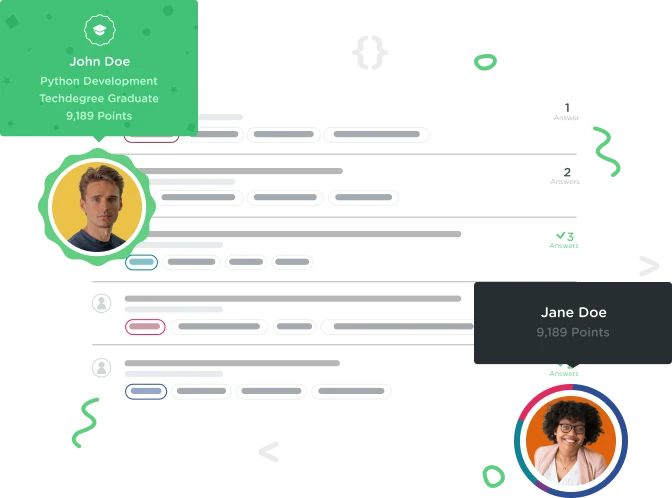

name564681351614
3,497 PointsMember Variable
Is a member variable just a variable that's defined within a class?
2 Answers

Ken Alger
Treehouse TeacherEric;
In a nutshell, yes.
Whew! That was a quick answer... okay, let's do a bit of a deeper dive into this. The official Java documentation on declaring Member Variables goes into it a bit more detail but, honestly, it wasn't enough for me. After a variety of sources of research here is how I kind of sum it up:
When we define a class, we can give that class member variables. These variables are members of that class. Take, for example, the following class declaration.
public class SomeClass {
int x;
int y;
}
SomeClass
has two member variables, x
& y
. When we define an object instance of SomeClass
it will still have two member variables, x
& y
. We can reference these members using the '.' character.
// Create an instance of SomeClass
SomeClass obj = new SomeClass();
// Assign new values to x & y
obj.x = 1;
obj.y = 42;
These variables belong to `SomeClass`, and are known as member variables. On the other hand, if we declare variables inside of a function, we call these local variables. These variables are local to a particular function, and not publicly accessible. For example, in the following function, we have no way of accessing the obj variable outside of the main(String[]) function.
```java
public static void main (String args[])
{
SomeClass obj = new SomeClass();
System.out.println ("X" + obj.x);
}
So, in the nutshell, a member variable is a variable that belongs to an object, whereas a local variable belongs to the current scope.
Hopefully that makes some sense. Please post back if you are still stuck on this concept. It is an important one as you progress through OOP languages.
Happy coding,
Ken
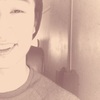
Jason S
16,247 Pointsshouldn't myClass() be SomeClass()?

Ken Alger
Treehouse TeacherDoh... Yes.
Harry Jones
2,964 PointsHarry Jones
2,964 Points"So, in the nutshell, a member variable is a variable that belongs to an object, whereas a local variable belongs to the current scope."
That line right there is perfect. Thanks :)