Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial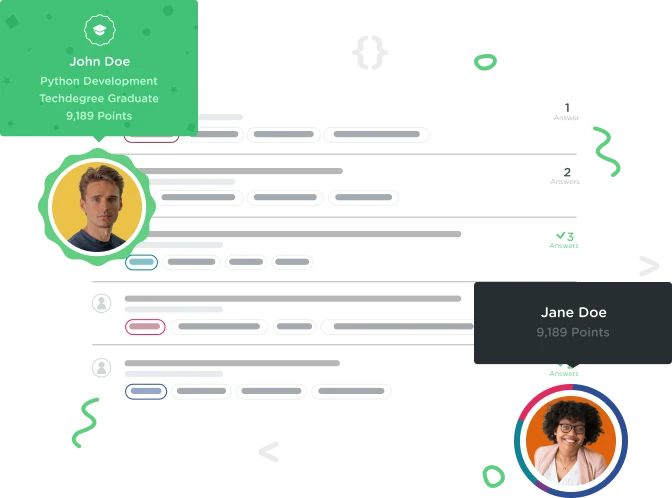
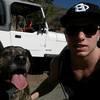
Hunter G
6,612 PointsMembership Challenge. Confused how functions recognize arguments. .
So I actually did some scouting and found the correct answer to this problem on the forums already which was very useful..BUT what I do not understand is how the arguments of the 'members' function is recognizing the values of 'my_dict' & 'my_list' even though the 'members' function arguments are 'the_dict' & 'the_list' . See how they were switched from 'my' to 'the' ? That is what i'm confused about. Anyways, the code below is the code I found on another forum post which was the correct answer. I put a comment on the line of def members function as well as the for and if loops to make my question further understandable. Thank you for taking the time to read this and help! :)
my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
def members(the_dict, the_list): # i used my_dict & my_list and it still returned correct
count = 0
for key in the_dict: # again, i used my_dict
if key in the_list: # again, i used my_list
count = count + 1
return count
2 Answers

Mike Kryski
2,625 PointsMy python is a little bit rusty, and I haven't done the coding challenge, so I'm not sure exactly what was required, but I'll try to help.... I think the reason you're getting confused, is that the variable names and the arguments names were the same.
In this example, you declared two variables:
my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
The variable names don't really matter, they could have just as easily been something like:
fruity_dictionary = {'apples': 1, 'bananas': 2, 'coconuts': 3}
yummy_list = ['apples', 'coconuts', 'grapes', 'strawberries']
These variables are then passed to the function when the function is called. This isn't included in the code above, but is how it would be validated.
members(fruity_dictionary, yummy_list)
When you define a function that requires arguments, you need to give those arguments a name, just like with variables. An argument in a function definition is simply a placeholder for the variables that you pass to it. In this example, the argument names were:
def members(the_dict, the_list)
Again, they could have been named whatever you wanted, like:
def members(thing1, thing2)
OR, in your case
def members(my_dict, my_list)
It's better to have descriptive names so you can quickly understand what's going on, but the point is that it doesn't really matter.
Hopefully that sheds a bit of light for you.

Mike Kryski
2,625 PointsI added to my answer a bit, but I'll clarify here:
A function is basically a block of code that you would want to use over and over again. Lets say that we want to write a function that adds two numbers together, and returns the result. This is how we might write it:
#declare a few variables
num1 = 25
num2 = 100
num3 = 15
#define our method, we'll call it add_these_up. Our argument names will be x and y.
def add_these_up(x, y):
sum = x + y
return sum
# to use the function, we need to call it and pass some variables. We can even save the result in another variable!
new_number = add_these_up(num1, num2)
another_new_number = add_these_up(num1, num3)
# new_number now has a value of 125, and another_new_number has a value of 40
In this example, we defined a function that will add two numbers. We then called it twice, and placed the result in two new variables. We can define functions anywhere in our code, and then use them later. It doesn't matter if we have variables, or other functions, defined before it.
Hope this helps.

Mike Kryski
2,625 PointsThe function knows which variable to use, because you need to explicitly tell it which ones to use when you call the function. If what you pass to the function is not what it is expecting, then it will give an error.
# Using the same example above, if we only passed in one variable, we would likely get an error.
some_number = add_these_up(num2) # this won't work
OR
some_number = add_these_up(num1, num2, num3) # this probably won't work either, or at least not how you want it too
Hunter G
6,612 PointsHunter G
6,612 PointsMike Kryski,
First of all I would like to say thank you for responding! Anyways, this is definitely starting to make more sense and you've clarified most of it for me BUT I still have a question. . Say you define numerous variables prior to the function definition and you place an argument into the function. . how does the function know which variable to use as the argument? Do you define it inside of the function properties below? If you are understanding what i'm asking, please give an example with your answer! :)