Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial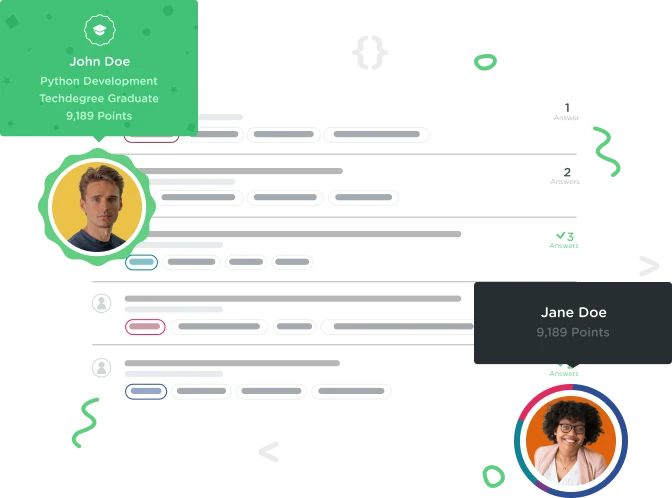

Jeremy Bradrick
6,731 PointsMembership Challenge in Dictionaries. Is it possible to do something like for each _ in _ do _
Is it possible to do something like, for each item in the list that's also in the dict, increment the number by 1, return the value of the number?
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
def members(my_dict, my_list):
my_dict = {"first_name":"Robert","middle_name":"Elias","last_name":"Lee"}
my_list = ["first_name", "social_security", "middle_name", "age", "last_name"]
keys_in_dict = 1
for my_list in my_dict:
keys_in_dict += 1
return keys_in_dict
1 Answer
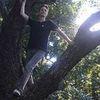
gyorgyandorka
13,811 PointsYou’re on the right track, that is exactly what you should do in order to solve the challenge. However, the execution (pun intended) is not correct yet.
Your function should work for any (legal) argument, not arbitrary ones. In your version you've set those arguments to some arbitrary value inside your function, and worked on those reassigned variables, but you shouldn't have. (Treehouse uses that example list and dictionary (above in the comment) passed in as arguments as a test case, that's why there is an expected result, 2 in this case)
So, let's try again: we have to initialize some "counter" variable, correct, but since we’ll increment it by 1 after we find the first matching item, we should start from 0.
def members(my_dict, my_list):
items_in_both = 0
...
Now we can iterate through the items on the list with a for
loop, and inside the loop we can make a condition check - if the item is among the dictionary keys (remember: ... in my_dict
will iterate through the keys by default, but you could make it more explicit by using ... in my_dict.keys()
), then we increment our counter variable.
(Note: we could work in the other direction, of course - iterate through the dictionary keys with our for
loop, and then compare those values with the items on the list.)
...
for item in my_list:
if item in my_dict:
items_in_both += 1
So, the final solution is simple as this (the funny thing is that the code can be readed aloud - almost - as your „pseudocode”):
def members(my_dict, my_list):
items_in_both = 0
# for each item in the list...
for item in my_list:
# that is also in the dict...
if item in my_dict:
# increment the number by 1...
items_in_both += 1
# ...and in the end, return the number:
return items_in_both
Now you probably understand why your code returned 4 instead of 2: you iterated through the my_dict
which you defined inside the function, with 3 key-value pairs, and you incremented the counter - with a starting value of 1 - each time by 1.
You actually never touched the original argument values, or even the reassigned my_list
inside your function; you simply counted all the keys in the dictionary (defined inside the function, by you), and added 1 to the result.
Jeremy Bradrick
6,731 PointsJeremy Bradrick
6,731 PointsWhen I run this, it says "expected 2, returned 4"