Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial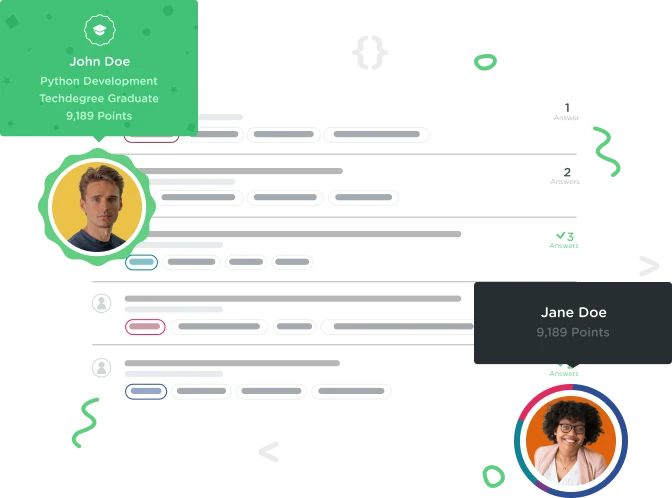

Frank Sherlotti
Courses Plus Student 1,952 PointsMembership python challenge, need help I'm confused.
Question says it all. I don't know what i'm doing and i'm basically looking for someone to clean up this mess and explain what i'm doing wrong.
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
def members(a_dict, a_list):
a_dict = {'weather': 1, 'orange': 2, 'red': 3, 'green': 4}
a_list = ['blue', 'orange', 'red', 'wet']
count = 1
for members in a_dict:
a_dict['weather']['orange']['red']['green']
if members in a_list:
a_list == a_dict
count += 1
return count
3 Answers

Dan Johnson
40,532 PointsYou'll want to remove these two lines:
a_dict = {'weather': 1, 'orange': 2, 'red': 3, 'green': 4}
a_list = ['blue', 'orange', 'red', 'wet']
Even if you were testing with them. You don't want to overwrite the values being passed in.
The line:
a_dict['weather']['orange']['red']['green']
Would required a nest of dictionaries four levels deep. While the dictionary could have other dictionaries as values (and so on) you can't count on it.
Your for loop and if statements are correct, but you don't need the check:
a_list == a_dict
All this would do is compare the dictionary against the list and then discard the result.
All that being said you actually have all the code you need already:
def members(a_dict, a_list):
# Start at 0. There might not be any similarities.
count = 0
for members in a_dict:
if members in a_list:
count += 1
# Watch for indentation
return count

Jeremy Fisk
7,768 Pointsconsider a couple of things:
1) pull your variables for this challenge out of the function, and define them once above the function call (except for count)
2) initiate count at count = 0
3) the first check can be for key in dict
4) immediately following, remove your second line of a_dict
5) then check if your key is in your list again, just as in your code
6) finally increment count
just as you have it and return that number

Frank Sherlotti
Courses Plus Student 1,952 PointsThanks really do appreciate the help!

Scott Simontacchi
2,071 PointsInteresting! Didn't Kenneth mention a more syntactically Pythonic way of incrementing at some point that was better than += 1? I can't seem to remember...
Frank Sherlotti
Courses Plus Student 1,952 PointsFrank Sherlotti
Courses Plus Student 1,952 PointsThanks again I really appreciate it! Is that example on the top always there to confuse me? Because I feel like it confuses me more then it helps.
Dan Johnson
40,532 PointsDan Johnson
40,532 PointsJust remember the sample input and output in the comments is there to clarify what you should be doing with the data, but it likely won't be the data passed in.