Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial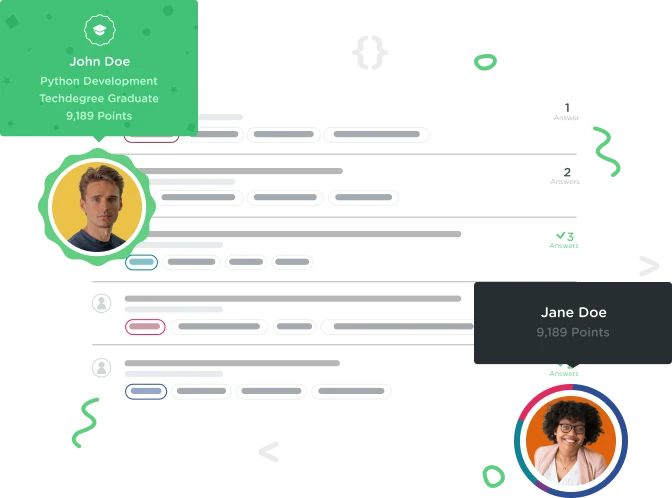

Darryl Amatsetam
6,141 PointsMemory leak in php
Hi, i've been typing the following code to connect to the database. However, I keep getting this error:
Fatal error: Out of memory (allocated 1846542336) (tried to allocate 65488 bytes) in C:\xampp\htdocs\zephineshop\inc\products.php on line 39
Here's my code:
database.php
<?php
function get_connection() {
try {
$db = new PDO("mysql:host=" . DB_HOST . ";dbname=" . DB_NAME .";port=" . DB_PORT,DB_USER,DB_PASS);
$db->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
$db->exec("SET NAMES 'utf8'");
return $db;
} catch (Exception $e) {
echo "Could not connect to the database.";
exit;
}
}
function return_all() {
try {
$db = get_connection();
$results = $db->query("SELECT * FROM products ORDER BY sku;");
$products = $results->fetchAll(PDO::FETCH_ASSOC);
} catch (Exception $e) {
echo "Data could not be retrieved from the database.";
exit;
}
return $products;
}
function return_by_sku($sku) {
try {
$db = get_connection();
$result = $db->prepare("SELECT * FROM products WHERE sku = ?;");
$result->bindParam(1, $sku);
$result->execute();
$product = $result->fetch(PDO::FETCH_ASSOC);
} catch (Exception $e) {
echo "Data could not be retrieved from the database.";
exit;
}
return $product;
}
function return_subset($start, $end) {
try {
$db = get_connection();
$results = $db->prepare("SELECT * FROM products
ORDER BY sku LIMIT ?, ?;");
$results->bindParam(1, $start);
$results->bindParam(2, $end);
$results->execute();
$products = $results->fetchAll(PDO::FETCH_ASSOC);
} catch (Exception $e) {
echo "Data could not be retrieved from the database.";
exit;
}
return $products;
}
function total_count() {
try {
$db = get_connection();
$count = $db->query("select count(*) from products;");
$total = $count->fetch(PDO::FETCH_ASSOC);
} catch (Exception $e) {
echo "Data could not be retrieved from the database.";
exit;
}
return intval($total);
}
function return_sizes_by_sku($sku) {
$sizes = array();
try {
$db = get_connection();
$result = $db->prepare("SELECT s.size FROM products as p
inner join products_sizes as ps on p.sku = ps.product_sku
inner join sizes as s on ps.size_id = s.id WHERE p.sku = ?
order by s.order;");
$result->bindParam(1, $sku);
$result->execute();
while($i = $result->fetch(PDO::FETCH_ASSOC)){
$sizes[] = $i['size'];
}
} catch (Exception $e) {
echo "Data could not be retrieved from the database.";
exit;
}
return $sizes;
}
products.php
<?php
require_once(ROOT_PATH . "inc/database.php");
/*
* Loops through all the products, looking for a search term in the product names
* @param string $s the search term
* @return array a list of the products that contain the search term in their name
*/
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach($all as $product) {
if (stripos($product["name"],$s) !== false) {
$results[] = $product;
}
}
return $results;
}
/*
* Counts the total number of products
* @return int the total number of products
*/
function get_products_count() {
return total_count();
}
/*
* Returns a specified subset of products, based on the values received,
* using the order of the elements in the array .
* @param int the position of the first product in the requested subset
* @param int the position of the last product in the requested subset
* @return array the list of products that correspond to the start and end positions
*/
function get_products_subset($positionStart, $positionEnd) {
$subset = get_products_subset($positionStart, $positionEnd);
return $subset;
}
/*
* Returns an array of product information matching the sku
* @param int $sku the sku of the product
* @return mixed array list of products information for matching product
*/
function get_single_product($sku) {
$product = return_by_sku($sku);
$sizes = return_sizes_by_sku($sku);
$product['sizes'] = array();
foreach ($sizes as $size) $product['sizes'][] = $size;
return $product;
}
/*
* Returns the full list of products. This function contains the full list of products,
* and the other model functions first call this function.
* @return array the full list of products
*/
function get_products_all() {
$products = return_all();
return $products;
}
1 Answer

Darryl Amatsetam
6,141 PointsGot it! It seemed that the method get_products_subset was refering to itself, therefore running in an endless loop. Which causes memory leaks.