Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial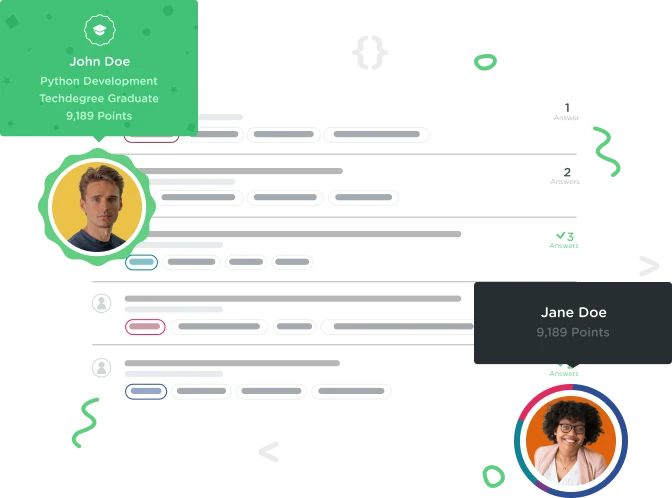
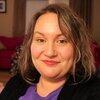
Michelle Walker
785 PointsMessage - choosing translation literal vs. string concatenation?
I used translation literal to form my message, but the solution in the video was string concatenation. How do you choose which one to use in which situations?
My code:
alert ("Let's do some math!");
let number1 = prompt('Type a number:');
let number2 = prompt('Type another number:');
number1 = parseFloat(number1);
number2 = parseFloat(number2);
let message =`<h1>Math with the numbers ${number1} and ${number2}</h1>`
let answer1 = number1+number2
let answer2 = number1 * number2
let answer3 = number1 / number2
let answer4 = number1 - number2
message += `${number1} + ${number2} = ${answer1} <br>
${number1} * ${number2} = ${answer2} <br>
${number1} / ${number2} = ${answer3} <br>
${number1} - ${number2} = ${answer4}`
document.write(message);
2 Answers
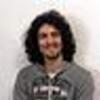
Gergely Bocz
14,244 PointsHi Michelle!
In short, my personal opinion is that template literals are better, because they are a bit easier to use and read.
I think the reason why string concatenation was used in the video, is that the video itself is pretty old, and template literals either hadn't been introduced yet, or got introduced not long before the video's release -- with ES6 in 2015 -- so it wasn't widely supported back then. As you can see on the moz dev page they are universally supported now with the exception of IE.
I tried searching for speed comparison results and found nothing conclusive: in some tests template literals did better, in others string concatenation.
However, template literals in the form of tagged templates can be parsed by functions. See the example at the moz dev page, or here.

Abdurahim S
4,177 PointsHi, I had the same approach and I was wondering why the multi-line strings inside the template literals didn't work on html without the <br> tag. But now I came to the conclusion, that html needs <br> tags anyway. Please proof me if I'm wrong.

Abdurahim S
4,177 PointsMy code looks like this:
alert("Let's do some math!");
let firstNumber = prompt("Please type a number.");
firstNumber = parseFloat(firstNumber);
let secondNumber = prompt("Please type a second number.");
secondNumber = parseFloat(secondNumber);
let message = `<h1>Math with the numbers ${firstNumber} and ${secondNumber}</h1>
${firstNumber} + ${secondNumber} = ${firstNumber + secondNumber} <br>
${firstNumber} * ${secondNumber} = ${firstNumber * secondNumber} <br>
${firstNumber} / ${secondNumber} = ${firstNumber / secondNumber} <br>
${firstNumber} - ${secondNumber} = ${firstNumber - secondNumber}`;
document.write(message);
Michelle Walker
785 PointsMichelle Walker
785 Pointsoh good. They are much easier for me and I was afraid it was a cop out. Thanks so much!
Jillian Lee
Full Stack JavaScript Techdegree Student 4,171 PointsJillian Lee
Full Stack JavaScript Techdegree Student 4,171 Points👍 I agree, I prefer template literals as well. To me, they are much easier to read and write in a program because they flow more like written text. You can also easily see the references to variables.