Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial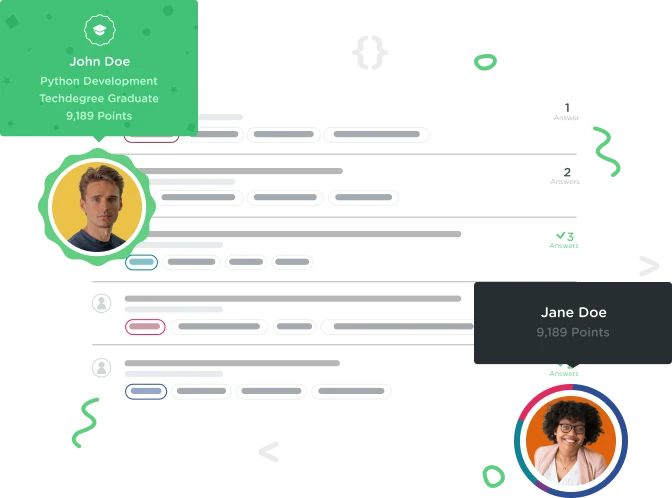

Graham Huxtable
Courses Plus Student 85 PointsMessage form not working.
Hello,
Pulling what's left of my hair out trying to get a message form to function. I have had a few different attempts at getting it to work with no luck. I followed the PHP contact form tutorials, but the message form simply loads a blank white screen when I submit it. If anyone can have a look and point out any glaring errors, it would be much appreciated.
<?php
if ($SERVER_["REQUEST_METHOD"] == "POST") {
$message = trim($_POST["message"]);
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
//Validation for empty message fields.
if ($message == "" OR $name == "" OR $email == "") {
echo "Please enter a message, name and email.";
exit;
}
//Validation for injection attacks into the message field.
foreach ( $_POST as $value ) {
if ( stripos($value,'Content-Type:') !== FALSE ) {
echo "There was an error with the information you entered.";
exit;
}
}
//Validation for the hidden field. If entered by a spambot, the form will not send.
if ($_POST["address"] != "") {
echo "Your form submission contains an error.";
exit;
}
//Calling the phpmailer class which will format the email. Email will not send if this is not pointing at the correct directory.
require_once("/inc/phpmailer/class.phpmailer.php");
$mail = new PHPMailer();
if (!$mail->ValidateAddress($email)) {
echo "Please enter a valid email address.";
exit;
}
$email_body = "";
$email_body = $email_body . $message . "<br><br>";
$email_body = $email_body . "From" . "<br><br>";
$email_body = $email_body . $name . "<br><br>";
$email_body = $email_body . $email;
$mail->SetFrom($email, $name); //States the sender's email address, and their name.
$address = "example@hotmail.com"; //States the email address of the recepiant.
$mail->AddAddress($address, "Example"); //States the name of the website which has sent the form.
$mail->Subject = "Example | " . $name; //Sets the subject of the email, and the name of the sender.
$mail->MsgHTML($email_body);
//An error message will display if the email did not send.
if(!$mail->Send()) {
echo "There was a problem sending the email: " . $mail->ErrorInfo;
exit;
}
//The Location command directs the server to this page after the php script has been completed.
header("Location: /index.sent.html");
exit;
}
?>
5 Answers

Chris Shaw
26,676 PointsHi ,
I'm inclined to say the below line is causing the issue as a forward slash dictates the root directory of the web server.
require_once("/inc/phpmailer/class.phpmailer.php");
Typically if the script is in a location that's relative to your script an relative path will suffice, i.e.
require_once("../inc/phpmailer/class.phpmailer.php");
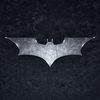
Logan R
22,989 PointsCan you please show us the code for the actual form page?
Also, what this file do?:
header("Location: /index.sent.html");

Graham Huxtable
Courses Plus Student 85 PointsThis is the html code for the form. The index.send.html page is just a thank you page, and also a test to see if I could get the php code to redirect to another page.
<form method='post' action='contact_form.php'>
<p class='content_text'>
<span class='first_content_letter_cf'>C</span><span class='first_content_word_cf'>ontact</span> us by phone, email, or in person with <br>
any queries. Feel free to email us a picture of <br>
your furniture for a rough quote.
</p>
<textarea id='contact_message' type='text' name='message' required>Message
</textarea>
<input id='contact_name' type='text' name='name' placeholder='Name' required>
<input id='contact_email' type='email' name='email' placeholder='Email' required>
<input style='display: none;' id='address' type='text' name='address' placeholder='address'>
<input id='contact_submit' type='submit' name='submit' value='Send'>
</form>
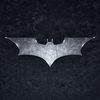
Logan R
22,989 PointsI am not very sure. This is a very misterious bug. Try adding "echo 'hi';" at the top and each step of your code. If no "hi" is echoed when you put it at the top of your PHP file, that means for some reason, your form is not submitting to "contact_form.php".
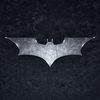
Logan R
22,989 PointsI tried running your code from my web server and it threw an error:
Notice: Undefined variable: SERVER_ in {My directory} on line 2
The line:
if ($SERVER_["REQUEST_METHOD"] == "POST") {
Just thought, did you mean $ _ SERVER? because $ _ SERVER works, $SERVER _ doesn't.

Graham Huxtable
Courses Plus Student 85 PointsThank you for the syntax correction, I'm still very new to php so haven't quite got the grasp of the language yet. I corrected the mistake but there is still an error preventing the page from working. I will try your suggestion of adding in an echo "hi"; after each statement tomorrow, seems like a good way of testing thanks for the tip. I'll let you know what the outcome is.
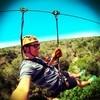
thomascawthorn
22,986 PointsNot sure index.sent.html is the best way... maybe just do message-sent.html.
if ($SERVER_["REQUEST_METHOD"] == "POST") {
should definitely be
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$SERVER is a global variable and cannot be mistyped / reused for any other purpose. I think with $SERVER you're checking something that doesn't exit. Because this if statement returns false, the whole execution code will not run.
There may be other bugs inside the statement, but it definitely won't be getting inside the block with $SERVER_.
To test if you make inside the conditional if statement, try adding this at the top
if ($_SERVER["REQUEST_METHOD"] == "POST") {
echo "MADE IT!";
exit;
// rest of your code
}
If successful, you should see "MADE IT" printed to a blank screen.
If you don't see this after you've corrected $_SERVER, and the method is definitely POST, it's likely there's a syntax error which is breaking the whole page. Go over your code with a fine tooth comb to make sure you've got all your semi-colons etc..

Graham Huxtable
Courses Plus Student 85 PointsOk so I'v added in an echo " " with a number after each statement, and also the echo "MADE IT!"; suggestion. To begin with I was able to get to number 5, after which is this statement:
//Calling the phpmailer class which will format the email. Email will not send if this is not pointing at the correct directory.
require_once("../inc/phpmailer/class.phpmailer.php");
$mail = new PHPMailer();
}
Unfortunately after temporarily removing it, a blank page now displays every time I try to test the form, even after it has been added back and I have reverted to the exact same code which gave me a count of 5. No idea what the problem is now, as I have literally changed nothing else in the code. I have even added in an echo "1"; before any of the code is run, at the very top of the php file, and even this does not display. At a bit of a loss at what to do here...
Edit: With the possibilities being either the phpmailer.php file is corrupt, not being referenced correctly, or mispelt somehow, I will have a look at how to sort them out and write an update if the problem is solved. That is, when I am able to get any response from the form...
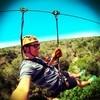
thomascawthorn
22,986 PointsIf at any point you don't get any output to the screen, it's likely there's a syntax error. Even if you didn't change anything.. you must've changed something otherwise it would work perfectly :-p
Are you familiar with command line? If you are, I can give some instructions to exactly where the errors will be on this page.
Take a really good look at opening and closing ( ) and { } and "" and '' as well as semi-colons.
Once you've resolved the syntax error and getting your test messages back, you can put another test message at the top of the php mailer file to see if it's calling correctly. Beware - if you 'require' something that doesn't exist, the page will die. You're saying I 'require' this file to run, so if it can't find the file, it doesn't run! This is another reason for an entire page break.
../ means you're looking up a directory for the inc folder. If the inc folder and this mail.php folder are in the same directory, the ../ is unnecessary.
Let us know how you get on
Graham Huxtable
Courses Plus Student 85 PointsGraham Huxtable
Courses Plus Student 85 PointsThanks for you answer Chris. Unfortunately still the same result, but I will remember this for future work.