Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial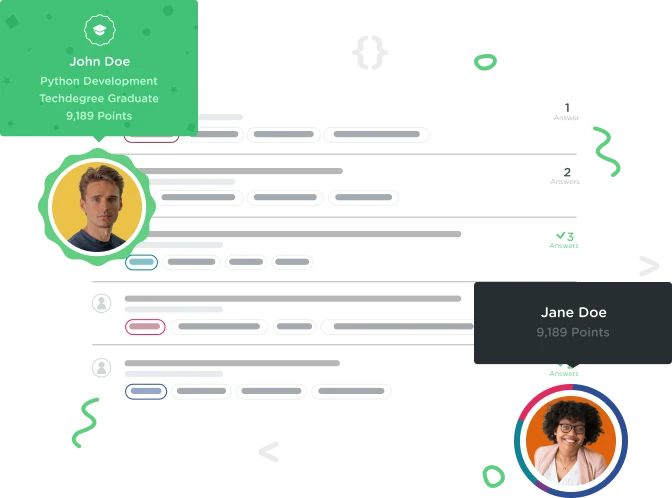
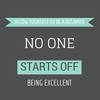
Hammad Nasir
Courses Plus Student 2,801 PointsMessage is sent after a long time, and some times it is never sent.
Hey, I'm following along the 'Build a Self-destructing message android app' tutorial on team treehouse.com
After completing this 'Sending a message' section when I tried to run the app & send the photos and videos, I noticed that the messages are taking a whole-lot time to get to the Parse back-end. I'm unable to figure out what's the problem is.
What the problem possibly be?
Here's my ChooseFriendsActivity.java (RecipientsActivity.java) file's code:
`import android.app.AlertDialog; import android.app.ListActivity; import android.net.Uri; import android.support.v7.app.ActionBar; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.ActionProvider; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.Toast;
import com.parse.FindCallback; import com.parse.Parse; import com.parse.ParseException; import com.parse.ParseFile; import com.parse.ParseObject; import com.parse.ParseQuery; import com.parse.ParseRelation; import com.parse.ParseUser; import com.parse.SaveCallback;
import java.util.ArrayList; import java.util.List;
public class ChooseFriendsActivity extends AppCompatActivity {
ListView list;
public static final String TAG = ChooseFriendsActivity.class.getSimpleName();
protected List<ParseUser> mFriends;
protected ParseRelation<ParseUser> mFriendsRelation;
protected ParseUser mCurrentUser;
protected MenuItem mSendMenuItem;
protected Uri mMediaUri;
protected String mFileType;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_choose_friends);
list = (ListView) findViewById(R.id.choose_friends_list);
list.setChoiceMode(ListView.CHOICE_MODE_MULTIPLE);
mMediaUri = getIntent().getData();
mFileType = getIntent().getExtras().getString(ParseConstants.KEY_FILE_TYPE);
}
@Override
public void onResume() {
super.onResume();
mCurrentUser = ParseUser.getCurrentUser();
mFriendsRelation = mCurrentUser.getRelation(ParseConstants.KEY_FRIENDS_RELATION);
ParseQuery<ParseUser> query = mFriendsRelation.getQuery();
query.addAscendingOrder(ParseConstants.KEY_USERNAME);
query.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> friends, final ParseException e) {
if (e == null) {
mFriends = friends;
String[] usernames = new String[mFriends.size()];
int i = 0;
for (ParseUser user : mFriends) {
usernames[i] = user.getUsername();
i++;
}
ArrayAdapter<String> adapter = new ArrayAdapter<String>(
list.getContext(),
android.R.layout.simple_list_item_checked,
usernames);
list.setAdapter(adapter);
list.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
if (list.getCheckedItemCount() > 0) {
mSendMenuItem.setVisible(true);
} else {
mSendMenuItem.setVisible(false);
}
}
});
} else {
Log.e(TAG, e.getMessage());
AlertDialog.Builder builder = new AlertDialog.Builder(ChooseFriendsActivity.this);
builder.setTitle(R.string.error_title);
builder.setMessage(e.getMessage());
builder.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_choose_friends, menu);
mSendMenuItem = menu.getItem(0);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
//noinspection SimplifiableIfStatement
if (id == R.id.action_send) {
ParseObject message = createMessage();
if (message == null) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setMessage("There was something wrong with the file selected. Please select another file.")
.setTitle("We're Sorry!")
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
} else {
send(message);
finish();
}
return true;
}
return super.onOptionsItemSelected(item);
}
protected ParseObject createMessage() {
ParseObject message = new ParseObject(ParseConstants.CLASS_MESSAGES);
message.put(ParseConstants.KEY_SENDER_ID, ParseUser.getCurrentUser().getObjectId());
message.put(ParseConstants.KEY_SENDER_NAME, ParseUser.getCurrentUser().getUsername());
message.put(ParseConstants.KEY_RECIPIENT_IDS, getRecipientIds());
message.put(ParseConstants.KEY_FILE_TYPE, mFileType);
byte[] fileBytes = FileHelper.getByteArrayFromFile(this, mMediaUri);
if (fileBytes == null) {
return null;
} else {
if(mFileType.equals(ParseConstants.TYPE_IMAGE)) {
fileBytes = FileHelper.reduceImageForUpload(fileBytes);
}
String fileName = FileHelper.getFileName(this, mMediaUri, mFileType);
ParseFile file = new ParseFile(fileName, fileBytes);
message.put(ParseConstants.KEY_FILE, file);
return message;
}
}
protected ArrayList<String> getRecipientIds() {
ArrayList<String> recipientIds = new ArrayList<String>();
for (int i = 0; i < list.getCount(); i++) {
if (list.isItemChecked(i)) {
recipientIds.add(mFriends.get(i).getObjectId());
}
}
return recipientIds;
}
protected void send(ParseObject message) {
message.saveInBackground(new SaveCallback() {
@Override
public void done(ParseException e) {
if (e == null) {
// success!
Toast.makeText(ChooseFriendsActivity.this, "Message sent!", Toast.LENGTH_LONG).show();
}
else {
AlertDialog.Builder builder = new AlertDialog.Builder(ChooseFriendsActivity.this);
builder.setMessage("There was an error sending your message. Please try again.")
.setTitle("We're Sorry!")
.setPositiveButton(android.R.string.ok, null);
AlertDialog dialog = builder.create();
dialog.show();
}
}
});
}
}
`
1 Answer

kunalshitut
5,872 PointsI agree, it takes a lot of time for mobile photos of around 2 -3 MBs to be sent to parse. Some times upto an hour as was in my case.
Luis Cole
17,228 PointsLuis Cole
17,228 PointsHow long does it takes to upload the file? I found out that sending images from the emulator was pretty quick but when i used my phone it takes like 30 seconds. That is because the images captured by the phone are more or less 2 mb compared to 20 kb from the ones captured in the emulator.