Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial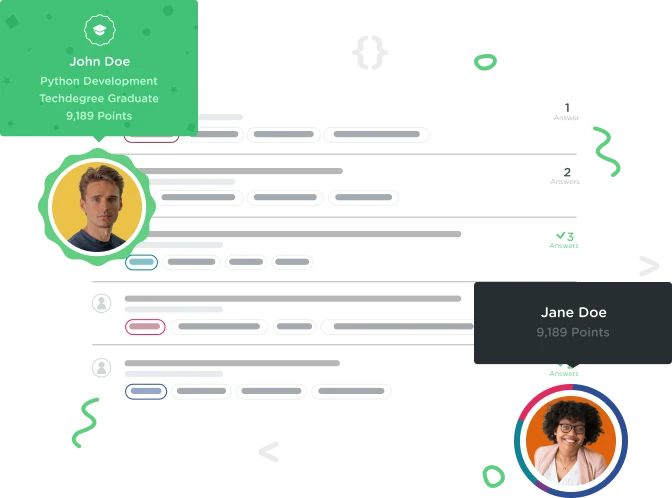

Travis Sly
3,947 PointsMessing around in VS code for practice and ran into error. HELP :)
So i was just trying to mess around in VS code trying out some different concepts in making my first baby "program"
first step is i wanted to make a function that would take 3 arguments: yield, years, amount i want the function to calculate the compound interest earned for an investment at a certain, yield, for a certain number of years.
the code i wrote:
const futureValue = (yield, years, amount,) => {
for (let i = 0; i <= years; i++){
let totalPortfolio = 0;
return totalPortfolio += (amount * (yield + 1));
}
}
console.log(futureValue(.1, 15, 1000));
the issue i keep getting is it will just log value of the return i would have after year one. i cannot figure out what im doing wrong to make it stop instead of running through the full loop iteration.
Is using a parameter as the stopping condition for the loop the issue? or is my logic flawed? idk it makes perfect sense in my head lol
thanks in advance for the help!!
1 Answer
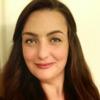
Jennifer Nordell
Treehouse TeacherHi there, Travis Sly! First, I applaud your willingness to experiment on your own! Way to make the material yours. This is a great way and, in my opinion, necessary to make the material stick.
There's a couple of things going on here. First, is the return
statement. A return
statement immediately ends function execution. The function will just stop running wherever it is when it is hit. So it goes through the first iteration and then ends immediately.
So we need to move the return
outside the loop and only return
once the loop has finished its job. But this also brings up the second point. This is the idea of "scope". Because you declared totalPortfolio
inside the loop with let
, it only exists inside the loop. Furthermore, it's being reset to 0 each iteration and I don't think you are meaning to do that. So with some very minor changes in placement, this runs like you want, I believe
const futureValue = (yield, years, amount,) => {
let totalPortfolio = 0;
for (let i = 0; i <= years; i++){
totalPortfolio += (amount * (yield + 1));
}
return totalPortfolio;
}
console.log(futureValue(.1, 15, 1000));
Hope this helps!
Travis Sly
3,947 PointsTravis Sly
3,947 PointsOh wow yes thank you very much! That's exactly what I was after! :D The way you explained it definitely helped solidify scope more, I'll have to keep playing around and practicing!
Travis Sly
3,947 PointsTravis Sly
3,947 Pointscould i trouble you for more input when you get a moment please? so I was trying to expand a little bit on my little future value calculator:
const futureValue = (yield, months, initialValue, monthlyInvestment) => { let totalPortfolio = []; let runningTotal = 0; for (let i = 0; i <= months; i++){ if (i % 12 === 0) { runningTotal += ((yield + 1) * (initialValue + monthlyInvestment)); totalPortfolio.push(
Your Year ${i/12} return is: $${runningTotal}
); } else { runningTotal += ((yield + 1) * (initialValue + monthlyInvestment)); }}
console.log(futureValue(.2, 120, 0, 1000));
the one thing that has me curious is where i am messing up because it eventually logs 'Your Year 10 return is: $145200'
however the correct answer for investing $1k a month compounding on 20% average ROI after 120 months it should be if im not mistaken: $311,504
What am i doing incorrectly here?