Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial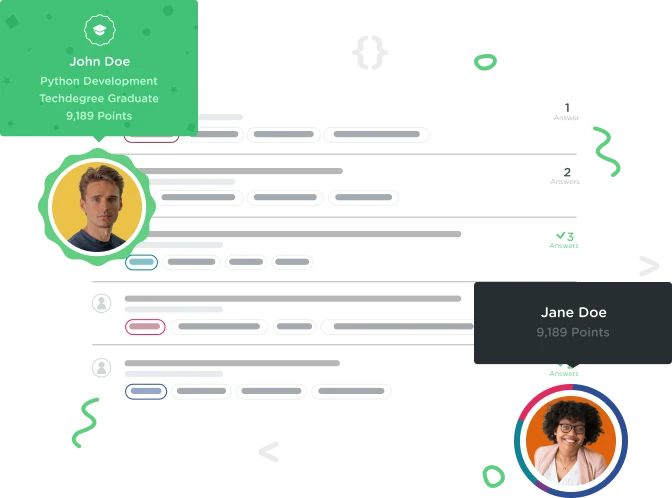
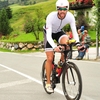
Axel Vuylsteke
2,787 PointsMessy_list
As I want to have some more info on this exercise. The issue is the removal of all non integers (booleans included). For example you want this exercise as a function where the variable given is a list.
def cleanuplist(messy_list)
for value in messy_list:
try:
x = value +1
except TypeError:
messy_list.remove(value)
However booleans are still getting through this result. Any idea how we could do this? The exercise itself is easy to solve by just adding del messy_list[3]. But only helps with small lists where you know the index of the boolean.
2 Answers
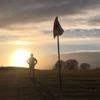
Stuart Wright
41,118 PointsThat is because False is treated as 0 and True as 1 when you try to add them, so neither False + 1 nor True + 1 will result in TypeError.
Also, it is not a good idea to remove items from a list as you iterate over it. For example, if you were to pass the list ['a', 'b', 'c'] into your function, you will see that 'b' still remains at the end. That is because once 'a' is removed, the loop continues on to what it thinks is the next element, but it ends up skipping 'b', because it's now in the position in the list that was previously occupied by 'a'.
The following line of code does what you want it to do, but you're not expected to be able to know this at this stage in the course, so don't worry if it doesn't make too much sense:
messy_list = [e for e in messy_list if type(e) is int]
The following thread might be helpful if you're interested in removing multiple elements from a list with one statement:
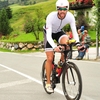
Axel Vuylsteke
2,787 PointsHi Stuart,
Indeed. The type(value) helps me out here ;-) After seeing the slices now it's clearer to me to do:
for value in messy_list[:]:
if (type(value) is int):
pass
else:
messy_list.remove(value)

Mike Morse
2,750 PointsAlex's response is interesting. It seems that the [:] in "for value in messy_list[:]" is key to getting the list item out of messy_list but I'm not sure why. The string and boolean are cleared out with it, so how is it working here on the list?
Christian Rowden
2,278 PointsChristian Rowden
2,278 PointsHere I am at work and I can't wait to get home and start tinkering because of this post. OP I hope you got the answer you were looking for.
Cheers!