Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial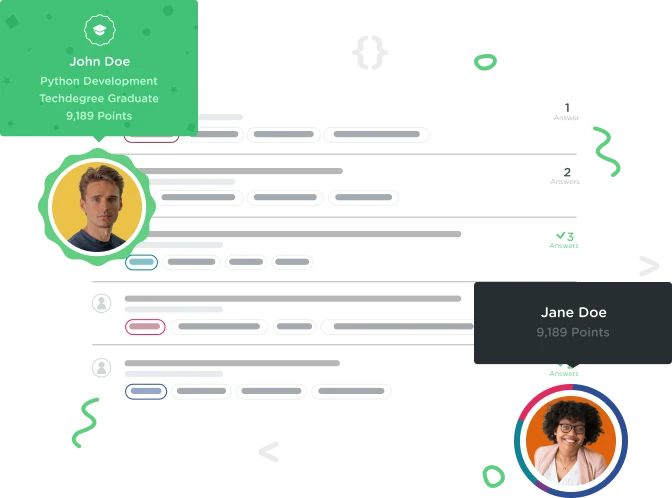
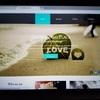
Kyle Jensen
Courses Plus Student 6,293 PointsMethod not functioning as expected - Address book project
I am having some trouble understanding why my code will not return false when an entry in the address book already exists. The boolean method CheckEntry() is used by two other methods - AddEntry() and RemoveEntry() - both are looking to verify if the entry exists. In Add() it is supposed to see if the contact already exists before adding another contact and shouldn't create the contact if it does (but it is adding them). remove() is supposed to check if it exists and uses the value of the stored variable to remove the current contact (but does nothing). I know I'm probably either missing something simple or have waaay over thought the process. My assumption is that check() is not working correctly since both of the functions tied to it are faulting. Anyone have any ideas?? Let me know if I need to explain anything further, too.
Here is all of my code...
namespace AddressBook {
class Contact {
public string Name;
public string Address;
public Contact(string name, string address) {
Name = name;
Address = address;
}
}
}
//-------------------------------------------------------------------------------
using System;
namespace AddressBook {
class AddressBook {
public readonly Contact[] contacts;
public AddressBook() {
contacts = new Contact[2]; ;
}
public void AddEntry(string name, string address) {
Contact AddContact = new Contact(name, address);
if (CheckEntry(name)) {
for (int i = 0; i < contacts.Length; i++) {
if (contacts[i] == null) {
contacts[i] = AddContact;
Console.WriteLine("Address Book updated. {0} has been added!", name);
break;
}
}
}
}
private string existingContact = "";
private bool CheckEntry(string name) {
foreach(Contact contact in contacts) {
if (contact == null) {
break;
}
else if (contact != null && contact.ToString() != name) {
continue;
}
else if (contact.ToString() == name) {
existingContact = contact.ToString();
return false;
}
}
return true;
}
public void RemoveEntry(string name) {
if( !(CheckEntry(name)) ) {
existingContact = null;
Console.WriteLine("{0} removed from contacts", name);
}
}
public string View() {
string contactList = "";
foreach(Contact contact in contacts) {
if(contact == null) {
break;
}
contactList += String.Format("Name: {0} -- Address: {1}" + Environment.NewLine, contact.Name, contact.Address);
}
return contactList;
}
}
}
//-------------------------------------------------------------------------------
using System;
namespace AddressBook {
class Program {
static void Main(string[] args) {
AddressBook addressBook = new AddressBook();
PromptUser();
void Menu() {
Console.WriteLine("TYPE:");
Console.WriteLine("'Add' to add a contact: ");
Console.WriteLine("'Remove' to select and remove a contact: ");
Console.WriteLine("'Quit' to exit: ");
}
void UpdateAddressBook(string userInput) {
string name = "";
string address = "";
switch ( userInput.ToLower() ) {
case "add":
Console.Write("Enter a name: ");
name = Console.ReadLine();
Console.Write("Enter an address: ");
address = Console.ReadLine();
addressBook.AddEntry(name, address);
break;
case "remove":
Console.Write("Enter a name to remove: ");
name = Console.ReadLine();
addressBook.RemoveEntry(name); //why doesnt this work!!??!!
break;
case "view":
Console.WriteLine(addressBook.View());
break;
}
}
void PromptUser() {
Menu();
string userInput = "";
while (userInput != "quit") {
Console.WriteLine("What would you like to do?");
userInput = Console.ReadLine();
UpdateAddressBook(userInput);
}
}
}
}
}
1 Answer
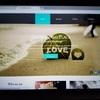
Kyle Jensen
Courses Plus Student 6,293 PointsJon Wood The problem was that it wasn't returning false. I have since figured it out. Though I'm not sure how to use the breakpoints you mentioned. I know that you can use them and how to set it, I've just never done it, and to be honest, hadn't even thought about it. Information about it would be nice, thank you.
Here is what I did to get it working:
- I made a change in Contact.cs adding a property
public string Name { get; private set; }
public string Address { get; private set; }
- I really wanted to eliminate that random variable - the one just outside of CheckEntry(). I didn't like it from the start, but I was so stuck I just did the first thing that came to mind when I should have walked away for a bit. As well, checking the object against the string was, well, not great. So instead of contact.ToString() I used the Contact properties, which I'm not sure why I didn't in the first place, especially since I had them elsewhere.
//original code
private string existingContact = "";
private bool CheckEntry(string name) {
foreach(Contact contact in contacts) {
if (contact == null) {
break;
}
else if (contact != null && contact.ToString() != name) {
continue;
}
else if (contact.ToString() == name) {
existingContact = contact.ToString();
return false;
}
}
return true;
}
//updated code
private bool CheckEntry(string name) {
foreach(Contact contact in contacts) {
if (contact == null) {
break;
}
else if (contact != null && contact.Name != name) {
continue;
}
else if (contact.Name == name) {
return false;
}
}
return true;
}
- so this inherently changes RemoveEntry() to something a bit more logical but, also wasn't tying into the CheckEntry() like I wanted to. This was something I was going to try and figure out after making it work.
//original code
public void RemoveEntry(string name) {
if( !(CheckEntry(name)) ) {
existingContact = null;
Console.WriteLine("{0} removed from contacts", name);
}
}
//updated code
public void RemoveEntry(string name) {
for(int i = 0; i < contacts.Length; i++) {
if(contacts[i].Name == name) {
contacts[i] = null;
break;
}
}
Console.WriteLine("{0} removed from contacts", name);
}
- finally view(). This method orignially had a break under the null conditional. This was not good because if the index 0 in the array was null then there would be no output even though index 1 had a Contact object. It also now lets the user know why nothing was returned if there are no contacts, instead of just empty space and a new prompt asking what to do next.
//original code
public string View() {
string contactList = "";
foreach(Contact contact in contacts) {
if(contact == null) {
break;
}
contactList += String.Format("Name: {0} -- Address: {1}" + Environment.NewLine, contact.Name, contact.Address);
}
return contactList;
}
}
}
//updated code
public string View() {
string contactList = "";
foreach (Contact contact in contacts) {
if(contact == null) {
continue;
}
contactList += String.Format("Name: {0} -- Address: {1}" + Environment.NewLine, contact.Name, contact.Address);
}
return (contactList != String.Empty) ? contactList : "Your Address Book is empty.";
}
- for others to note:
- I had also posted this question on StackOverflow. The response I got was well thought out. I definitely have a love-hate relationship with StackOverflow. Here is the code that was given to me. For anyone not understanding why this is better, please feel free to ask and I will do my best to explain.
public bool AddEntry(string name, string address) {
if (!ContainsEntry(name)) {
Contact AddContact = new Contact(name, address);
for (int i = 0; i < contacts.Length; i++) {
if (contacts[i] == null) {
contacts[i] = AddContact;
Console.WriteLine("Address Book updated. {0} has been added!", name);
return true;
}
}
Console.WriteLine($"Cannot add name ({name}) to Address Book since it is full!");
// TODO: Throw some exception or specific return values to indicate the same to the caller
} else {
Console.WriteLine($"Name ({name}) already exists in Address Book!");
// TODO: Update the address?
}
return false;
}
private int GetEntryIndex(string name) {
for (int i = 0; i < contacts.Length; i++) {
if (contacts[i] != null && contacts[i].Name == name)
return i;
}
return -1;
}
private bool ContainsEntry(string name) {
return GetEntryIndex(name) != -1;
}
public void RemoveEntry(string name) {
var index = GetEntryIndex(name);
if (index != -1) {
contacts[index] = null;
Console.WriteLine("{0} removed from contacts", name);
}
}
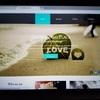
Kyle Jensen
Courses Plus Student 6,293 PointsNOTE: I upvoted my own post because of the answer from StackOverflow. I think others could definitely learn from it.

Jon Wood
9,884 PointsGlad you got it working! I missed the contact.ToString()
! If you're using Visual Studio I'll be glad to help you with breakpoints, if you'd like.
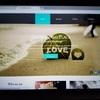
Kyle Jensen
Courses Plus Student 6,293 PointsJon Wood Thank you, I am too. No worries about Contact.ToString(). And, yes, your help would be greatly appreciated.
Jon Wood
9,884 PointsJon Wood
9,884 PointsIt always returns false when you try it? My first thought is that it's only checking the
ToString
of thename
so it seems to be case sensitive in the check. You can also try setting a breakpoint and step through the code when you run it. I can help with that, if you need.