Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial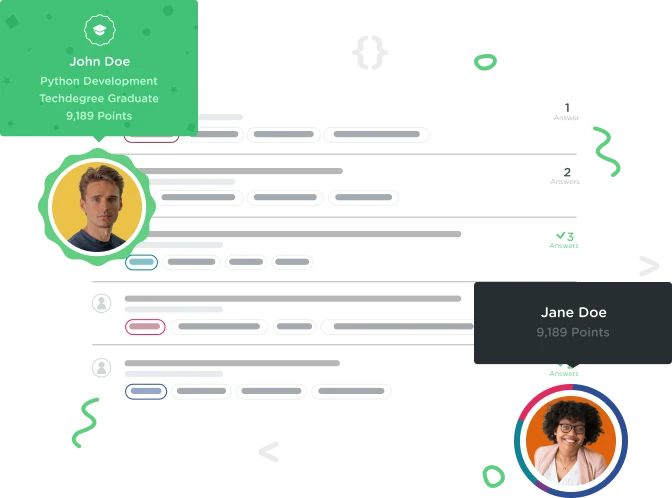

Danny Low
550 PointsMethod overloading
In the GoKart quiz I have been trying to follow the PEZ examples. I understand I am trying to pass one method to another but I still get Task 1 error. Any simplified help appreciated.
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive(int numberOfLaps) {
while (numberOflaps > 0 ){
lapsDriven += numberOfLaps;
barCount -= numberOfLaps;
}
public void drive() {
drive(1);
}
}
}
2 Answers

Charlie Gallentine
12,092 PointsIn your code, the 'while' loop is unnecessary to complete the challenge, the drive method is meant to be called once and increment or decrement by the passed in number of laps:
public void drive(int numberOfLaps) {
lapsDriven += numberOfLaps;
barCount -= numberOfLaps;
}
The drive method you have next, which passes 1 for a default is then correct, however, it looks like your curly braces don't line up and that you are grouping both drive methods into a single larger method. This is probably the bigger issue as it is more syntax heavy:
public void drive(int numberOfLaps) {
while (numberOflaps > 0 ){
lapsDriven += numberOfLaps;
barCount -= numberOfLaps;
}
public void drive() {
drive(1);
}
} //This currently matches the first curly brace after "(int numberOfLaps)"
To complete the challenge, and to properly use method signatures for different functions, the methods need to be two separate methods with the same name and different parameters:
public void drive(int numberOfLaps) {
lapsDriven += numberOfLaps;
barCount -= numberOfLaps;
}
public void drive() {
drive(1);
}
The way your code was initially laid out, it would increment/decrement lapsDriven and barCount respectively by the passed parameter, then it would have gotten confused by the declaration of another method/declaration. This fix allows either to be called independently with regards to the method signature.
For future reference, on these coding challenges, a "task one is no longer passing" error usually is just a syntax error in whatever you're working on in the current task.

Danny Low
550 PointsThanks Charlie, that is really helpful and cheers for the tip on error codes will save me time in the future.