Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial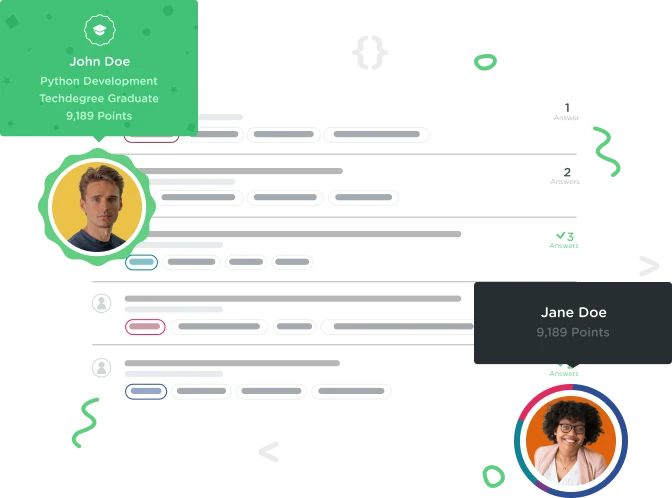
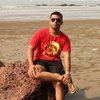
adriantennison
3,090 PointsMethod Signatures
How to call the drive method which is already declared and pass 1 to it as laps
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
int lap ;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void drive(){
drive(mBarsCount -= 1);
}
public void drive(int laps) {
// Other driving code omitted for clarity purposes
mBarsCount -= laps;
}
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_BARS;
}
}
2 Answers
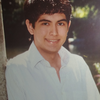
Jon Kussmann
Courses Plus Student 7,254 PointsThe idea is you will have the drive() method call the drive(int laps) method. In your drive() method, you do not need to do any calculation, just call the drive(int laps) method. The drive(int laps) method will do the subtraction for you - like so:
public void drive(){
drive(1);
}

Hayes McCardell II
643 PointsNot sure exactly what you're asking here. But you do have two drive methods with the same name, which isn't a great idea. If you want a method that takes an int laps, then perhaps name the method driveThisManyLaps.
So instantiate a new instance of GoKart, then access the method you need. Such as:
GoKart marioKart = new GoKart();
marioKart.driveThisManyLaps(1);
//or
marioKart.drive(); //
You could add some logic so that for every lap driven, one mbar would be decremented. This could all be done in one drive method.