Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial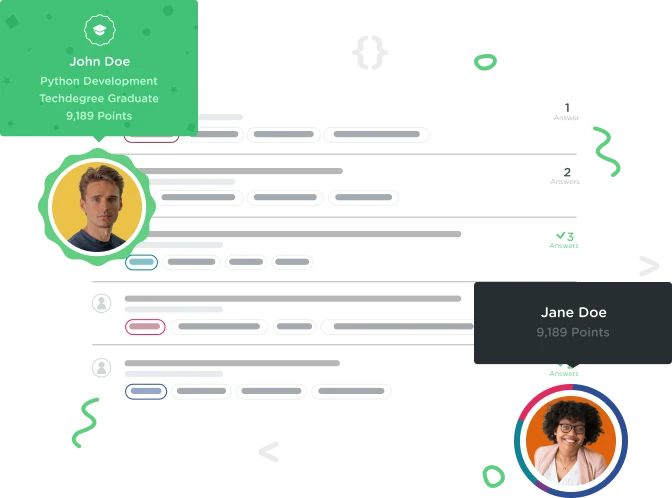
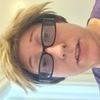
Katriel Paige
9,260 PointsMethod signatures, this challenge, and DRY - how to solve this default parameters challenge?
I got to the point where I fixed my typo (had the brackets nested originally) but I know I'm still duplicating the method. However, my other attempts bring up multiple syntax errors. What do I need to do to solve this challenge? It seems really tricky.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
} public void addItem(Product item){
System.out.printf("Adding 1 of %s to the cart.%n", item.getName());
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
1 Answer
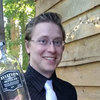
Evan Demaris
64,262 PointsHi Katriel,
This challenge mentions Please imagine lots and lots of code here. Don't repeat it.
in the method you're writing a new signature for. The intent of that comment is for you to keep the code DRY by calling the original method in the new signature; so addItem(item, 1);
instead of re-writing the code from the first method.
Hope that helps!
Katriel Paige
9,260 PointsKatriel Paige
9,260 PointsI tried addItem(item, 1); and got a screen full of syntax errors, which is why I'm really confused at this...
Evan Demaris
64,262 PointsEvan Demaris
64,262 PointsThe challenge will pass if you uncomment the code in Example.java, then update ShoppingCart.java to look as below; if you're still experiencing errors after that point, which specific errors are they?
Katriel Paige
9,260 PointsKatriel Paige
9,260 PointsThis is what I get:
Evan Demaris
64,262 PointsEvan Demaris
64,262 PointsThat error indicates that you've got to delete the second parameter in the second
addItem()
method; the method signature is identical in both methods, which makes the first function redundant.