Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial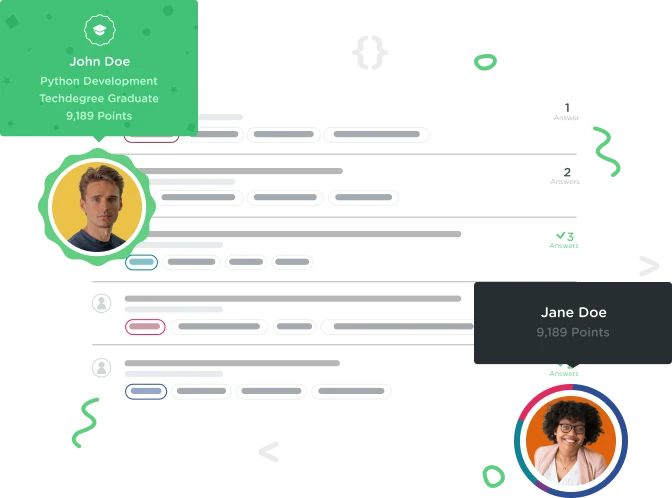

Dervin Buckery
Courses Plus Student 531 PointsMethods within methods
I was attempting the extra credit challenge. After looking around I found a line on code of stack overflow.
CODE: isInvalidWord (noun.toLowerCase().contains("Jerk".toLowerCase()));
The code works but I am kind of confused about how it works, So I am trying to break it down but I am not sure if I am correct.
My hypothesis:
noun.toLowerCase() - Since the variable noun contains input from the user it is basically taking that input and turning it to all lowercase. - User inputs ("JERK") the method will change it to ("jerk")
.contains( - This part is scanning for the continued word/characters
"Jerk".toLowerCase()) -Now its taking the contained word I entered (Jerk) and converting it to (jerk).
Is this the right logic?
1 Answer
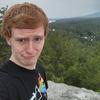
Brendon Butler
4,254 PointsThat answer is esentially correct. Just instead of nested methods, they're piggyback methods. (They have an actual name, but I can't remember it at this moment).
Basically any methods that return a value, can have another method concatenated on the end of it. You can create an infinite loop of these if you wanted, but there wouldn't really be any point to that.
If you wanted to make your own you can either have the method return a primitive data type or the instance of the class.
class Example {
public static void main(String[] args) {
RandomWords rw = new RandomWords();
String word = rw.changeWord().changeWord().getWord().toUpperCase().concat("-test");
System.out.println(word);
}
}
class RandomWords {
private String randomWord;
private String[] words;
RandomWords () {
words = new String[]{"Hello", "World", "Peter", "Monopoly"};
changeWord();
}
public Example changeWord() {
Random random = new Random();
randomWord = words[random.nextInt(3)];
return this;
}
public String getWord() {
return randomWord;
}
}
Sometimes this can be super handy! I'd definitely take a look into it.
Note: basically the string "jerk" has the String object's methods. When you use the toLowerCase
method, it returns a String, so you can append another method from the String class. Then keep doing it to your hearts content. But as soon as you return a different type of variable you would have to use methods from that new type of variable. The contains
method returns a Boolean, so you would no longer be able to append a method such as toUpperCase
. Hopefully, this makes sense. (I wrote it very quickly)