Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial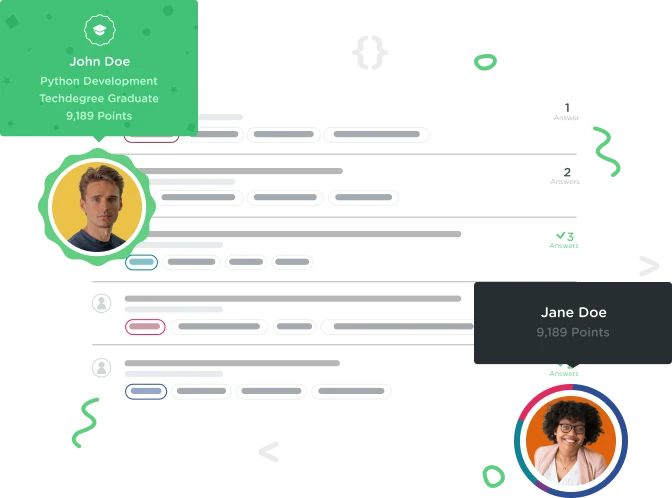

Ivan Monnier
Courses Plus Student 629 PointsMiddle interpretations
Just an observation wit little importance:
For the input string "Kenneth" your expected result is "kennETH"
If we consider the length of the word as 7 characters, the middle is 3.5, so if we round this number as an integer the result is 3, then I understand that result should be kenNETH and no kennETH.
This was my original code:
def sillycase(str_in): middle = int(len(str_in)/2) str_out = str_in[:middle].lower() + str_in[middle:].upper() return(str_out)
To get the challenge approved, had to do this:
def sillycase(str_in): middle = int(len(str_in)/2) if middle < len(str_in)/2: middle += 1 str_out = str_in[:middle].lower() + str_in[middle:].upper() return(str_out)
# The first half of the string, rounded with round(), should be lowercased.
# The second half should be uppercased.
# E.g. "Treehouse" should come back as "treeHOUSE"
def sillycase(str_in):
middle = int(len(str_in)/2)
if middle < len(str_in)/2:
middle += 1
str_out = str_in[:middle].lower() + str_in[middle:].upper()
return(str_out)
2 Answers
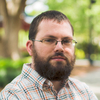
Kenneth Love
Treehouse Guest Teacherround(3.5)
will always produce 4.
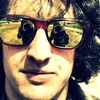
Adam Van Antwerp
3,104 PointsSOO this threw me for a bit of a loop at first. First of all, this is one of those answers that work differently in python2 relative to python3! In python2, len('kenneth')/2 will always evaluate to 3, since you are dividing an integer by another integer, so int(len('kenneth')/2) will never be less than len('kenneth')/2 (since they are always equal).
Moving on! Your problem lies in how you are trying to use the slice. Remember: str[0:3] should return you 'ken' NOT 'kenn'. The index is only included in the slice if it is in the start index, and will be excluded if it is in the end index. SO when you ask for str[0:3], you're asking for indices 0, 1 and 2, ending at 3 (not included). Your solution requires you to shift to the left one whenever there is an odd number. If the string has an even number of letters, this isn't a problem because there is no discrepancy as far as where the 'middle' of the string lies. For example, if the string was 'adam', len(str)/2 results in 2 and str[0:2] = 'ad' and str[2:] = 'am'. I hope this was helpful!
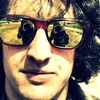
Adam Van Antwerp
3,104 PointsI guess what I'm trying to say and butchered: you obviously understand how to get this working in python3, and how to use the slices right. The only input I have is that this solution wouldn't work in python2, because that if statement would never be triggered. I guess what you were trying to say is that, with odd length words, the position of the middle is slightly arbitrary, right? In my opinion, your original solution without the if statement is just as correct as the actual solution (if not more), it's just that treehouse's definition of middle differs from yours when it comes to odd length words.
Ivan Monnier
Courses Plus Student 629 PointsIvan Monnier
Courses Plus Student 629 PointsAnd that's completely true! I just checked and it seems to be an error in my math bases, back to the basics :/ jeje. Sorry!
Apart of that, I was thinking that the function int() also rounded a float number but I just made some test and I saw my mistake.
Thanks for the replies Kenneth and Adam!