Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial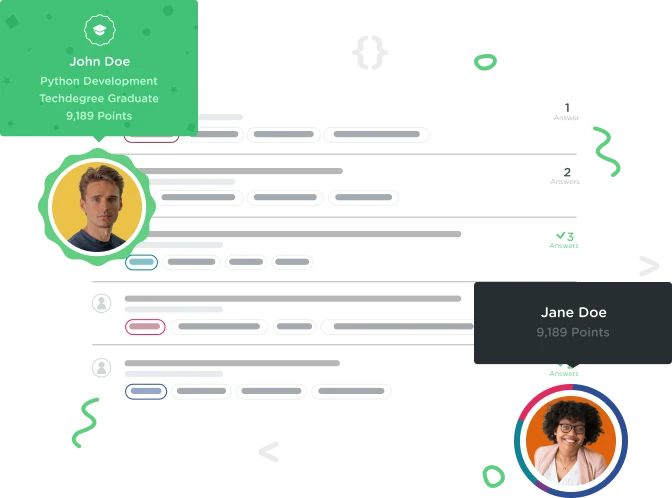
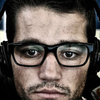
Ricardo Silva
12,144 PointsMiddleware routing (One ip server multiple domains redirection )
Hi guys,
I need some help.
How can i change this (Work!):
app.use(require('./_appOne/routes'));
to this (Not Work):
app.use((req, res) => {
//Get the hostname
let hostName = req.headers.host.split(':')[0];
//switch between projects
switch (hostName) {
case 'mydomainone.com':
require('./_appOne/routes')
break;
case 'mydomaintwo.com':
require('./_appTwo/routes')
break;
}
});
One ip server multiple domains redirection
Adicional Info: Project tree
server.js
_appOne routes index.js views index.pug
_appTwo routes index.js views index.pug
2 Answers
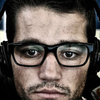
Ricardo Silva
12,144 PointsI found a solution to my problem.
NodeJs + Express Routing
var bouncy = require('bouncy');
var server = bouncy(function (req, res, bounce) {
if (req.headers.host === 'beep.example.com') {
bounce(8001);
}
else if (req.headers.host === 'boop.example.com') {
bounce(8002);
}
else {
res.statusCode = 404;
res.end('no such host');
}
});
server.listen(8000);
However, would like to know if this would be the best option (from a performance standpoint). Because I actually have to have three scripts to run instead of one
- Bounce main server
- First App
- Second App
will it be too heavy for the raspeberry pi?

Iain Simmons
Treehouse Moderator 32,305 PointsI don't know for sure it would work, but try just adding a return
before the require
statements in your initial code.
Alternatively, assign each of the separate routes to variables, and choose one to be your default and change to using a ternary statement so it will use
one or the other:
const appOneRoutes = require('./_appOne/routes');
const appTwoRoutes = require('./_appTwo/routes');
let routes;
app.use((req, res, next) => {
let hostName = req.headers.host.split(':')[0];
routes = hostName === 'mydomaintwo.com' ? appTwoRoutes : appOneRoutes;
next();
});
app.use(routes);
Gari Merrifield
9,597 PointsGari Merrifield
9,597 PointsFor starters, your let statement appears like it may return "http" or "https", at least from what I can find in a quick search. So, I suspect that what your switch statement is looking for will never happen.
Typically, under Apache or nGinx, you configure the web server itself to capture and direct the requested domain name. I think from your code, you could be working with Node.js as your web server, and I won't be able to help you there.