Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial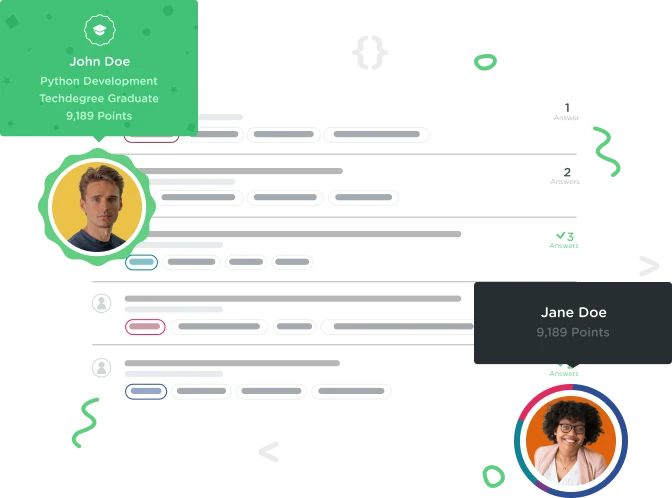

James Delaplain
5,872 PointsMight'eve asked already, but ITS STUCK 3 hours now trying to figure out how it is now stuck in the last two modules. :(
What could be Wrong? **********App.js*****
//Problem: No user interaction causes no change to application
//Solution: When user interacts cause changes appropriately
var color = $(".selected").css("background-color");
var $canvas = $("canvas");
var context = "$canvas[0].getContext("2d");
var lastEvent;
var mouseDown = false;
//When clicking on control list items
$(".controls").on("click", "li", function() {
//Deselect siblings elements
$(this).siblings().removeClass("selected");
//Select clicked element
$(this).addClass("selected");
//Cache current color
color = $(this).css("background-color");
});
//When "New Color" is pressed
$("#revealColorSelect").click(function(){
//Show color select or hide the color select
changeColor();
$("#colorSelect").toggle();
});
//Update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + ", " + g +", " + b + ")");
}
//When color sliders change
$("input[type=range]").on("input", changeColor);
//When add color is pressed
$("#addNewColor").click(function(){
//Append the color to the controls ul
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"));
$(".controls ul").append($newColor);
//Select the new color
$newColor.click();
});
//On mouse events on the canvas
$canvas.mousedown(function(e) {
lastEvent = e;
mouseDown == true;
}).mousemove(function(e) {
if(mouseDown) {
context.beginPath();
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
context.lineTo(e.offsetX, e.offsetY);
context.strokeStyle = color;
context.stroke();
lastEvent = e;
}
}).mouseup(function() {
mouseDown = false;
});.mouseLeave(function(){
$canvas.mouseup();
});
******************************style.CSS***********************************
body {
background: #384047;
font-family: sans-serif;
}
canvas {
background: #fff;
display: block;
margin: 50px auto 10px;
border-radius: 5px;
box-shadow: 0 4px 0 0 #222;
cursor: url(../img/cursor.png), crosshair;
}
.controls {
min-height: 60px;
margin: 0 auto;
width: 600px;
border-radius: 5px;
overflow: hidden;
}
ul {
list-style:none;
margin: 0;
float: left;
padding: 10px 0 20px;
width: 100%;
text-align: center;
}
ul li, #newColor {
display:block;
height: 54px;
width: 54px;
border-radius: 60px;
cursor: pointer;
border: 0;
box-shadow: 0 3px 0 0 #222;
}
ul li {
display:inline-block ;
margin: 0 5px 10px;
}
.red {
background: #fc4c4f;
}
.blue {
background: #4fa3fc;
}
.yellow {
background: #ECD13F;
}
.selected {
border: 7px solid #fff;
width: 40px;
height: 40px;
}
button {
background: #68B25B;
box-shadow: 0 3px 0 0 #6A845F;
color: #fff;
outline: none;
cursor: pointer;
text-shadow: 0 1px #6A845F;
display: block;
font-size: 16px;
line-height: 40px;
}
#revealColorSelect {
border: none;
border-radius: 5px;
margin: 10px auto;
padding: 5px 20px;
width: 160px;
}
/* New Color Palette */
#colorSelect {
background: #fff;
border-radius: 5px;
clear: both;
margin: 20px auto 0;
padding: 10px;
width: 305px;
position: relative;
display:none;
}
#colorSelect:after {
bottom: 100%;
left: 50%;
border: solid transparent;
content: " ";
height: 0;
width: 0;
position: absolute;
pointer-events: none;
border-color: rgba(255, 255, 255, 0);
border-bottom-color: #fff;
border-width: 10px;
margin-left: -10px;
}
#newColor {
width: 80px;
height: 80px;
border-radius: 3px;
box-shadow: none;
float: left;
border: none;
margin: 10px 20px 20px 10px;
}
.sliders p {
margin: 8px 0;
vertical-align: middle;
}
.sliders label {
display: inline-block;
margin: 0 10px 0 0;
width: 35px;
font-size: 14px;
color: #6D574E;
}
.sliders input {
position: relative;
top: 2px;
}
#colorSelect button {
border: none;
border-top: 1px solid #6A845F;
border-radius: 0 0 5px 5px;
clear: both;
margin: 10px -10px -7px;
padding: 5px 10px;
width: 325px;
}
************index.html**************************
<!DOCTYPE html>
<html>
<head>
<title>Simple Drawing Application</title>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<canvas width="600" height="400"></canvas>
<div class="controls">
<ul>
<li class="red selected"></li>
<li class="blue"></li>
<li class="yellow"></li>
</ul>
<button id="revealColorSelect">New Color</button>
<div id="colorSelect">
<span id="newColor"></span>
<div class="sliders">
<p>
<label for="red">Red</label>
<input id="red" name="red" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="green">Green</label>
<input id="green" name="green" type="range" min=0 max=255 value=0>
</p>
<p>
<label for="blue">Blue</label>
<input id="blue" name="blue" type="range" min=0 max=255 value=0>
</p>
</div>
<div>
<button id="addNewColor">Add Color</button>
</div>
</div>
</div>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
2 Answers
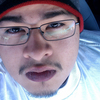
Chyno Deluxe
16,936 PointsThere are two things I see wrong with the code in regards to syntax errors. I don't know where you are suppose to be in relation to the course but here are the issues that you'll need to fix in order to get rid of he syntax errors.
- You have an unnecessary (") on your context variable.
- You have a semi-colon just before your .mouseleave
//Problem: No user interaction causes no change to application
//Solution: When user interacts cause changes appropriately
var color = $(".selected").css("background-color");
var $canvas = $("canvas");
var context = $canvas[0].getContext("2d");
var lastEvent;
var mouseDown = false;
//When clicking on control list items
$(".controls").on("click", "li", function() {
//Deselect siblings elements
$(this).siblings().removeClass("selected");
//Select clicked element
$(this).addClass("selected");
//Cache current color
color = $(this).css("background-color");
});
//When "New Color" is pressed
$("#revealColorSelect").click(function(){
//Show color select or hide the color select
changeColor();
$("#colorSelect").toggle();
});
//Update the new color span
function changeColor() {
var r = $("#red").val();
var g = $("#green").val();
var b = $("#blue").val();
$("#newColor").css("background-color", "rgb(" + r + ", " + g +", " + b + ")");
}
//When color sliders change
$("input[type=range]").on("input", changeColor);
//When add color is pressed
$("#addNewColor").click(function(){
//Append the color to the controls ul
var $newColor = $("<li></li>");
$newColor.css("background-color", $("#newColor").css("background-color"));
$(".controls ul").append($newColor);
//Select the new color
$newColor.click();
});
//On mouse events on the canvas
$canvas.mousedown(function(e) {
lastEvent = e;
mouseDown == true;
}).mousemove(function(e) {
if(mouseDown) {
context.beginPath();
context.moveTo(lastEvent.offsetX, lastEvent.offsetY);
context.lineTo(e.offsetX, e.offsetY);
context.strokeStyle = color;
context.stroke();
lastEvent = e;
}
}).mouseup(function() {
mouseDown = false;
}).mouseLeave(function(){
$canvas.mouseup();
});
I hope this helps put you on the right path.

James Delaplain
5,872 Pointsthank you for the Help !!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!!! Darn funny little things like that are blind to us sometimes. THANk you for LOOKINg hope you didn't get time away from a good amber beer and conversation. Golly I need that!
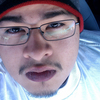
Chyno Deluxe
16,936 Points=) Not a problem. Glad I could help.
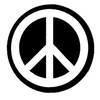
john larson
16,594 PointsI just realized a lot of people make a big thing about not forgetting to put in semicolons, but more problems happen cause there in when they're not supposed to be.
Chyno Deluxe
16,936 PointsChyno Deluxe
16,936 Points//Fixed Code Presentation How to post Code