Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial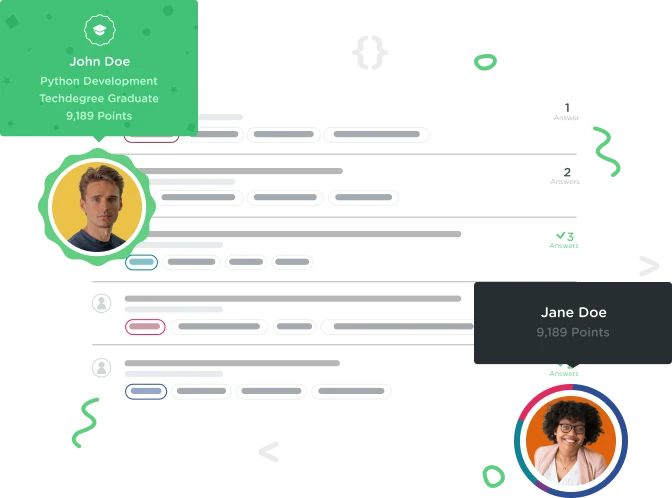

kevinkrato
5,452 PointsMind being too blown
Hello, so I've been working through this challenge and I tried writing it out myself and went down this overly-complex 'rabbit-hole' of functions, for-statements, conditional statements, etc. After digging myself too far into that hole, I went back and watched what the teacher did. It's pretty simple so I figured I would re-create his work without looking at his video. I came across something, a programming concept, that I'm struggling to wrap my mind around. It's nothing NASA level, but I would like to have it explained out to me.
Data Array Source:
var studentData = [
{ name:'Kevin', track:'JavaScript', achievements:18, points:2230 },
{ name:'ScaryTerry', track:'Java', achievements:22, points:2731 },
{ name:'Rick', track:'HTML', achievements:131, points:17311 },
{ name:'Morty', track:'HTML', achievements:13, points:1341 },
{ name:'Jerry', track:'CSS', achievements:0, points:13 }
];
CORRECT
for(var i = 0; i < studentData.length; i += 1) {
student = studentData[i];
message += '<h2> Student: ' + student.name + '</h2>';
message += '<p> Track: ' + student.track + '</p>';
message += '<p> Achievements: ' + student.achievements + '</p>';
message += '<p> Score: ' + student.points + '</p>';
}
INCORRECT
for(var i = 0; i < studentData.length; i += 1) {
student = studentData[i];
message = '<h2> Student: ' + student.name + '</h2>';
message += '<p> Track: ' + student.track + '</p>';
message += '<p> Achievements: ' + student.achievements + '</p>';
message += '<p> Score: ' + student.points + '</p>';
}
So, the difference between the 'CORRECT' and 'INCORRECT' blocks is that the first 'message' that starts off the HTML text is a '+=' vs. '='.
Could someone explain to me how the program is thinking through the 'INCORRECT' version and why it only results in displaying the last object's data (Jerry) in the studentData variable and not instead cycling through all of the array, posting the HTML messages, then repeating the for loop until completed?
TLDR; why does the '+=' make such a huge difference compared to '=' in this code?
Thanks! (i'll best answer whoever gives the... well... best answer.)
3 Answers
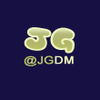
Jonathan Grieve
Treehouse Moderator 91,253 PointsWell the difference between =
and +=
is that the first is purely an assignment operator.
You're either giving a new variable a value or resetting the value of an existin variable to the new value. i.e.
var name;
name = "Kevin";
console.log(name);
name = "Jonathan";
console.log(name)
+= is a little different.
What that's saying is take the value of the variable to the left of the operator and add the value to the right to the end of it.
So it would look something like this.
var name;
name = "Kevin";
name += " Krato";
//outputs Kevin Krato
console.log(name)
My guess is the message
variable is previously declared in the challenge and you just need the += to make the required additions to the string!
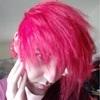
Patrik Horváth
11,110 Points- INCORRECT - you have to echo/print inside For Loop, because you always overwrite your variable ( you get always only LAST student if you echo/print outside for loop)
- CORRECT - you have to echo/print outside For Loop

andren
28,558 PointsCould someone explain to me how the program is thinking through the 'INCORRECT' version and why it only results in displaying the last object's data (Jerry) in the studentData variable and not instead cycling through all of the array, posting the HTML messages, then repeating the for loop until completed?
The program is cycling though all of the items in the array in both examples, the issue is that when you set a variable equal to a value you completely replace the value that was in the variable previously. Meaning that at the start of each loop the message
variable is set to '<h2> Student: ' + student.name + '</h2>';
and anything that was in it previously disappears.
The += operator on the other hand simply adds the value to the variable, it never replaces the content, which means that the content of the message
variable is never reset like it is in the "incorrect" code example.
Here is some code that illustrates this difference that might be easier to wrap your mind around:
example = "Hello"
// example at this point holds "Hello"
example = "World"
// example at this point holds "World"
example2 = "Hello "
// example2 at this point holds "Hello "
example2 += "World"
// example2 at this point holds "Hello World"
With the example2
variable you can see that the second string is appended to the variable, rather than having it's value replaced outright like with the example
variable. The exact same thing happens with the message
variable within the loop in your examples. In the "correct" code example it keeps its values though all of the loops, in the "incorrect" code example it gets is values replaced at the start of each loop iteration.
Patrik Horváth
11,110 PointsPatrik Horváth
11,110 Points