Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial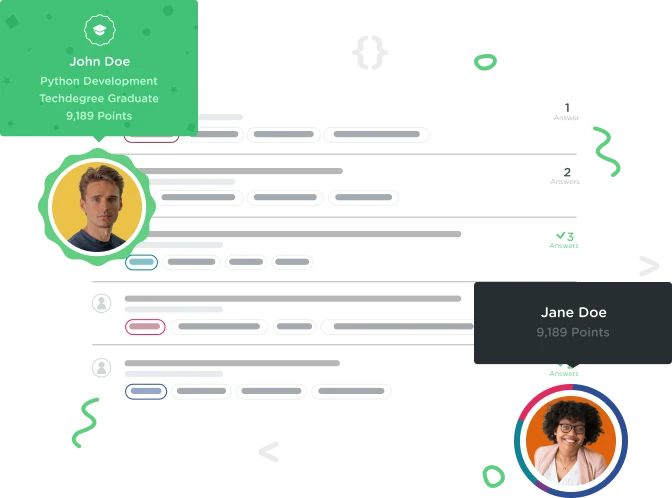
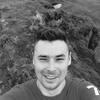
jamesjones21
9,260 Pointsmind is blown about 'this' keyword in Javascript
Hi,
I have the following object which calcAge property is set to a method (so it will be similar to a function expression.
The code below where I console.log(this) it will refer to the current object.
const john = {
firstName: 'John',
lastName: 'Smith',
birthYear: 1990,
family: ['jane', 'bob', 'emily'],
job: 'teacher',
isMarried: false,
calcAge: function(){
console.log(this);
//return 2018 - this.birthYear;
},
};
But when I change it to the ES6 arrow function it is then pointing to the global scope which is the window:
const john = {
firstName: 'John',
lastName: 'Smith',
birthYear: 1990,
family: ['jane', 'bob', 'emily'],
job: 'teacher',
isMarried: false,
calcAge: () => {
console.log(this);
//return 2018 - this.birthYear;
},
};
Can someone please attempt to explain this?
Kind regards,
James
2 Answers
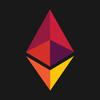
Richard Verbraak
7,739 PointsAs someone who just struggled to learn about the this keyword. I found this helpful topic about ES6 Arrow functions and the this keyword.
If I'm reading correctly, arrow functions use lexical scoping. The this keyword doesn't bind to it's parent so to speak (the object) but to it's function. So it get's the window object instead.
Feel free to correct me here.
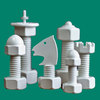
Steven Parker
231,184 PointsArrow functions are not always suitable replacements for conventional functions. One way they are different is that they do not establish a value for "this" like conventional functions do. For this reason (and perhaps others), MDN suggests that "Arrow function expressions are ill suited as methods".
For more details on the differences, see the MDN page on Arrow Functions.
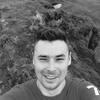
jamesjones21
9,260 PointsSo they are more suitable as function expression? Which is why they were created.
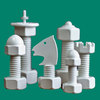
Steven Parker
231,184 PointsWell, they are function expressions. What MDN is suggesting is that they might not be the best choice for class method implementation.
jamesjones21
9,260 Pointsjamesjones21
9,260 PointsJust read that now but found a YouTube video on it which explains it all. If arrow function is used in the object then it binded to the window which means it was binded to the window when the function was created. How mad is this language, 🤣
Here is the link: https://youtu.be/eOI9GzMfd24
6 mins onwards you'll see he puts an example which I kinda get k It. Just have to know when and where to use them
Richard Verbraak
7,739 PointsRichard Verbraak
7,739 PointsAh thanks for the video. I literally just started learning about that keyword today and was so confused the first 5 hours haha. Then I see your post and it made me scratch my head again hahah. But atleast we both got it now!
jamesjones21
9,260 Pointsjamesjones21
9,260 Pointsthis is my current practice to understand, so this will be a good session to get my head around it. Which I am hoping to use this within my current small project and change some of syntax to refer to this.
https://github.com/bladedevleoper/wage-planner
jamesjones21
9,260 Pointsjamesjones21
9,260 Pointshere is some of my understanding from off top of my head: