Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial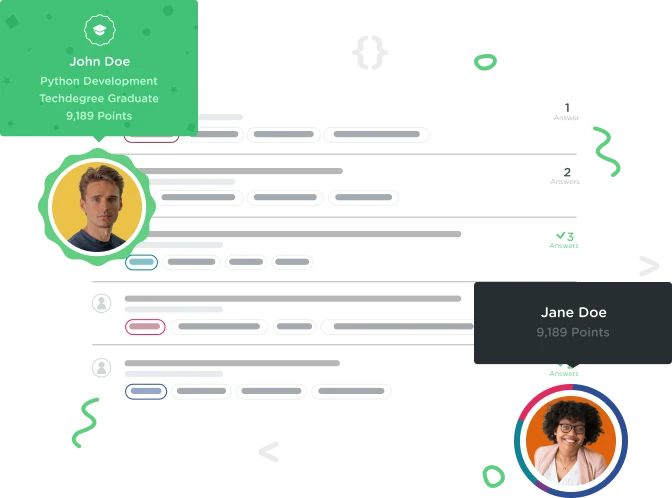
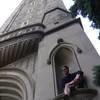
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMinesweeper
I have been working on a minesweeper clone with JavaScript for the past few days. It's not totally done, still a few UI aspects to add but the game is playable. I wanted to post it for anyone curious to view the code, play the game and maybe critique the code. I am linking the github repo and there is a link to a github pages demo. I would appreciate anyone who wants to critique any aspects of the code!
1 Answer
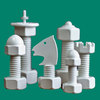
Steven Parker
231,248 PointsBeautiful job so far! You're only a few minor tweaks away from a fully implemented clone of the original!
At first glance, the code looks very clean. I spotted one opportunity for making it a bit more compact:
drawBorderingMinesCount(space)
{
const mineCount = space.borderingMinesCount;
if (mineCount > 0) {
const el = document.getElementById(space.id);
const colors = ['#0E00FB', '#037E00', '#FD0A00', '#040280',
'#810202', '#00807F', 'black', '#808080'];
el.style.color = colors[mineCount - 1];
el.textContent = `${mineCount}`;
}
}
Be sure to post an update when you finish. Great work!
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsThanks very much Steven. I did get some inspiration on the CSS from someone else's minesweeper clone though so I can't take too much credit. I am concerned though that some of my code might not be very efficient in some places. In expert mode the game can be slightly slow. I am hoping that a future version can be a little faster.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsAdmittedly that is a much more elegant solution than I had. I think I just learned a nice little technique. Thanks!!
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsHere is a "complete" demo: https://michaelacook.github.io/minesweeper/ Steven, is this a project I should bother showing employers? Soon I'll be starting to apply for junior developer jobs. I've heard the opinion that projects like this aren't worth showing; yet I feel like the amount of work I put into it merits at least giving it a spot in my portfolio. But I would value your input on this.
Steven Parker
231,248 PointsSteven Parker
231,248 PointsI'm not sure what "projects like this" means, or where you got that advice, but this is a great demo I and think it would be an excellent addition to your portfolio.
Some minor functional observations — as I recall, the goal in the original was to clear all non-mine squares, but this one only seems to register a "win" from flagging the mines themselves (which is optional in the original). It also seems to have a bug where in some cases, even flagging all the mines doesn't stop the timer.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsI noticed those same bugs and corrected them. The demo should reflect the most recent changes. In my travels on YouTube I encountered a developer who appears to make his living selling over-priced tutorials advising that only projects that an employer might actually pay you to build should be used in a portfolio, and games and other pet projects should be left out because they aren't "serious" projects. My impression was that that is only true to a point, but I wanted to know what you thought.
Steven Parker
231,248 PointsSteven Parker
231,248 PointsI was able to flag all the mines and clear all the other squares with the clock still running just now.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsThat is very strange. Which browser are you using? And would you be so kind as to clear your browser cache and then try again and let me know the result? That was happening before on my end but not since I fixed it.
Steven Parker
231,248 PointsSteven Parker
231,248 PointsI use Chrome, and still no difference. Timer keeps running when all non-mines are cleared and/or all mines are flagged. Note the the latter seems to occur only is you first set flags on non-mines and then remove and replace them correctly later.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsAh, I think I misread you before. I found the bug you were referring to and fixed it. When unflagging a non-mine containing space, the
handleRightClicks
method was still subtracting one from the game object'sflaggedMines
property. Should work fine now. Thanks very much for doing all this testing for me!Steven Parker
231,248 PointsSteven Parker
231,248 PointsPost back if you update the code to allow a "win" by removing all non-mines (with or without marking any).
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsI have pushed a new commit allowing you to win without flagging any spaces. Simply isolating all the mines is enough to win the game. I would have done it sooner but I work a full-time day job and study like crazy at night. If you feel like it let me know if you notice any bugs, though I think it should work fine. Thanks again for your help. Soon I'll update my readme and mention you under the ackknowledgements.
Steven Parker
231,248 PointsSteven Parker
231,248 PointsGood job!
Steven Parker
231,248 PointsSteven Parker
231,248 PointsMichael Cook — I played it one more time today and I eliminated all but 10 squares on the beginner level and the clock still ran. Then I placed just one flag and it scored a "win".
It took several more (totally fine) games to re-create the bug but I got the same behavior once more.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsThanks for letting me know. These bugs are really stubborn. You think you have everything working right, the code seems to check out and bam. Bug. But I guess that's just software development. Hopefully not incompetence.
Michael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsMichael Cook
Full Stack JavaScript Techdegree Graduate 28,975 PointsI had a look and it seems the
handleClick
method was only checking for a win after a left click on a single space, then opening the board. Thus the game could be complete because the board opens in such a way that the remaining mines are isolated, yet the game would not check for a win. I made a simple alteration that should fix this. If you happen to notice yet another bug do let me know and again, thanks for playing the game and pointing out these bugs!!